How to Map a Function in NumPy
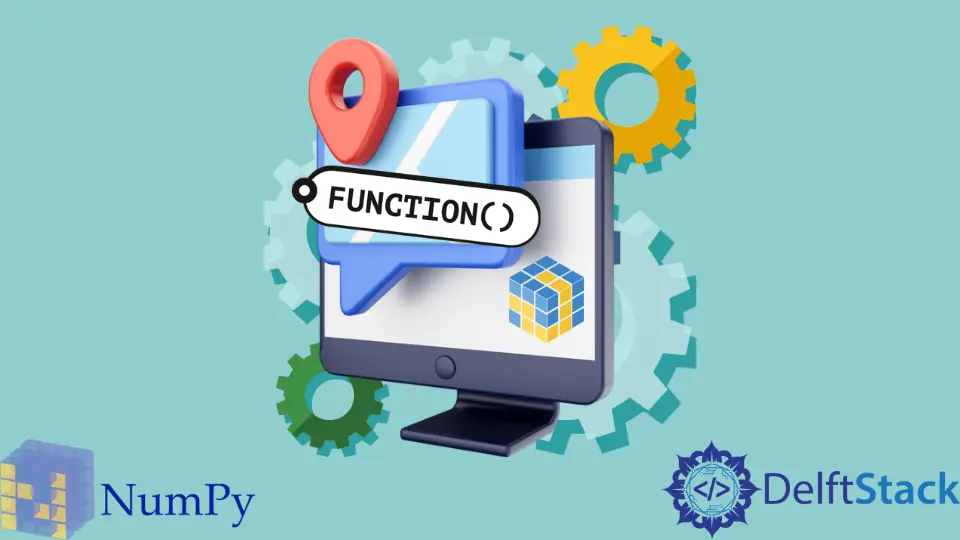
Mapping functions over arrays is a fundamental task in data manipulation and analysis. In Python, NumPy provides powerful tools to achieve this efficiently. When working with large datasets, you might find yourself needing to apply a function to each element of a NumPy array. Fortunately, there are two main methods to accomplish this: the numpy.vectorize()
function and using the lambda
keyword. Both methods allow you to apply custom functions seamlessly, enhancing your data processing capabilities.
In this article, we will explore these methods in detail, providing clear examples to help you understand how to effectively map functions in NumPy.
Understanding numpy.vectorize()
The numpy.vectorize()
function is a convenient way to apply a function to each element of an array. It essentially transforms a regular Python function into a vectorized function, enabling it to operate element-wise on NumPy arrays. This approach is particularly useful when you have a function that is not inherently vectorized and you want to apply it across an entire array.
Here’s how you can use numpy.vectorize()
to map a function over a NumPy array:
import numpy as np
def square(x):
return x ** 2
array = np.array([1, 2, 3, 4, 5])
vectorized_square = np.vectorize(square)
result = vectorized_square(array)
print(result)
Output:
[ 1 4 9 16 25]
In this example, we define a simple function called square
, which takes a number and returns its square. We then create a NumPy array containing integers from 1 to 5. By using np.vectorize()
, we convert the square
function into a vectorized version that can operate on the entire array at once. The result is a new array containing the squares of the original integers.
Using numpy.vectorize()
is particularly advantageous when dealing with more complex functions that might not be natively compatible with NumPy’s array operations. It abstracts away the need for explicit loops, making your code cleaner and more readable. However, while vectorize
is convenient, keep in mind that it may not always be the most efficient option for very large datasets compared to other vectorized operations available in NumPy.
Using Lambda Functions for Mapping
Another effective way to map functions over a NumPy array is by utilizing the lambda
keyword. Lambda functions are anonymous functions defined with the lambda
keyword, providing a way to create small, one-off functions without the need for a formal function definition. This method is particularly useful for simple operations that can be expressed in a single line.
Here’s how to apply a lambda function to a NumPy array:
import numpy as np
array = np.array([1, 2, 3, 4, 5])
result = np.array(list(map(lambda x: x ** 2, array)))
print(result)
Output:
[ 1 4 9 16 25]
In this code snippet, we create a NumPy array with integers from 1 to 5. We then use the map()
function to apply a lambda function that squares each element of the array. The map()
function returns an iterator, so we convert it back to a NumPy array using np.array()
. The result is identical to the previous example, providing us with the squares of the original integers.
Using lambda functions with map()
is a concise and efficient way to apply simple operations across arrays. It allows for quick one-liners that can enhance code readability, especially when the operation is straightforward. However, for more complex operations, defining a full function might be more appropriate for clarity and maintainability.
Conclusion
Mapping functions over NumPy arrays is a powerful technique for data manipulation in Python. By leveraging numpy.vectorize()
and lambda functions, you can efficiently apply custom operations to your data. Each method has its strengths, with vectorize()
being ideal for complex functions and lambda functions providing a quick solution for simpler tasks. Understanding these methods will significantly enhance your ability to work with arrays in NumPy. As you become more familiar with these techniques, you’ll find that they can greatly streamline your data processing workflows.
FAQ
-
What is numpy.vectorize() used for?
numpy.vectorize() is used to convert a regular Python function into a vectorized function that can operate element-wise on NumPy arrays. -
Can I use lambda functions with NumPy arrays?
Yes, you can use lambda functions with NumPy arrays, typically in conjunction with themap()
function to apply simple operations. -
What are the advantages of using numpy.vectorize()?
The advantages include cleaner code, the ability to apply non-vectorized functions to arrays, and improved readability by avoiding explicit loops. -
Are lambda functions efficient for large datasets?
While lambda functions are concise and readable, for very large datasets, using built-in NumPy functions or vectorized operations may be more efficient. -
Can I combine both methods in my code?
Yes, you can combine both numpy.vectorize() and lambda functions in your code to achieve more complex data transformations.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn