NumPy Tutorial - NumPy Array Append
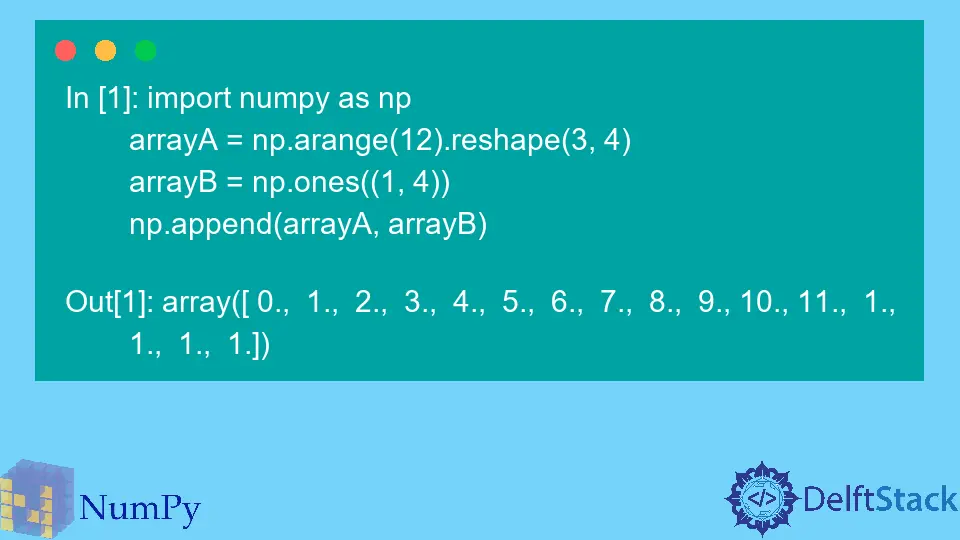
Numpy has also append
function to append data to array, just like append
operation to list
in Python. But in some cases, append
in NumPy is also a bit similar to extend
method in Python list
.
Array append
Let’s first list the syntax of ndarray.append
.
- Input Parameters
parameter name | type of data | Description |
---|---|---|
arr |
array_like | An array to add an element |
values |
array_like | Array added |
axis |
INT | The axis along which values are appended. |
Let’s make some examples,
In[1]: import numpy as np
arrayA = np.arange(12).reshape(3, 4)
arrayB = np.ones((1, 4))
np.append(arrayA, arrayB)
Out[1]: array([0., 1., 2., 3., 4., 5., 6., 7., 8., 9., 10., 11., 1.,
1., 1., 1.])
When axis
is not given, both arr
and values
are flattened before operation. The result will be a 1-D array. In the example above, we don’t need to care about the shape of two given arrays.
In[2]: np.append(arrayA, arrayB, axis=0)
Out[2]: array([[0., 1., 2., 3.],
[4., 5., 6., 7.],
[8., 9., 10., 11.],
[1., 1., 1., 1.]])
In[2]: np.append(arrayA, np.ones((1, 3)), axis=0)
---------------------------------------------------------------------------
ValueError Traceback(most recent call last)
<ipython-input-25-fe0fb14f5df8 > in < module > ()
--- -> 1 np.append(arrayA, np.ones((1, 3)), axis=0)
D: \ProgramData\Anaconda3\lib\site-packages\numpy\lib\function_base.py in append(arr, values, axis)
5164 values = ravel(values)
5165 axis = arr.ndim-1
-> 5166 return concatenate((arr, values), axis=axis)
ValueError: all the input array dimensions except for the concatenation axis must match exactly
When axis
is equal to 0
, array values
will be appended to arr
in the direction of column. It will raises the ValueError
if two given arrays don’t have the same length in the row - ValueError: all the input array dimensions except for the concatenation axis must match exactly
.
You could try by yourself to append the data in the row direction with the parameter axis=1
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook