How to Get Number of Rows in NumPy
-
Get the Number of Rows in
NumPy
Array Using thearray.shape
Property -
Get the Number of Rows in
NumPy
Array Using thelen()
Function -
Get the Number of Rows in
NumPy
Array Using thenp.size()
Function - Conclusion
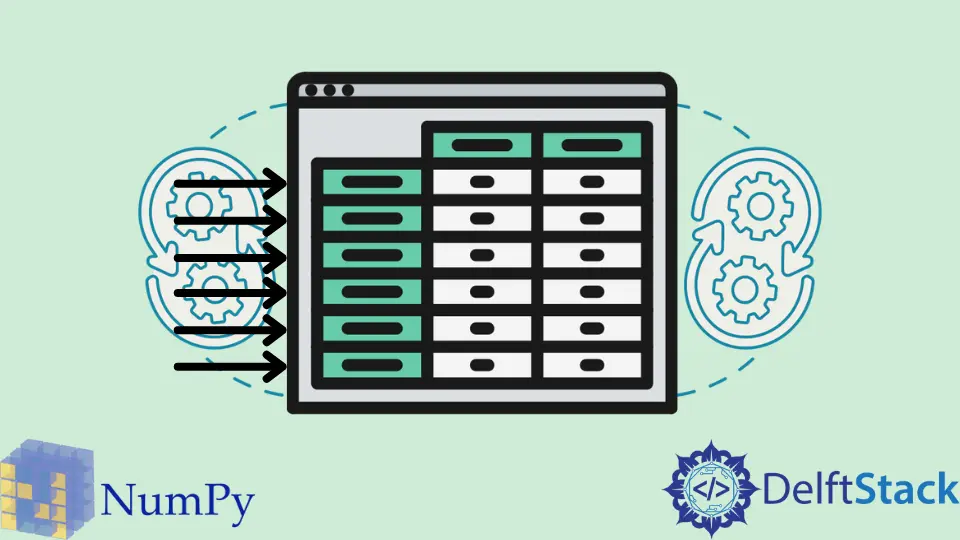
Counting the number of rows in a NumPy
array is a common requirement when working with data.
In this article, we’ll explore several methods to achieve this, including using the array.shape
property, the len()
function, and the np.size()
function.
Get the Number of Rows in NumPy
Array Using the array.shape
Property
The array.shape
property in NumPy
is used to obtain the dimensions (shape) of a NumPy
array. It returns a tuple that specifies the number of elements along each axis of the array.
Syntax:
array.shape
Parameter:
array
: TheNumPy
array for which you want to retrieve the shape information.
The following code example shows how we will get the number of rows as well as the number of columns of a 2D NumPy
array with the array.shape
property.
import numpy as np
array = np.array([[1,3,5],[2,4,6]])
rows, columns = array.shape
print("Rows = ",rows)
print("Columns = ", columns)
In the code above, we import the NumPy
library as np
. Then, we create a NumPy
array named array
, with two rows and three columns that contain integers.
Next, we use the .shape
property of the array
to get its dimensions. The property .shape
produces a tuple containing the number of rows and columns.
After that, we’re unpacking that tuple into the rows
and columns
variables. Lastly, we print the number of rows and columns in the array
.
Output:
Rows = 2
Columns = 3
In the output, we see that there are 2 rows, which represent the vertical dimension of the 2D array, and there are 3 columns, indicating the horizontal dimension of the 2D array.
Get the Number of Rows in NumPy
Array Using the len()
Function
The len()
function counts the items in a sequence, such as a tuple, list, string, or NumPy
array. When we use len()
with a NumPy
array, it gives us the number of elements in the array’s first dimension, helping us determine the number of rows in the array.
Syntax:
len(object)
Parameter:
object
: The object whose length you want to determine.
The following code example explains how to use the len()
function to determine the number of rows in a 2D NumPy
array.
import numpy as np
array = np.array([[1, 3, 5], [2, 4, 6]])
num_rows = len(array)
print("Rows = ", num_rows)
In the code above, we import the NumPy
library as np
.
Then, we create a NumPy
array called array
by using the np.array()
function. This array is a 2D array with two rows and three columns containing integer values.
After that, we use the built-in len()
function to determine the number of elements in the array
. When applied to a NumPy
array, it counts the number of elements in the first dimension, which corresponds to the number of rows.
Lastly, we print the number of rows in the array
.
Output:
Rows = 2
In the output, we can see that there are 2 rows in the array
. This indicates that the array has a vertical dimension with two rows.
Get the Number of Rows in NumPy
Array Using the np.size()
Function
The np.size()
function in NumPy
is used to find the total number of elements in an array, which can be a useful way to determine the number of rows in a NumPy
array. When applied to a NumPy
array, it returns the total number of elements in the array, regardless of the number of dimensions.
Syntax:
np.size(a, axis=None)
Parameters:
a
: TheNumPy
array for which you want to find the number of elements.axis
(optional): An integer or tuple specifying the axis or axes along which the size should be calculated. If not provided, it calculates the total number of elements in the array. If specified, it calculates the size along the specified axis or axes.
The following code example explains how to use the np.size()
Function to determine the number of rows in a 2D NumPy
array.
import numpy as np
array = np.array([[1, 3, 5], [2, 4, 6]])
num_rows = np.size(array, 0)
print("Rows = ", num_rows)
In the code above, we import the NumPy
library as np
.
Then, we create a NumPy
array named array
using the np.array()
function. This array is a 2D array with two rows and three columns containing integer values.
Next, we use the np.size()
function to count the number of elements along a specified axis. In this case, we specify axis=0
to count elements along the first dimension, which corresponds to the number of rows.
Lastly, we print the number of rows in the array
.
Output:
Rows = 2
The output displays that there are 2 rows in the array
. The np.size()
function with axis=0
counts the number of elements along the first dimension, which signifies the number of rows in the 2D array.
Conclusion
In this article, we’ve covered different approaches to calculate the number of rows in a NumPy
array, a fundamental task in data analysis. We discussed the array.shape
property, the len()
function, and the np.size()
function, giving us the adaptability to change according to the requirements of your data analysis and manipulation tasks.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn