How to NumPy Shuffle Two Arrays
-
NumPy Shuffle Two Arrays With the
sklearn.utils.shuffle()
Function in Python -
NumPy Shuffle Two Arrays With the
numpy.random.shuffle()
Function -
NumPy Shuffle Two Corresponding Arrays With the
numpy.random.permutation()
Function in Python
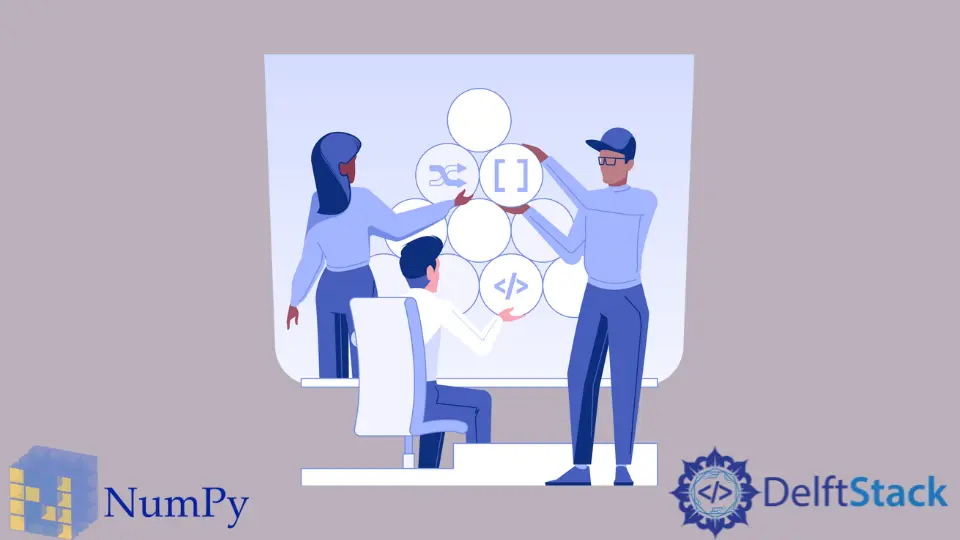
This tutorial will introduce how to shuffle two NumPy arrays in Python.
NumPy Shuffle Two Arrays With the sklearn.utils.shuffle()
Function in Python
Suppose we have two arrays of the same length or same leading dimensions, and we want to shuffle them both in a way that the corresponding elements in both arrays remain corresponding. In that case, we can use the shuffle()
function inside the sklean.utils
library in Python. This shuffle()
function takes the arrays as input parameters, shuffles them consistently, and returns a shuffled copy of each array. See the following code example.
import numpy as np
from sklearn import utils
array1 = np.array([[0, 0], [1, 1], [2, 2]])
array2 = np.array([0, 1, 2])
array1, array2 = utils.shuffle(array1, array2)
print(array1)
print(array2)
Output:
[[0 0]
[2 2]
[1 1]]
[0 2 1]
In the above code, we shuffled the two arrays, array1
and array2
, with the shuffle()
function inside the sklearn.utils
library in Python. We first created both arrays with the np.array()
function. We then shuffled the arrays with the shuffle()
function inside the sklearn.utils
library and saved the shuffled arrays inside array1
and array2
. In the end, we printed the elements inside both arrays. The output shows that the elements of both arrays correspond even after shuffling.
NumPy Shuffle Two Arrays With the numpy.random.shuffle()
Function
If we don’t want to import the sklearn
package and want to achieve the same goal as the previous one by using the NumPy package, we can use the shuffle()
function inside the numpy.random
library. This shuffle()
function takes a sequence and randomizes it. We can then use this randomized sequence as an index for the two arrays to shuffle them. The following code example shows us how we can shuffle two arrays with the numpy.random.shuffle()
function.
import numpy as np
array1 = np.array([[0, 0], [1, 1], [2, 2]])
array2 = np.array([0, 1, 2])
randomize = np.arange(len(array2))
np.random.shuffle(randomize)
array1 = array1[randomize]
array2 = array2[randomize]
print(array1)
print(array2)
Output:
[[2 2]
[0 0]
[1 1]]
[2 0 1]
We first created the arrays with the np.array()
function. We then created a sequence of integers that is equal to the length of the second array with the np.arange(len(array2))
function. After that, we randomized the sequence with the shuffle()
function inside the np.random
library and used it as an index for both arrays to shuffle them.
NumPy Shuffle Two Corresponding Arrays With the numpy.random.permutation()
Function in Python
We can also use the permutation()
function inside the numpy.random
library to create a randomized sequence of integers within a specified range in Python. This sequence can then be used as an index for both arrays to shuffle them.
import numpy as np
def shuffle(x, y):
p = np.random.permutation(len(y))
return x[p], y[p]
array1 = np.array([[0, 0], [1, 1], [2, 2]])
array2 = np.array([0, 1, 2])
array1, array2 = shuffle(array1, array2)
print(array1)
print(array2)
Output:
[[0 0]
[2 2]
[1 1]]
[0 2 1]
In the above code, we defined a function shuffle()
that takes two arrays and randomizes them with the permutation()
function inside the numpy.random
library in Python. We specified the length of the randomized sequence to be equal to the number of elements in the second array with the len(y)
function. We then used the randomized sequence p
as an index for both arrays and returned them. The shuffled arrays returned by the shuffle()
function are stored inside the array1
and array2
arrays.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn