Python Tutorial - Class
- Introduction to OOP
- Python Classes and Objects
- Python Class Constructors
- Delete Attributes of the Class
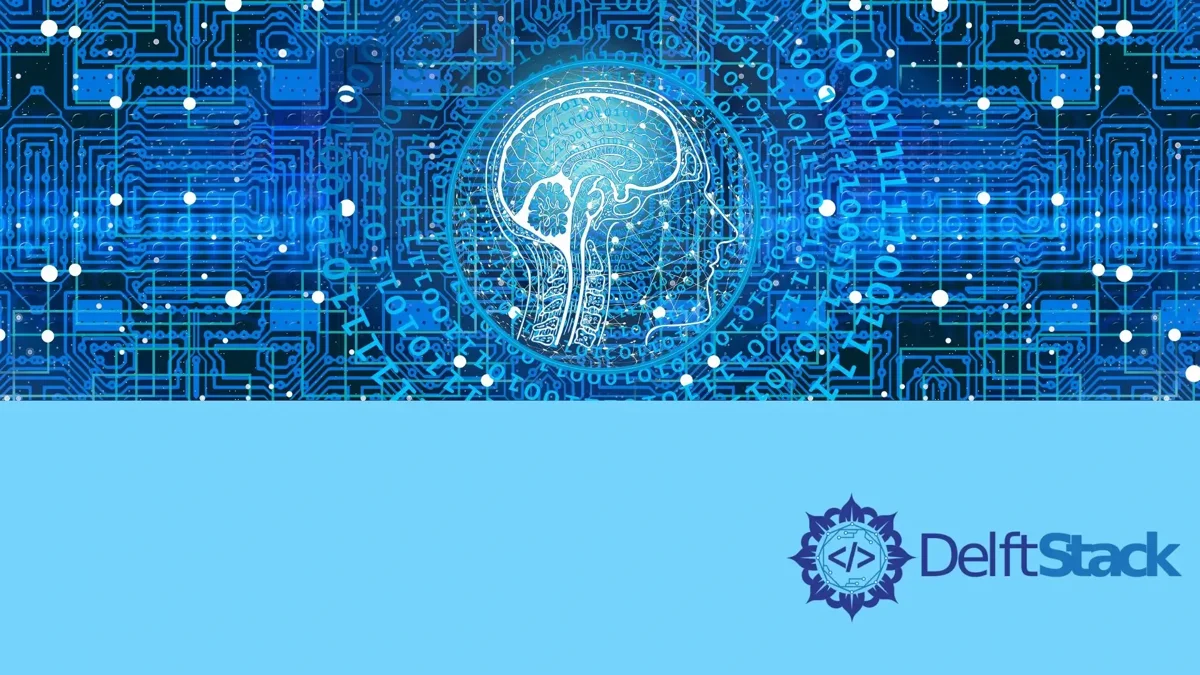
We will talk about the basics of Python object-oriented programming (OOP) like class, object in this section.
Python is an object-oriented programming language, in other words, everything in Python is an object.
Introduction to OOP
The basics of Object Oriented Programming (OOP) consists of the following terminologies:
- Classes
- Data members
- Methods
- Objects
- Constructors
- Method Overloading
- Operator Overloading
- Inheritance
- Class Instances and Instantiation
Python Classes and Objects
A class can be thought of as an outline for an object whereas, an object is a collection of data members and the member function.
A class contains data members (variables) and member functions (methods) that can be accessed by using the objects of that class.
Create a Class
A class is created by using the class
keyword and then the name of the class:
The syntax is as follows:
class NameofClass:
# data members of the class
# member functions of the class
Example:
>>> class Waiter:
name = "Andrew"
def display():
print('Waiter Class')
>>> print(Waiter.display)
<function Waiter.display at 0x0359E270>
>>> print(Waiter.name)
Andrew
This example creates a class named Waiter
that contains a data member name
and a member method display()
.
Create an Object Instance of a Class
An object instance can be created by using the following syntax:
obj = className()
Example:
>>> w = Waiter()
>>> print(w.name)
Andrew
>>> w.display()
Waiter Class
You could see here that the object of the Waiter
class is used to access the members (attributes) of the class by using the dot operator.
Class Attributes
Along with data members and member functions, there are some built-in attributes of a Python class.
__dict__
: is a dictionary that contains the namespace of the class.__doc__
: is the documentation string of the class__name__
: is the name of the class__module__
: is the module in which the class is defined.__bases__
: is a tuple that contains base class.
These attributes can be accessed by using the dot operator.
Example:
>>> class Waiter:
"This is a waiter class"
name="Andrew"
def display():
print("You are in waiter class")
>>> w = Waiter()
>>> print(w.__doc__)
This is a waiter class
>>> print(w.__dict__)
{}
>>> print(w.__module__)
__main__
Python Class Constructors
A constructor in Python OOP is created by using __init__()
. This built-in method is a special method called when an object of the corresponding class is created.
Constructors are used to initializing the data members of the class.
>>> class Waiter:
def __init__(self, n):
self.name=n
self.age=20
def display(self):
print(self.name)
>>> w=Waiter('Rob')
>>> w.display()
Rob
Here, self
argument is used in constructor definition and also all class methods. Therefore, when you call a function such as w.display()
, it is interpreted as Waiter.display(w)
.
Delete Attributes of the Class
You can use the del
statement to delete the attributes and objects of a class.
>>> w=Waiter('Rob')
>>> w.__dict__
{'name': 'Rob', 'age': 20}
>>> del w.age
>>> w.__dict__
{'name': 'Rob'}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook