numpy.random.permutation() Function in NumPy
- What is numpy.random.permutation()?
- Using numpy.random.permutation() with an Array
- Using numpy.random.permutation() with an Integer
- Differences Between numpy.random.permutation() and numpy.random.shuffle()
- Conclusion
- FAQ
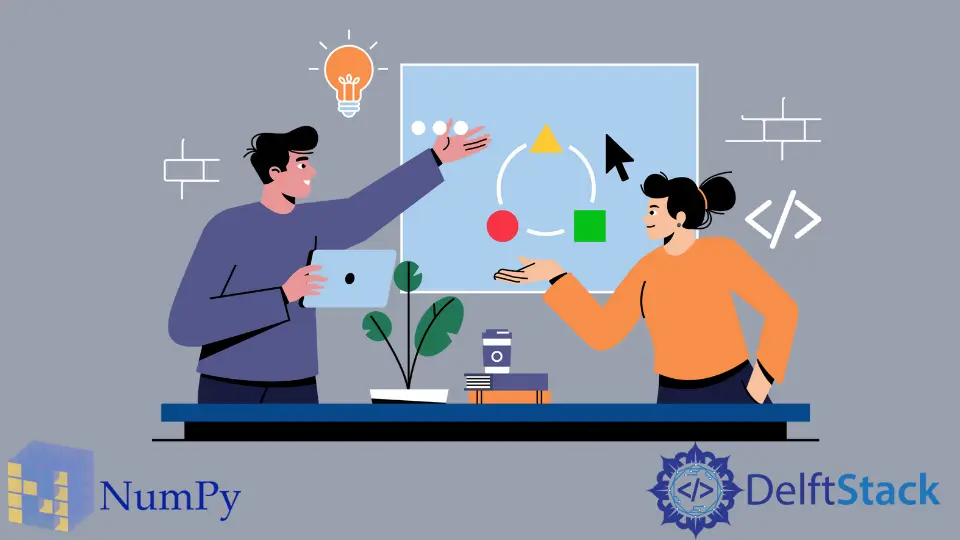
When it comes to data manipulation and analysis in Python, NumPy stands out as a powerful library. One of its most useful features is the numpy.random.permutation()
function. This function is essential for anyone looking to shuffle data effectively, especially in the realm of machine learning and statistical analysis. The main distinction between numpy.random.permutation()
and numpy.random.shuffle()
is crucial: while permutation()
returns a shuffled copy of the array, shuffle()
modifies the original array directly.
This article will delve into how to use numpy.random.permutation()
effectively, providing clear examples and explanations to enhance your understanding.
What is numpy.random.permutation()?
The numpy.random.permutation()
function is designed to randomly permute a sequence or return a permuted range. It can take either an array or an integer as input. When an integer is passed, it generates a random permutation of integers from 0 to n-1. If an array is provided, it produces a shuffled copy of that array without altering the original data. This feature makes permutation()
particularly useful in scenarios where you need to maintain the integrity of your data while still requiring a randomized version for testing or validation purposes.
Using numpy.random.permutation() with an Array
To illustrate how numpy.random.permutation()
works with an array, let’s consider a simple example. We will create an array of integers and use the function to generate a shuffled copy.
import numpy as np
original_array = np.array([1, 2, 3, 4, 5])
shuffled_array = np.random.permutation(original_array)
print("Original Array:", original_array)
print("Shuffled Array:", shuffled_array)
Output:
Original Array: [1 2 3 4 5]
Shuffled Array: [3 1 5 2 4]
In this example, we first import the NumPy library and create an array called original_array
containing integers from 1 to 5. We then call np.random.permutation(original_array)
to create a shuffled version of this array, which we store in shuffled_array
. The original array remains unchanged, demonstrating the primary advantage of using permutation()
. This feature is particularly beneficial in scenarios where retaining the original dataset is essential, such as during cross-validation in machine learning.
Using numpy.random.permutation() with an Integer
The numpy.random.permutation()
function can also accept an integer as an argument. In this case, it generates a random permutation of integers ranging from 0 to n-1. This can be helpful for generating random indices or for use in simulations.
import numpy as np
n = 5
permuted_indices = np.random.permutation(n)
print("Permuted Indices:", permuted_indices)
Output:
Permuted Indices: [4 0 2 3 1]
In this code snippet, we set n
to 5 and call np.random.permutation(n)
. The function returns a shuffled array of indices from 0 to 4. This feature is particularly useful in scenarios such as shuffling data labels or creating random samples from a dataset. By obtaining a random order of indices, you can easily access elements of an array or perform operations like bootstrapping or cross-validation in machine learning tasks.
Differences Between numpy.random.permutation() and numpy.random.shuffle()
Understanding the differences between numpy.random.permutation()
and numpy.random.shuffle()
is crucial for effective data manipulation. While both functions are used for shuffling, their behavior differs significantly.
numpy.random.shuffle()
The numpy.random.shuffle()
function modifies the original array in place. This means that the original data structure is altered, and no new array is created. This can be useful when you want to shuffle data and do not need to retain the original order.
import numpy as np
original_array = np.array([1, 2, 3, 4, 5])
np.random.shuffle(original_array)
print("Shuffled Original Array:", original_array)
Output:
Shuffled Original Array: [2 5 1 4 3]
In this example, we see that np.random.shuffle(original_array)
directly shuffles the contents of original_array
, and the original order is lost. This can be useful in scenarios where you want to quickly randomize data without needing to create a separate copy. However, it’s essential to remember that this function alters the original dataset, which may not be desirable in all situations.
Conclusion
The numpy.random.permutation()
function is a valuable tool in the NumPy library for anyone working with data in Python. Its ability to shuffle data while preserving the original array makes it an essential function for tasks such as data analysis and machine learning. By understanding how to use permutation()
effectively, you can enhance your data manipulation skills and ensure that your analyses are both robust and reliable. Whether you’re generating random indices or creating shuffled copies of your datasets, numpy.random.permutation()
is a function worth mastering.
FAQ
- what is numpy.random.permutation() used for?
numpy.random.permutation() is used to create a shuffled copy of an array or to generate a random permutation of integers.
-
how does numpy.random.permutation() differ from numpy.random.shuffle()?
numpy.random.permutation() returns a shuffled copy of the array, while numpy.random.shuffle() modifies the original array in place. -
can numpy.random.permutation() accept an integer as input?
Yes, when an integer is passed to numpy.random.permutation(), it generates a random permutation of integers from 0 to n-1. -
is it safe to use numpy.random.permutation() on large datasets?
Yes, numpy.random.permutation() is efficient and can handle large datasets, but be mindful of memory usage when creating copies. -
can I use numpy.random.permutation() for machine learning tasks?
Absolutely! It is commonly used in machine learning for shuffling data, creating random samples, and performing cross-validation.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn