Python Tutorial - Statement and Comment
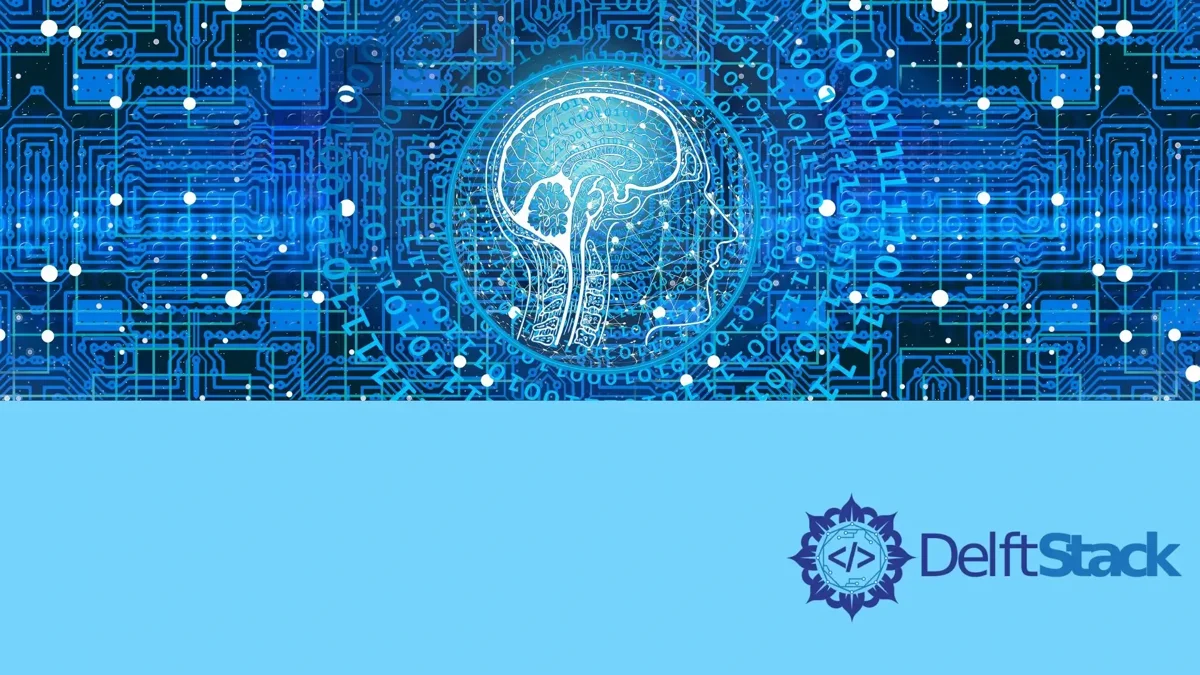
In this section, Python statements, indentation and comments will be discussed. Docstring
as a special type of Python comment is also introduced in the last session.
Python Statement
A Python statement is an instruction given to the interpreter to execute. A statement can contain an expression like below
result = x + y
Python statement can be regarded as an instruction to the interpreter to solve the expression and store its result in a variable. The statements such as for
, while
, print
have special meaning; these statements will be discussed in a later section.
Python Multi-Line Statements
When you press enter key after a statement, that particular statement is terminated and is a one-line statement. A multi-line statement can be created in Python by using line continuation character \
which will extend Python statement to multi-line.
Consider the code below:
x = 100 + 101 + 102 + 103 + 104 + 105
This is called explicit line continuation.
You can also do implicit line continuation by using parenthesis ()
, square brackets []
or curly braces {}
.
For example, you can write above multiple line statement using parenthesis as:
x = 100 + 101 + 102 + 103 + 104 + 105
Python Indentation
The block of statements, for example, the body of a function or a loop or class, starts with indentation. The indentation should be same for each statement inside the block. You will get an IndentationError
when indentation is incorrect.
Usually, 4 spaces for indentation as advised in Style Guide for Python Code. Consider the example below:
x = {1, 2, 3, 4}
for i in x:
print(i)
Indentation is basically used to create more readable programs.
In the example below, the same code is written in two different ways:
x = {1, 2, 3, 4}
for i in x:
print(i)
for i in x:
print(i)
You can see here that the first example has a better readability than the second one.
Python Comments
Comments are used to describe the purpose or working of a program. Comments are the lines ignored by Python during interpreting and they do not disturb the flow of a program.
If you are writing a code of hundreds of lines, you should add comments as other user does not have enough time to read every line to understand the working of the code. In this way, comments increase readability and explain how the code works as well.
A Python comment starts from hash #
symbol.
# Single Line comment
# Program to print a string
print("Hello Python Programmer")
Python Multi-Line Comments
Using hash symbol in each line can define a multi-line comment. But there is another way to add a multi-line comment in Python, that is using triple quotation marks. You can use either '''
or """
.
"""Multi-line
comments in
Python Programming language"""
Triple quotation marks are actually used to define multiline documentation string but you can also use them as multi-line comments.
Docstring
in Python
Docstring is the documentation string which is the first statement in a Python function, class, module, etc. The description and comments of functions, methods, and class are inside a docstring (documentation string).
Consider the example below:
def sum(a, b):
"""This function adds two values"""
return a + b
You can see here docstring tells what the function does.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook