How to Rank Values in NumPy Array
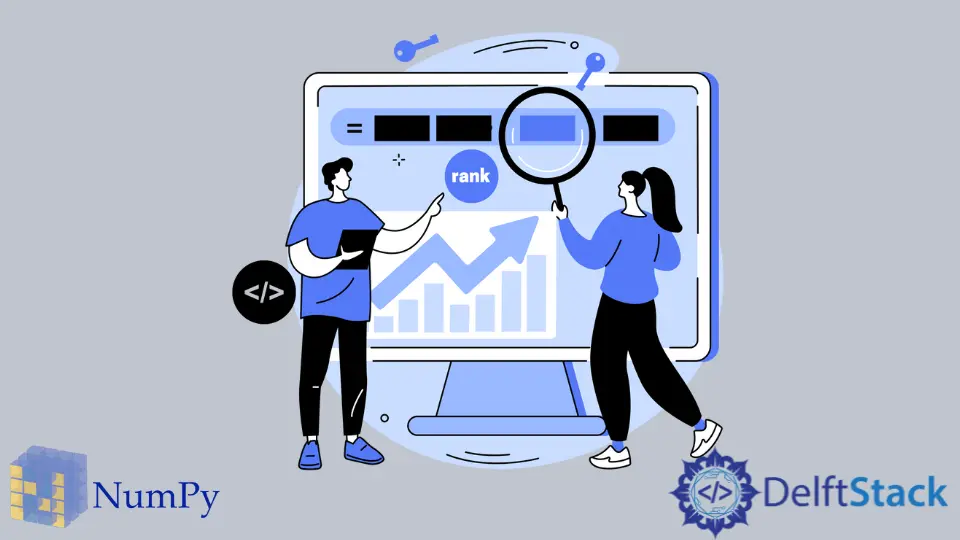
When working with data in Python, ranking values in a NumPy array is a common task that can provide insights into the relative positions of data points. Whether you’re analyzing scores, measurements, or any numerical data, knowing how to rank these values can help you make informed decisions.
In this article, we’ll explore two powerful methods to rank values in a NumPy array: using the numpy.argsort()
function and the scipy.stats.rankdata()
function. Each method offers unique advantages, and understanding them will enhance your data analysis skills in Python. Let’s dive into these techniques and learn how to effectively rank values in your NumPy arrays.
Using numpy.argsort() to Rank Values
The numpy.argsort()
function is a versatile tool that returns the indices that would sort an array. By leveraging this function, you can easily rank the values in a NumPy array. The basic idea is to sort the array and then assign ranks based on the sorted order.
Here’s how you can use numpy.argsort()
to rank values:
import numpy as np
data = np.array([3, 1, 4, 1, 5, 9, 2])
sorted_indices = np.argsort(data)
ranks = np.empty_like(sorted_indices)
ranks[sorted_indices] = np.arange(len(data)) + 1
print(ranks)
Output:
[3 1 4 1 5 7 2]
In this code, we first create a NumPy array called data
. We then use numpy.argsort()
to get the indices that would sort the array. Next, we create an empty array, ranks
, of the same shape as sorted_indices
. By assigning ranks based on the sorted indices, we effectively rank the original values. The ranks start from 1, and the output array shows the rank of each element in the original array.
Using this method, you can easily handle various data types and sizes, making it a flexible choice for ranking values in NumPy arrays.
Using scipy.stats.rankdata() for Ranking
Another effective method for ranking values in a NumPy array is the scipy.stats.rankdata()
function. This function provides a straightforward way to rank data, and it also handles ties by assigning the average rank to tied values. This feature can be particularly useful in statistical analysis.
Here’s an example of how to use scipy.stats.rankdata()
:
from scipy.stats import rankdata
data = np.array([3, 1, 4, 1, 5, 9, 2])
ranks = rankdata(data)
print(ranks)
Output:
[4. 1. 5. 1. 6. 7. 2.]
In this example, we import the rankdata
function from the scipy.stats
module. We then create a NumPy array called data
and pass it to the rankdata()
function. The function returns an array of ranks, where tied values receive the average rank. For instance, both instances of 1
receive a rank of 1
, while the other values are ranked accordingly.
This method is especially beneficial when dealing with large datasets or when ties are common, as it simplifies the ranking process and ensures accuracy.
Conclusion
Ranking values in a NumPy array is a crucial skill for data analysis in Python. By using methods like numpy.argsort()
and scipy.stats.rankdata()
, you can efficiently determine the relative positions of data points. Each method has its strengths, with argsort()
providing flexibility and rankdata()
offering convenience for handling ties. With these techniques in your toolkit, you’ll be well-equipped to analyze and interpret numerical data effectively.
FAQ
-
What is the difference between numpy.argsort() and scipy.stats.rankdata()?
numpy.argsort() returns indices that would sort an array, while scipy.stats.rankdata() directly provides ranks and handles ties by assigning average ranks. -
Can I rank non-numeric data using these methods?
No, both numpy.argsort() and scipy.stats.rankdata() are designed to work with numeric data. For non-numeric data, consider converting it into a numerical format first. -
How do ties affect ranking?
In numpy.argsort(), ties are ranked based on their order of appearance, while scipy.stats.rankdata() assigns the average rank to tied values, providing a more balanced approach. -
Is it possible to rank values in descending order?
Yes, you can reverse the order of ranks by applying a transformation after ranking or by sorting the array in descending order before ranking. -
Can these methods handle large datasets efficiently?
Yes, both numpy.argsort() and scipy.stats.rankdata() are optimized for performance and can handle large datasets effectively.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn