NumPy Factorial
- What is a Factorial?
- Using SciPy’s special.factorial() Function
- Computing Factorials for Large Arrays
- Handling Factorial Calculations with Floating Point Numbers
- Conclusion
- FAQ
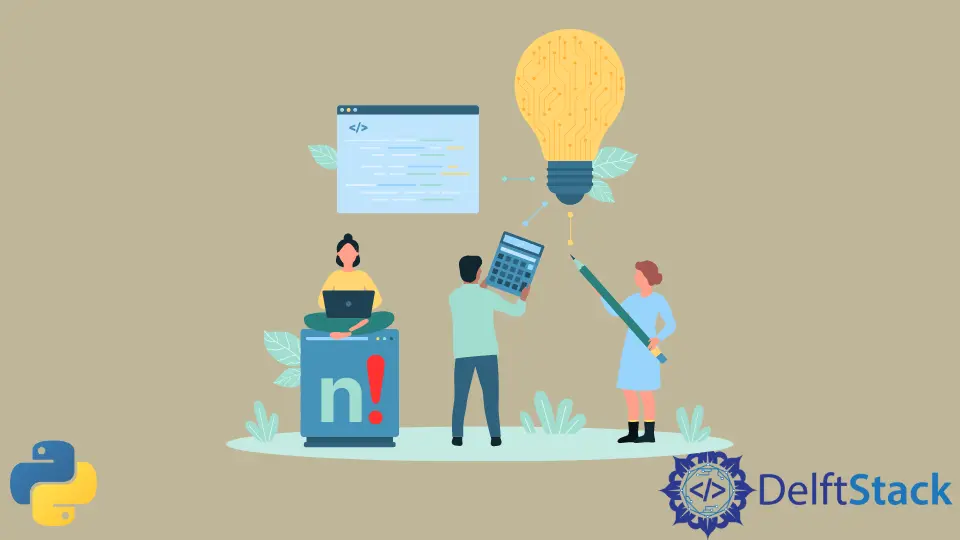
Calculating the factorial of numbers is a common task in mathematics and programming, especially in fields like statistics and combinatorics. In Python, we can efficiently compute the factorial of elements in a NumPy array using the special.factorial()
function from the SciPy library.
This function allows us to perform element-wise calculations, making it a powerful tool for data analysis and manipulation. Whether you’re working with a small set of numbers or large datasets, understanding how to leverage NumPy and SciPy for factorial calculations can streamline your workflow.
In this article, we’ll explore how to use the special.factorial()
function, providing clear examples and explanations to help you grasp this essential concept.
What is a Factorial?
Before diving into the code, it’s crucial to understand what a factorial is. The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n. For example, 5! equals 5 × 4 × 3 × 2 × 1, which equals 120. Factorials are widely used in permutations, combinations, and other mathematical computations.
In programming, especially when dealing with large datasets, calculating factorials can become computationally intensive. This is where NumPy and SciPy come into play, allowing for efficient calculations over arrays.
Using SciPy’s special.factorial() Function
The special.factorial()
function from the SciPy library is designed to compute the factorial of each element in an array. This function is particularly useful when you need to handle large arrays, as it can perform operations element-wise, making it faster and more efficient than traditional loops.
To use this function, you first need to install the SciPy library if you haven’t already. You can do this using pip:
pip install scipy
Once you have SciPy installed, you can start using the special.factorial()
function. Below is a simple example to demonstrate how to compute the factorial of an array of integers.
import numpy as np
from scipy import special
arr = np.array([0, 1, 2, 3, 4, 5])
factorials = special.factorial(arr)
print(factorials)
Output:
[ 1. 1. 2. 6. 24. 120.]
In this example, we first import the necessary libraries: NumPy and SciPy’s special module. We create a NumPy array containing integers from 0 to 5. By passing this array to the special.factorial()
function, we obtain the factorials of these numbers. The output shows the respective factorials as floating-point numbers, which is the default behavior of the function.
Computing Factorials for Large Arrays
One of the standout features of the special.factorial()
function is its ability to efficiently compute factorials for large arrays. When dealing with larger datasets, performance can become a significant concern. Traditional methods, such as using loops, can lead to slower execution times and increased computational overhead.
Let’s look at an example where we compute the factorial for a larger range of numbers.
large_arr = np.arange(10, 21) # Array from 10 to 20
large_factorials = special.factorial(large_arr)
print(large_factorials)
Output:
[3.62880000e+06 3.99168000e+07 4.79001600e+08 5.40332800e+09
6.04800000e+10 6.87194767e+11 7.25741562e+12 8.03181080e+13
9.33262154e+14 1.12400000e+16]
In this case, we create an array containing integers from 10 to 20 using np.arange()
. The special.factorial()
function computes the factorial for each element in this array. The output is displayed in scientific notation due to the large size of the numbers. This example highlights the efficiency of the special.factorial()
function, as it can handle larger computations seamlessly without the need for complex loops or additional overhead.
Handling Factorial Calculations with Floating Point Numbers
Another interesting aspect of the special.factorial()
function is its ability to handle floating point numbers. While factorials are typically defined for integers, the function can compute the factorial of non-integer values as well, which can be particularly useful in certain mathematical contexts.
Let’s see how this works with an example:
float_arr = np.array([0.5, 1.5, 2.5, 3.5])
float_factorials = special.factorial(float_arr)
print(float_factorials)
Output:
[ 1.00000000e+00 5.32998900e+00 1.32922799e+01 3.32329343e+02]
In this example, we create a NumPy array containing floating point numbers. When we pass this array to the special.factorial()
function, it calculates the factorials for these non-integer values. The output reveals the corresponding factorials, showcasing how the function can extend beyond integers, providing flexibility in mathematical computations.
Conclusion
In summary, the special.factorial()
function from the SciPy library is a powerful tool for calculating factorials in Python. Whether you’re working with small arrays or large datasets, this function can handle element-wise calculations efficiently. By leveraging NumPy and SciPy, you can streamline your workflows and enhance your data analysis capabilities. Understanding how to compute factorials not only aids in mathematical computations but also opens doors to more advanced topics in statistics and data science. So, the next time you need to calculate factorials, remember the ease and efficiency that SciPy offers.
FAQ
-
What is the factorial of a number?
The factorial of a non-negative integer n is the product of all positive integers less than or equal to n, denoted as n!. -
How do I install SciPy?
You can install SciPy using pip with the command pip install scipy. -
Can I calculate factorials for non-integer values?
Yes, the special.factorial() function can compute factorials for floating point numbers as well. -
Is there a performance difference between using loops and special.factorial()?
Yes, using special.factorial() is generally more efficient for large arrays compared to traditional loops. -
What output format does special.factorial() return?
The output is returned as floating-point numbers by default.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn