How to Slice 2D Array in NumPy
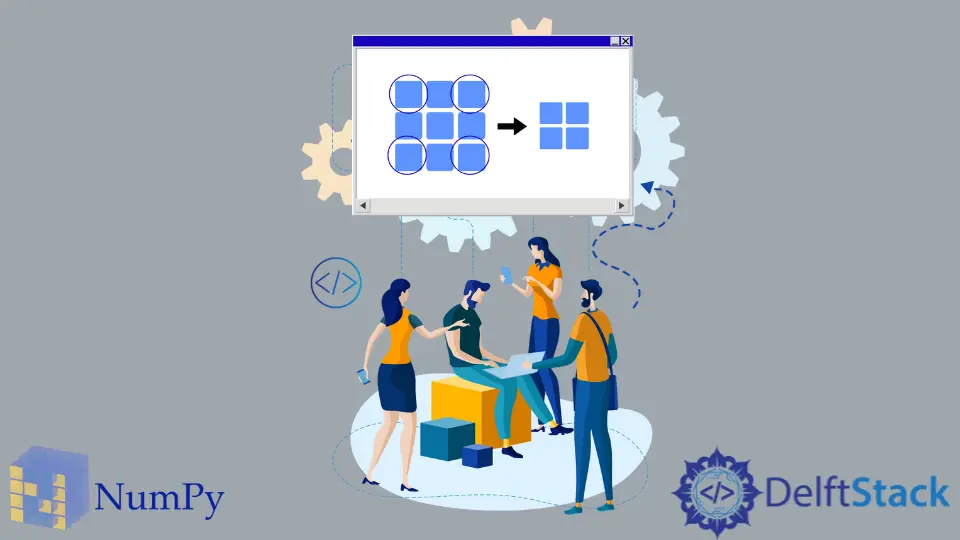
This tutorial will introduce how to slice a 2D array in NumPy.
Slice 2D Array With Array Indexing in NumPy
If we have a main 2D NumPy array and we want to extract another 2D sub-array from it, we can use the array indexing method for this purpose. Let us take an array of 4*4
shape for this example. It is pretty simple to extract the first and last elements of the array. For example, array[0:2,0:2]
will give us a view or sub-array that contains the first two elements inside the array both vertically and horizontally. Similarly, array[2:,2:]
will give us a view or sub-array that contains the last two elements inside the array both vertically and horizontally. The more complex job is getting the elements from different rows and columns by skipping a middle row or column. The following code example shows us exactly how to achieve this with array indexing in Python.
import numpy as np
x = range(16)
x = np.reshape(x, (4, 4))
print(x)
y = x[[[0], [2]], [1, 3]]
print(y)
Output:
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]
[12 13 14 15]]
[[ 1 3]
[ 9 11]]
In the above code, we extracted the elements in rows 1 and 3 that intersect with columns 1 and 3 while skipping row 2 and column 2 with the array indexing method in Python. This can also be done with a similar approach but with some different syntax, as shown in the coding example below.
import numpy as np
x = range(16)
x = np.reshape(x, (4, 4))
print(x)
y = x[0::2, 1::2]
print(y)
Output:
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]
[12 13 14 15]]
[[ 1 3]
[ 9 11]]
In the above code, we also extracted the elements in row 1 and 3 that intersect with the column 1 and 3 while skipping row 2 and column 2 with the array indexing method in Python. This method is easier than the previous approach because it does not involve too many brackets and is overall more readable.
Slice 2D Array With the numpy.ix_()
Function in NumPy
The numpy.ix_()
function forms an open mesh form sequence of elements in Python. This function takes n
1D arrays and returns an nD array. We can use this function to extract individual 1D slices from our main array and then combine them to form a 2D array. The following code example does the same job as the previous examples but by using the numpy.ix_()
function with array indexing in Python.
import numpy as np
x = range(16)
x = np.reshape(x, (4, 4))
print(x)
y = x[np.ix_([0, 2], [1, 3])]
print(y)
Output:
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]
[12 13 14 15]]
[[ 1 3]
[ 9 11]]
In the above code, we basically did the exact same thing as the previous examples but by using the np.ix_()
function along with array indexing in Python.
The main idea behind all these examples is the same. When we created our main array, a buffer was allocated to it depending upon its shape and size. The array indexing method creates a new object of array data type that points at the memory buffer of our main array. In all of the above examples, even though y
is a new array, but it does not take any buffer in memory. It only points at certain locations on the memory buffer of the array x
. This is what makes the array indexing method better than just creating a new array.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn