How to Create a Simple Click Counter Using JavaScript
- Click Counter on a Button Using JavaScript
- Click Counter on a div Using JavaScript
- Click Counter - The Unobstructive Approach
- Remarks
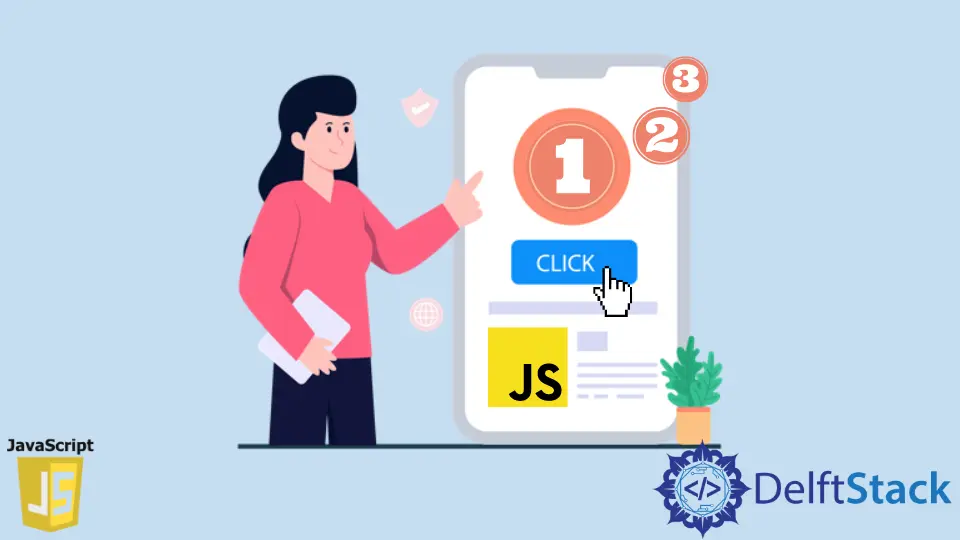
A click counter records the number of clicks and displays it on the screen. The counter makes use of the JavaScript click
event to increment the counter value. We can use it in various areas, including in games (to increase the points or score value) and in some time-saving hacks. Let us look at the code to create a simple click counter in JavaScript.
Click Counter on a Button Using JavaScript
<h3><center>JavaScript Click Counter</center></h3>
<div>
<center><h3 id="counter-label">0</h3></center>
</div>
<center>
<div>
<button onclick="incrementClick()">Click Me</button>
<button onclick="resetCounter()">Reset</button>
</div>
</center>
var counterVal = 0;
function incrementClick() {
updateDisplay(++counterVal);
}
function resetCounter() {
counterVal = 0;
updateDisplay(counterVal);
}
function updateDisplay(val) {
document.getElementById('counter-label').innerHTML = val;
}
In the code, we increment the value of the counter variable counterVal
by 1
on each user click. The steps are as follows.
-
We create a counter display in the HTML with
<h3>
tag and assign it an idcounter-label
so that it can be updated from the javascript code by thedocument.getElementById()
function in javascript. -
In the HTML, we also add a couple of buttons. One button with the
Click Me
text, which on click increases the counter value by1
. Similarly, in the other button, we have aReset
text in the HTML that will reset the counter to0
. -
On clicking the
click me
button, we need to increment the counter value. For that, we use theonclick
event listener. And trigger theincrementClick()
function with the click of theClick Me
button. -
Inside the
incrementClick()
function, we increment the global variablecounterVal
by 1 and call theupdateDisplay
function. -
On clicking of the
Reset
button, we call theresetCounter()
function. In this function we reset the global counter value (counterVal
) to0
and call theupdateDisplay()
function. -
In the
updateDisplay()
function we display the value it recieves as a parameter, into the<h3>
tag which holds the counter value. It uses thedocument.getElementById()
function of javascript to query for the HTML element with idcounter-label
and then updates the innerHTML attribute with the new counter value. TheinnerHTML
attribute further changes the text that is displayed in the HTML GUI Interface.
The above code sample displays the click counter and increments it on a button click. In most use cases, we may not apply the click counter always on a button. To make it flexible, we will have to support the feature for other HTML elements such as div
, li
etc. Almost all HTML elements have the click
event with the onclick
event handler.
Click Counter on a div Using JavaScript
In games, the scenes don’t always have a button to trigger the counter increment. Mostly it may be made up of HTML elements like div
. In such cases, we can use the onclick
event handler of the div
tag to capture the click for incrementing the counter value. Refer to the following code sample.
<h3><center>JavaScript Click Counter</center></h3>
<div>
<center><h3 id="counter-label">0</h3></center>
</div>
<center>
<div>
<div onclick="incrementClick()">Click Me</div>
<button onclick="resetCounter()">Reset</button>
</div>
</center>
var counterVal = 0;
function incrementClick() {
updateDisplay(++counterVal);
}
function resetCounter() {
counterVal = 0;
updateDisplay(counterVal);
}
function updateDisplay(val) {
document.getElementById('counter-label').innerHTML = val;
}
You may observe that in this example we have used div
in the line <div onclick="incrementClick()">Click Me</div>
. With this change, the incrementClick()
gets triggered on a click of the div instead of the button. The code will work fine with HTML tags like <li>
, <a>
, <div>
etc. as well. Almost all the HTML tags that support the onclick
event handlers can be associated with an onclick
listener and worked upon similarly.
Click Counter - The Unobstructive Approach
If we implement the click counter with an unobstructed approach in JavaScript, we will need to remove the onclick
event handler in HTML and move it to the JavaScript code. The code structure looks a bit different though, observe the following sample code.
<h3><center>JavaScript Click Counter</center></h3>
<div>
<center><h3 id="counter-label">0</h3></center>
</div>
<center>
<div>
<div id="click-div">Click Me</div>
<button id="reset-button">Reset</button>
</div>
</center>
window.onload =
function() {
let clickDiv = document.getElementById('click-div');
clickDiv.onclick = incrementClick;
let resetBtn = document.getElementById('reset-button');
resetBtn.onclick = resetCounter;
}
var counterVal = 0;
incrementClick =
function() {
updateDisplay(++counterVal);
}
function resetCounter() {
counterVal = 0;
updateDisplay(counterVal);
}
function updateDisplay(val) {
document.getElementById('counter-label').innerHTML = val;
}
Here, we bind the click events into JavaScript code. The structure remains the same, except that we use an onclick
listener to the Click Me
div and the Reset
button. This binding takes place at the run time. And to that click listener, we bind our defined functions. So the code looks similar and does not have many changes.
Remarks
The difference between the unobstructed approach is that we bind the onclick
listener in the javascript code rather than doing it in the HTML. It will be hard to realize from the HTML alone if the button or the div is assigned an onclick
event handler.
With this approach, the code looks cleaner as all the onclick
events are bound and handled in the JavaScript. It keeps the HTML clean with the markup as no JavaScript code intrudes in HTML.
The code will not work if we do not set the onclick
listener within the window.onload
function. The document.getElementById("click-div")
code will return null
as it will not be able to find the HTML element. The wondow.onload
function gets triggered once the window is loaded and the DOM is ready. Hence by putting the code inside the window.onload
block ensures the HTML element is loaded and can be queried with the document.getElementById()
function of JavaScript.