How to Remove Event Listener in JavaScript
- Understanding Event Listeners
-
The
removeEventListener
Method - Using Anonymous Functions
- Event Delegation
- Conclusion
- FAQ
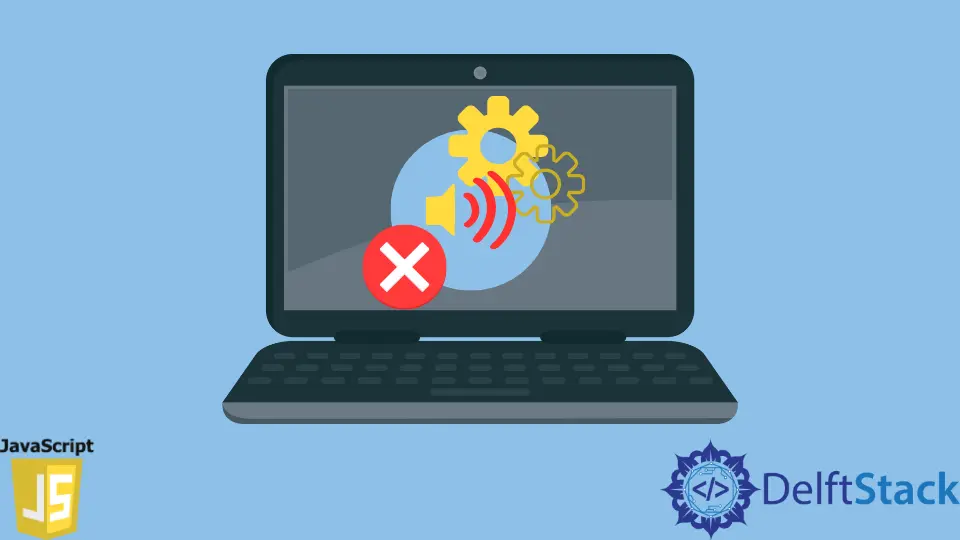
When working with JavaScript, managing event listeners is crucial for creating responsive web applications. An event listener allows your code to react to user interactions, like clicks or keyboard inputs. However, there are times when you might need to remove an event listener to optimize performance or prevent memory leaks.
In this tutorial, we will explore how to effectively remove event listeners in JavaScript, ensuring your application runs smoothly. By the end, you’ll have a solid understanding of the methods available for removing event listeners and when to use each one.
Understanding Event Listeners
Before we dive into removing event listeners, let’s clarify what they are. An event listener is a function that waits for a specific event to occur on a target element. For instance, you might want to trigger a function when a user clicks a button. Adding an event listener is straightforward, but it’s equally important to know how to remove one when it’s no longer needed.
Removing event listeners is essential for maintaining performance and preventing memory leaks. When you add an event listener, it holds a reference to the function you provided. If you don’t remove it when it’s no longer needed, it can lead to unintended behavior, such as multiple event triggers or increased memory usage.
The removeEventListener
Method
The primary method for removing an event listener in JavaScript is removeEventListener
. This method requires two parameters: the event type and the function reference that was used when the listener was added. Here’s how you can use it effectively.
Example: Removing an Event Listener
const button = document.getElementById('myButton');
function handleClick() {
console.log('Button clicked!');
}
button.addEventListener('click', handleClick);
// Later in the code, when you want to remove the listener
button.removeEventListener('click', handleClick);
In this example, we first select a button element and define a function handleClick
that logs a message when the button is clicked. We then add the event listener using addEventListener
. When we want to stop listening for clicks, we call removeEventListener
, passing in the same event type and function reference. It’s crucial to use the same function reference; otherwise, the event listener won’t be removed.
Using Anonymous Functions
One common mistake is using anonymous functions when adding event listeners. If you do this, you won’t be able to remove the listener later since you won’t have a reference to the function.
Example: Issue with Anonymous Functions
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
console.log('Button clicked!');
});
// Attempting to remove the listener
button.removeEventListener('click', function() {
console.log('Button clicked!');
}); // This won't work
In this case, the removeEventListener
call fails because the function passed to it is a different instance than the one added. To avoid this, always use named functions when adding event listeners if you plan to remove them later.
Event Delegation
Another useful technique is event delegation, which allows you to manage event listeners more efficiently. Instead of adding an event listener to each child element, you can add one listener to a parent element. This way, you can control events for multiple child elements without needing to add and remove listeners for each one.
Example: Event Delegation
const list = document.getElementById('myList');
function handleListClick(event) {
if (event.target.tagName === 'LI') {
console.log('List item clicked:', event.target.textContent);
}
}
list.addEventListener('click', handleListClick);
// Later, if you want to remove the listener
list.removeEventListener('click', handleListClick);
In this scenario, we add a single event listener to the parent ul
element. The handleListClick
function checks if the clicked target is a list item (LI
). This method is efficient because it reduces the number of event listeners needed. When you want to remove the listener, you simply call removeEventListener
on the parent element, just like before.
Conclusion
Removing event listeners in JavaScript is a vital skill for any web developer. By understanding how to use removeEventListener
effectively, you can manage your application’s performance and avoid potential issues with memory leaks. Remember to always use named functions when you plan to remove listeners later, and consider event delegation for efficient event management. With these techniques, your JavaScript applications will be more robust and responsive.
FAQ
-
How do I know if an event listener is active?
You can check if an event listener is active by keeping track of the function references you’ve added. There isn’t a built-in method to check active listeners directly. -
Can I remove multiple event listeners at once?
No, you need to callremoveEventListener
for each listener you want to remove individually. -
What happens if I try to remove an event listener that doesn’t exist?
If you attempt to remove a listener that wasn’t added, nothing happens, and no error is thrown.
-
Is it necessary to remove event listeners?
While it’s not always necessary, removing event listeners can help prevent memory leaks and improve performance, especially in single-page applications. -
Can I remove an event listener from a different context?
No, you must callremoveEventListener
on the same element where the listener was added, using the same function reference.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn