How to Return JSON in Django
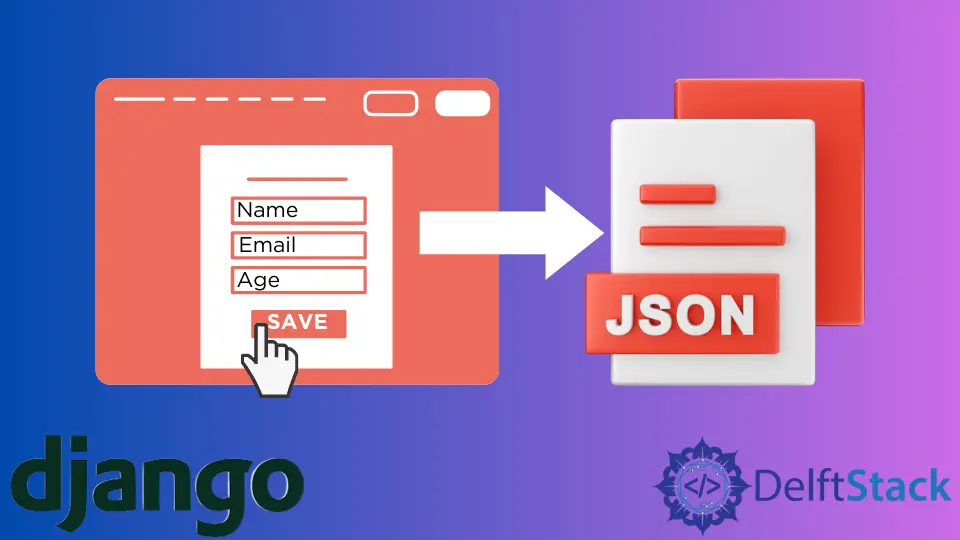
This discussion will look at a short introduction to JSON format and convert data into JSON format using the JsonResponse
class in Django.
Use the JsonResponse
Class to Return Data Into JSON Format in Django
JSON is also known as JavaScript object notation, and it is simply data representation. It is used widely across the internet for almost every single API.
JSON is extremely lightweight to send data on the client site. It also integrates very nicely with JavaScript.
Since JSON is just a superset of JavaScript, it integrates nicely with JavaScript. Almost every major language has a library or built-in functionality to parse JSON strings into objects or classes.
To pass data in JSON format is extremely easy in Django; let’s see how it works.
Let’s start by creating a Profile
model. We will create three fields name
, email
and bio
in this model. These fields would be CharField
with 1000 max_length
.
class Profile(models.Model):
name = models.CharField(max_length=1000)
email = models.CharField(max_length=1000)
bio = models.CharField(max_length=1000)
We need to register the Profile
model in the admin.py
file.
from .models import Book, Profile
admin.site.register(Book)
admin.site.register(Profile)
We need to create a new URL, so it will call the json
function from the views.py
file whenever we hit this URL.
urlpatterns = [
path("admin/", admin.site.urls),
path("json", views.json, name="json"),
]
Now we need to create a new function that is json
. First, we need to import JsonResponse
and other required modules.
from .models import Profile
from django.http import JsonResponse
In the json
function, we get values from Profile
objects using objects.values()
and convert them into a list data type.
After creating the data
variable, we need to return data in JSON response. After passing a data
variable in the JsonResponse
class, we must make sure that we pass the safe
argument and set it equal to False
.
def json(request):
data = list(Profile.objects.values())
return JsonResponse(data, safe=False)
To create our model, we need to make the migration. The following commands will allow us to see our models in the admin panel.
python manage.py makemigrations
python manage.py migrate
Now everything will work fine, just run the server using this command and see what happens.
python manage.py runserver
We will run the browser and go through with this address http://127.0.0.1:8000/admin
to the admin panel. If we open our model the first time, we need to add the model using the top-right button.
After filling the fields, we need to save this object and go to the JSON page through http://127.0.0.1:8000/json
.
[
{
id: 1,
name: "jeams parker",
email: "exampl@example.com",
bio: "This is the example bio"
}
]
Now we can access data in JSON format.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn