How to Delete Row in NumPy
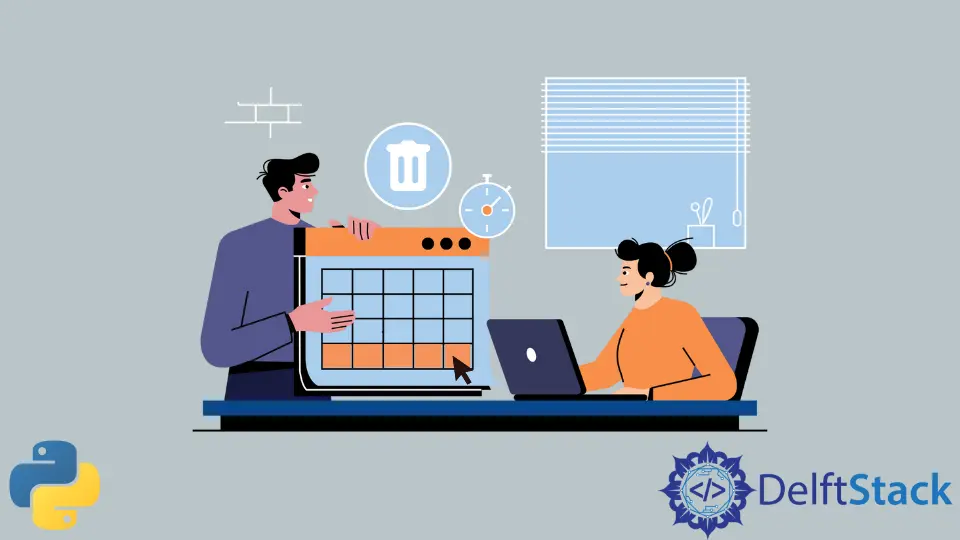
When working with multi-dimensional arrays in Python, NumPy is an indispensable library. One common task you might encounter is the need to delete a row from a NumPy array. Whether you’re cleaning up your data or simply manipulating arrays for analysis, understanding how to use the numpy.delete()
function is crucial. This function not only allows you to remove rows but also provides flexibility in how you manage your data structures.
In this article, we will explore various methods to delete a row in NumPy, complete with clear examples and explanations. By the end, you’ll be equipped with the knowledge to handle row deletions effectively in your data analysis projects.
Using numpy.delete()
Function
The most straightforward way to delete a row in a NumPy array is by using the numpy.delete()
function. This function takes three primary arguments: the array you want to modify, the index of the row you wish to remove, and the axis along which to perform the operation.
Here’s a simple example:
import numpy as np
array = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
new_array = np.delete(array, 1, axis=0)
print(new_array)
Output:
[[1 2 3]
[7 8 9]]
In this example, we start with a 3x3 array. By calling np.delete(array, 1, axis=0)
, we remove the row at index 1, which corresponds to the second row of the original array. The axis=0
parameter indicates that we are working along the rows. The resulting new_array
now contains only the first and third rows.
This method is highly effective for quickly removing a row, but keep in mind that it creates a new array rather than modifying the original one. This behavior is typical in NumPy, where immutability is a common practice to avoid unintentional data loss.
Deleting Multiple Rows
If you need to delete more than one row, numpy.delete()
can handle that too. You can provide a list of indices to remove multiple rows at once.
Here’s how to do it:
import numpy as np
array = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
[10, 11, 12]])
new_array = np.delete(array, [1, 3], axis=0)
print(new_array)
Output:
[[1 2 3]
[7 8 9]]
In this scenario, we have a 4x3 array. By passing the list [1, 3]
to np.delete()
, we remove the second and fourth rows from the original array. The result is a new array that only includes the first and third rows. This method is particularly useful when you have specific rows that you want to eliminate, allowing for greater flexibility in data manipulation.
Conditional Row Deletion
Sometimes, you may want to delete rows based on specific conditions rather than by index. For instance, if you want to remove all rows where a certain condition is met (like a value being less than a threshold), you can use boolean indexing.
Here’s an example:
import numpy as np
array = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
[10, 11, 12]])
condition = array[:, 0] > 5
new_array = array[condition]
print(new_array)
Output:
[[ 6 7 8]
[ 9 10 11]
[12 13 14]]
In this example, we create a boolean condition that checks if the first column values are greater than 5. We then use this condition to index the original array. The resulting new_array
contains only the rows that satisfy the condition. This method is particularly powerful for data filtering, enabling you to remove undesirable rows based on dynamic criteria rather than static indices.
Conclusion
Deleting rows in NumPy is a fundamental operation that can greatly enhance your data manipulation capabilities. Whether you choose to use the numpy.delete()
function, remove multiple rows, or apply conditional logic, understanding these methods will empower you to manage your datasets more effectively. As you continue to work with NumPy, remember that each method has its unique advantages, so choose the one that best fits your specific needs. With practice, you’ll become adept at handling arrays and performing complex data operations with ease.
FAQ
-
what is the numpy.delete() function used for?
The numpy.delete() function is used to remove elements from a NumPy array along a specified axis. -
can I delete multiple rows at once in NumPy?
Yes, you can delete multiple rows by passing a list of indices to the numpy.delete() function. -
how do I delete rows based on a condition in NumPy?
You can use boolean indexing to filter rows based on specific conditions, allowing you to remove rows dynamically. -
does numpy.delete() modify the original array?
No, numpy.delete() creates a new array with the specified rows removed, leaving the original array unchanged. -
what happens if I try to delete a row index that doesn’t exist?
If you attempt to delete a row index that is out of bounds, NumPy will raise an IndexError.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn