NumPy Intersection of Two Arrays
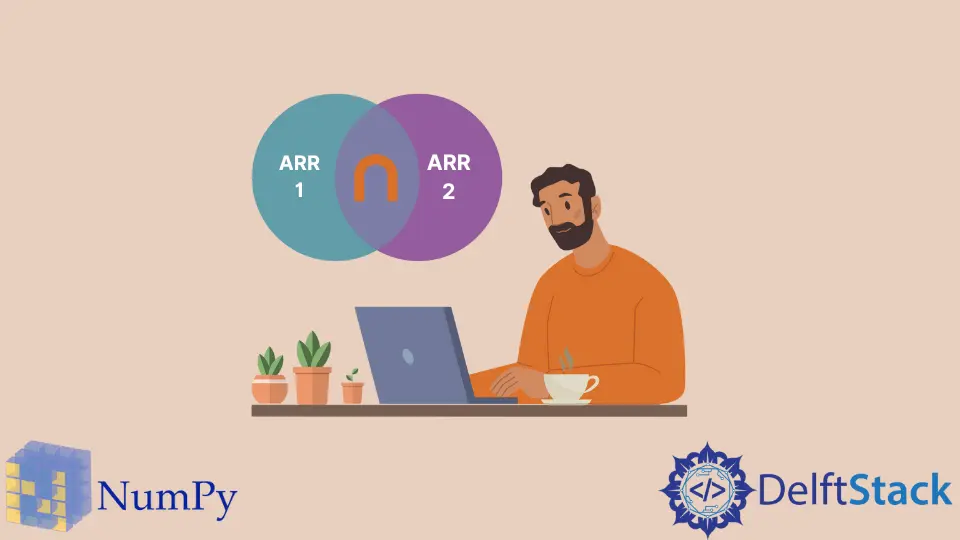
When working with data in Python, you often encounter situations where you need to find common elements between two arrays. This is particularly true in data analysis, machine learning, and scientific computing. NumPy, a powerful library for numerical computing, provides efficient methods for handling such tasks.
In this article, we will explore two primary methods to find the intersection of two 1-dimensional arrays: numpy.in1d()
and numpy.intersect1d()
. We will break down each method with clear examples, so you can easily understand how to implement them in your projects. Whether you’re a beginner or an experienced programmer, this guide will enhance your skills in data manipulation using NumPy.
Using numpy.in1d() Method
The numpy.in1d()
method is a straightforward way to test whether each element of one array is present in another. This method returns a boolean array that indicates the presence of elements from the first array in the second array. Here’s how to use it effectively.
import numpy as np
array1 = np.array([1, 2, 3, 4, 5])
array2 = np.array([3, 4, 5, 6, 7])
intersection = np.in1d(array1, array2)
print(intersection)
Output:
[False False True True True]
In the example above, we first import the NumPy library and define two arrays, array1
and array2
. The np.in1d()
function checks each element in array1
to see if it exists in array2
. The output is a boolean array indicating which elements are present: True
for elements that exist in array2
and False
for those that do not. While this method is useful for testing membership, it does not return the actual intersecting values directly. Instead, it provides a mask that you can use to extract the common elements from the original array.
To get the actual intersecting values, you can combine np.in1d()
with boolean indexing:
common_elements = array1[intersection]
print(common_elements)
Output:
[3 4 5]
This additional step yields the actual intersection of the two arrays, allowing you to work directly with the common elements.
Using numpy.intersect1d() Method
The numpy.intersect1d()
method offers a more direct approach to finding the intersection of two arrays. Unlike numpy.in1d()
, this method returns the unique values that are present in both input arrays. This can be particularly useful when you want to retrieve the intersecting elements without additional steps.
import numpy as np
array1 = np.array([1, 2, 3, 4, 5])
array2 = np.array([3, 4, 5, 6, 7])
intersection = np.intersect1d(array1, array2)
print(intersection)
Output:
[3 4 5]
In this example, we again define two arrays, array1
and array2
. The np.intersect1d()
function directly computes the intersection of both arrays. The result is an array containing the unique elements that appear in both input arrays. This method is particularly advantageous because it automatically handles duplicates and returns a sorted array of common elements.
Using numpy.intersect1d()
is typically more efficient when you need the actual intersecting values, as it simplifies your code and reduces the need for additional steps. This method is especially beneficial when dealing with larger datasets, as it is optimized for performance.
Conclusion
Finding the intersection of two arrays in Python using NumPy is a straightforward task thanks to the powerful functions it provides. Whether you choose to use numpy.in1d()
for testing membership or numpy.intersect1d()
for directly retrieving common elements, both methods have their unique advantages. Understanding these methods enhances your data manipulation capabilities and allows for more efficient coding practices. As you continue to work with NumPy, mastering these techniques will undoubtedly improve your data analysis skills and contribute to your success in various programming endeavors.
FAQ
-
What is the difference between numpy.in1d() and numpy.intersect1d()?
numpy.in1d() checks for membership and returns a boolean array, while numpy.intersect1d() returns the unique intersection of two arrays directly. -
Can I use numpy methods with multi-dimensional arrays?
The methods discussed are designed for 1-dimensional arrays. For multi-dimensional arrays, you may need to flatten them or use different approaches. -
Are there performance differences between the two methods?
Yes, numpy.intersect1d() is generally more efficient for retrieving intersecting values, while numpy.in1d() may require additional steps to achieve the same result. -
How do I handle duplicates in my arrays?
numpy.intersect1d() automatically handles duplicates by returning unique values in the intersection.
- Is NumPy the only library for array manipulation in Python?
While NumPy is one of the most popular libraries for numerical computing, there are other libraries like pandas and SciPy that offer additional functionality for array manipulation.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn