NumPy Tutorial - NumPy Math Operation and Broadcasting
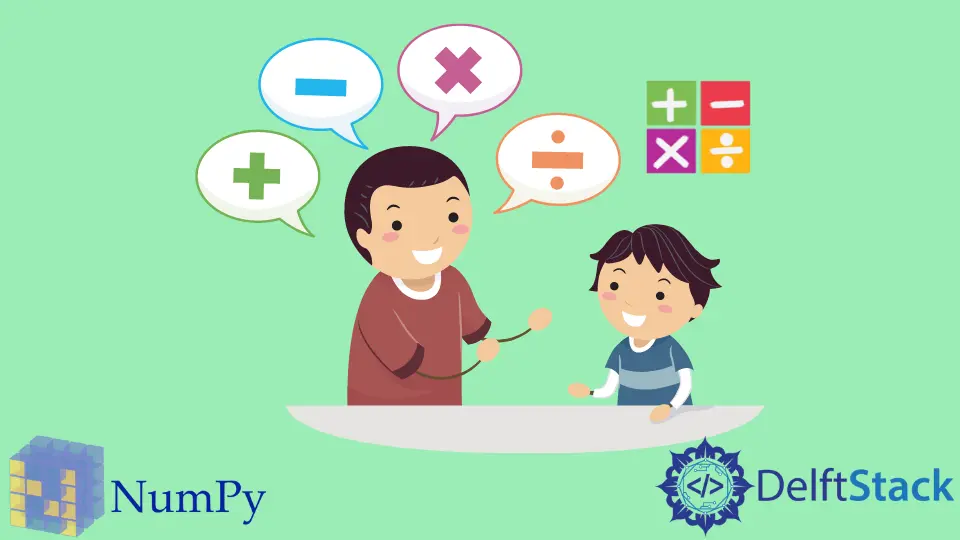
We’ll cover operations in NumPy in this chapter, like basic arithmetic operations and matrix operations.
Let’s start with basic arithmetic operation.
NumPy Arithmetic Operation
Addition, subtraction, multiplication and division are the most basic arithmetic operations in NumPy. They are similar to normal arithmetic operations between numbers.
import numpy as np
arrayA = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
arrayB = arrayA.T
# array([[1, 2, 3],
# [4, 5, 6],
# [7, 8, 9]])
arrayB = arrayA.T
# array([[1, 4, 7],
# [2, 5, 8],
# [3, 6, 9]])
arrayA + arrayB
# array([[ 2, 6, 10],
# [ 6, 10, 14],
# [10, 14, 18]])
arrayA - arrayB
# array([[ 0, -2, -4],
# [ 2, 0, -2],
# [ 4, 2, 0]])
arrayA * arrayB
# array([[ 1, 8, 21],
# [ 8, 25, 48],
# [21, 48, 81]])
arrayA / arrayB
# array([[1. , 0.5 , 0.42857143],
# [2. , 1. , 0.75 ],
# [2.33333333, 1.33333333, 1. ]])
It should be noted that the matrix multiplication operation - *
, multiplies the elements at the same position on the two arrays to get the element in the same position of the result array. It is not the dot product of two given arrays that should be calculated with np.dot
method.
np.dot(arrayA, arrayB)
# array([[ 14, 32, 50],
# [ 32, 77, 122],
# [ 50, 122, 194]])
NumPy Broadcasting
Two arrays should have the same shape in the array math operation. But NumPy introduces the concept of broadcasting to autofill the array if possible when two arrays don’t have the same shape.
Let me explain this concept with examples,
import numpy as np
arrayA = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
arrayA + 1
# array([[ 2, 3, 4],
# [ 5, 6, 7],
# [ 8, 9, 10]])
Here, 1
is added to all the elements of arrayA
, or in other words, 1
is broadcasted from the shape (1, 1)
to the same shape of arrayA
- (3, 3)
to make the array addition operation possible.
The actual operation is as below,
arrayA + np.array([[1, 1, 1], [1, 1, 1], [1, 1, 1]])
Broadcasting in NumPy could also be used in below scenarios,
Two Arrays Has the Same Length in One Dimension, and One Array Has the Length as 1 in the Other Dimension
Let’s start with one example,
arrayC = np.array([10, 11, 12])
arrayA + arrayC
# array([[11, 13, 15],
# [14, 16, 18],
# [17, 19, 21]])
The shape of arrayA
is (3, 3)
and the shape of arrayC
is (3, 1)
. It meets the criteria of array dimensions, and the data on the single row in arrayC
will be broadcasted to three rows to match the shape of arrayA
.
It is also applicable if two arrays has the same length of row.
arrayD = np.array([[10], [11], [12]])
# array([[10],
# [11],
# [12]])
arrayA + arrayD
# array([[11, 12, 13],
# [15, 16, 17],
# [19, 20, 21]])
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook