NumPy Deep Copy
- Understanding Deep Copy in NumPy
- Method 1: Using copy.deepcopy()
- Method 2: User-Defined Deep Copy Approach
- Conclusion
- FAQ
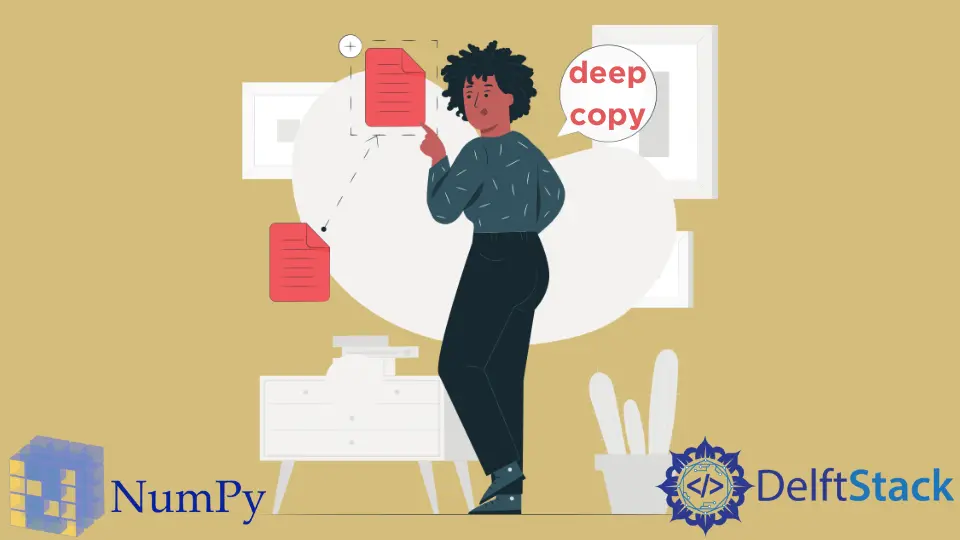
When working with NumPy, the ability to create deep copies of arrays is essential for efficient data manipulation and analysis. Deep copying ensures that changes made to one array do not affect another, allowing for safer data handling in various applications, from scientific computing to machine learning.
In this article, we will explore two main methods for achieving deep copies of NumPy arrays in Python: using the built-in copy.deepcopy()
function and a user-defined approach. By the end, you’ll have a solid understanding of how to implement these techniques effectively.
Understanding Deep Copy in NumPy
Deep copying in NumPy is a crucial concept that prevents unintended side effects when modifying arrays. Unlike shallow copies, where modifications to one array can impact another, deep copies create entirely independent duplicates. This is particularly important when working with complex data structures or when you need to preserve the original data while experimenting with modifications.
In Python, the copy
module provides a straightforward way to create deep copies, while custom methods can offer more control and flexibility. Let’s dive into the specifics of each method.
Method 1: Using copy.deepcopy()
The simplest way to create a deep copy of a NumPy array is by utilizing the copy.deepcopy()
function from the copy
module. This method is effective for duplicating any object, including NumPy arrays. Here’s how you can implement it:
import numpy as np
import copy
original_array = np.array([[1, 2, 3], [4, 5, 6]])
deep_copied_array = copy.deepcopy(original_array)
deep_copied_array[0, 0] = 99
print("Original Array:")
print(original_array)
print("\nDeep Copied Array:")
print(deep_copied_array)
Output:
Original Array:
[[1 2 3]
[4 5 6]]
Deep Copied Array:
[[99 2 3]
[ 4 5 6]]
In this example, we first import the necessary modules and create an original NumPy array. We then use copy.deepcopy()
to create a deep copy of that array. After modifying the first element of the deep copied array, we print both arrays to show that the original remains unchanged. This method is straightforward and works well for most scenarios.
Using copy.deepcopy()
is beneficial because it handles complex nested structures seamlessly. However, it may be less efficient for large arrays compared to NumPy’s built-in functions. It’s essential to consider the trade-offs between ease of use and performance when choosing this method.
Method 2: User-Defined Deep Copy Approach
While copy.deepcopy()
is convenient, you might want to create a deep copy using a more tailored approach, especially if you are working with large datasets or require specific handling. You can achieve this using the NumPy np.copy()
function, which is optimized for NumPy arrays.
import numpy as np
original_array = np.array([[1, 2, 3], [4, 5, 6]])
deep_copied_array = np.copy(original_array)
deep_copied_array[0, 0] = 99
print("Original Array:")
print(original_array)
print("\nDeep Copied Array:")
print(deep_copied_array)
Output:
Original Array:
[[1 2 3]
[4 5 6]]
Deep Copied Array:
[[99 2 3]
[ 4 5 6]]
In this example, we again start with an original NumPy array. Instead of using copy.deepcopy()
, we utilize np.copy()
, which is specifically designed for NumPy arrays. After modifying the deep copied array, we print both arrays to confirm that the original remains intact.
The np.copy()
function is highly efficient, especially for large datasets. It creates a deep copy of the array, ensuring that any changes to the copied array do not affect the original. This method is often preferred in performance-sensitive applications where speed and memory efficiency are critical.
Conclusion
Understanding how to create deep copies of NumPy arrays is a vital skill for any Python programmer working with data. Whether you choose to use the copy.deepcopy()
function for its simplicity or the np.copy()
method for its performance, both approaches ensure that you can manipulate data safely without unintended side effects. With this knowledge, you can confidently handle data in your projects, knowing that your original datasets remain untouched.
FAQ
-
what is the difference between shallow copy and deep copy?
A shallow copy creates a new object but does not create copies of nested objects, while a deep copy creates a completely independent copy of the entire object, including nested structures. -
when should I use np.copy() over copy.deepcopy()?
Use np.copy() when working with NumPy arrays for better performance, especially with large datasets. Use copy.deepcopy() for more complex objects or when you need to copy non-NumPy structures. -
can I deep copy a multi-dimensional NumPy array?
Yes, both copy.deepcopy() and np.copy() can create deep copies of multi-dimensional NumPy arrays without any issues. -
does deep copying affect performance?
Yes, deep copying can affect performance, especially for large datasets. np.copy() is generally faster and more memory-efficient for NumPy arrays compared to copy.deepcopy(). -
are there any limitations to using copy.deepcopy() with NumPy arrays?
While copy.deepcopy() works well, it may not be as efficient as np.copy() for large arrays, as it handles more complex data structures and may introduce unnecessary overhead.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn