How to Create a JSON Response in Django
-
Create a JSON Response Using Django’s In-Build Class
JsonResponse
-
Convert
QuerySet
to a Python Dictionary and Create a JSON Response -
Create a JSON Response Using Django’s In-Build Class
HttpResponse
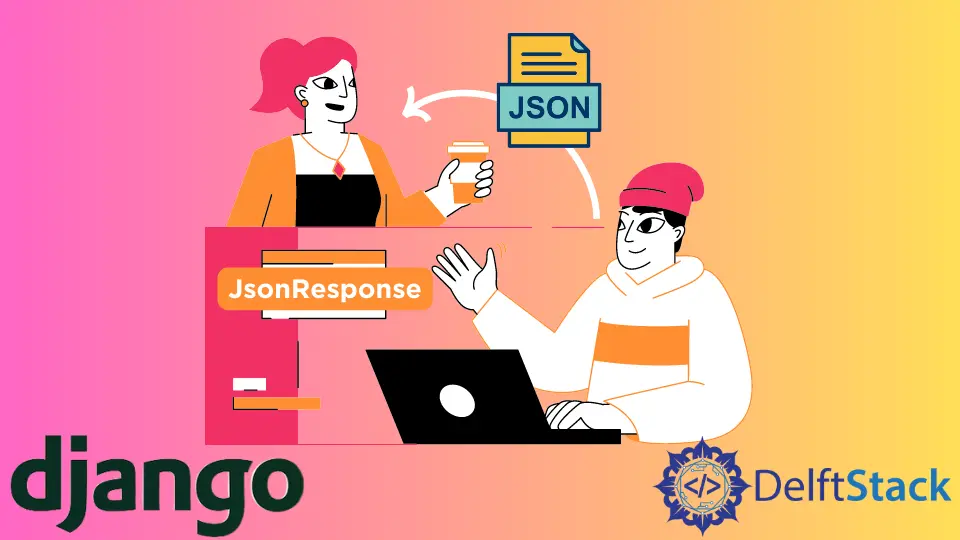
When working with APIs or in general, we sometimes have to send data from the server to the client in the form of JSON (JavaScript Object Notation). Especially with APIs, JSON is a mandatory form in which data is sent or retrieved.
In Django, working with JSON Responses is relatively easy. There are actually two ways in which we can create JSON Responses.
Create a JSON Response Using Django’s In-Build Class JsonResponse
JsonResponse
, as the name suggests, is used to create a JSON Response. This class’s constructor accepts a Python dictionary as a parameter and creates a JSON Response based on that dictionary.
The simplest possible example is as follows.
from django.views import View
from django.http import JsonResponse
# Function based view
def myView(request):
data = {
"name": "Vaibhav",
"age": 20,
"hobbies": ["Coding", "Art", "Gaming", "Cricket", "Piano"],
}
return JsonResponse(data)
# Class based view
class MyView(View):
def get(self, request):
data = {
"name": "Vaibhav",
"age": 20,
"hobbies": ["Coding", "Art", "Gaming", "Cricket", "Piano"],
}
return JsonResponse(data)
But not all the glitter is gold. JsonResponse
throws an error when dealing with a QuerySet
or any Django model.
QuerySet
is not a Python dictionary. If we have to send or share a QuerySet
or a Django model in a JSON Response, it must be converted to a Python dictionary.
Now, there are two ways in which we can do this. First, create a function that accepts a Django model or an array of QuerySet
and converts them into a Python dictionary or an array of Python dictionaries. The second option is to use Django serializers. This tutorial will only talk about the custom function because serializers is a big topic itself.
If you want, you can learn about serializers here.
Convert QuerySet
to a Python Dictionary and Create a JSON Response
For the sake of explanation, we’ll consider a simple Django model and learn how to share such a model with a JSON Response.
Consider the following model.
from django.db import models
from django.contrib.auth.models import User
class Blog(models.Model):
author = models.ForeignKey(User, on_delete=models.CASCADE, related_name="blogs")
title = models.CharField(max_length=250)
description = models.CharField(max_length=500)
showcaseImage = models.ImageField(upload_to="Images/")
dateTimeOfCreation = models.DateTimeField(auto_now=True)
shareURL = models.URLField()
likes = models.IntegerField()
disLikes = models.IntegerField()
bookmarks = models.IntegerField()
def __str__(self):
return self.title
If we have to convert this model into a Python dictionary and send it in a JSON Response, we can create a utility function that handles the conversion. Then inside the views, we can create a dictionary and add a key-value pair for the converted Python dictionary and then create a JsonResponse
.
Refer to the following code.
from django.views import View
from django.http import JsonResponse
from . import models
def blogToDictionary(blog):
"""
A utility function to convert object of type Blog to a Python Dictionary
"""
output = {}
output["title"] = blog.title
output["description"] = blog.description
output["showcaseImage"] = blog.showcaseImage.url
output["dateTimeOfCreation"] = blog.dateTimeOfCreation.strftime(
"%m/%d/%Y, %H:%M:%S"
)
output["shareURL"] = blog.shareURL
output["likes"] = blog.likes
output["disLikes"] = blog.disLikes
output["bookmarks"] = blog.bookmarks
return output
# Function based view
def myView(request):
# Single Blog
blog = models.Blog.objects.get(id=1)
# Multiple Blogs
blogs = models.Blog.objects.all()
tempBlogs = []
# Converting `QuerySet` to a Python Dictionary
blog = blogToDictionary(blog)
for i in range(len(blogs)):
tempBlogs.append(
blogToDictionary(blogs[i])
) # Converting `QuerySet` to a Python Dictionary
blogs = tempBlogs
data = {"blog": blog, "blogs": blogs}
return JsonResponse(data)
In the above code, we created a utility function blogToDictionary()
that handles the conversion of an object of type Blog
. This function iterates over all the fields, stored them in a dictionary, and finally, returned the dictionary. Then, inside the view, we converted the fetched objects and created a JsonResponse
.
Create a JSON Response Using Django’s In-Build Class HttpResponse
We can also create a JSON response using HttpResponse
. The procedure is pretty much the same. We first create a dictionary of the data we wish to send. Then we make a class HttpResponse
object. We set the content_type
as application/json
and convert the Python dictionary to JSON using Python’s in-build library json
. Lastly, we send the converted dictionary with the HttpResponse
.
import json
from django.views import View
from django.http import JsonResponse
# Function based view
def myView(request):
data = {
"name": "Vaibhav",
"age": 20,
"hobbies": ["Coding", "Art", "Gaming", "Cricket", "Piano"],
}
return HttpResponse(json.dumps(data), content_type="application/json")
# Class based view
class MyView(View):
def get(self, request):
data = {
"name": "Vaibhav",
"age": 20,
"hobbies": ["Coding", "Art", "Gaming", "Cricket", "Piano"],
}
return HttpResponse(json.dumps(data), content_type="application/json")
As mentioned above, a QuerySet
or a Django model must be converted to a Python dictionary before sending it using this method.