파이썬 튜토리얼-클래스
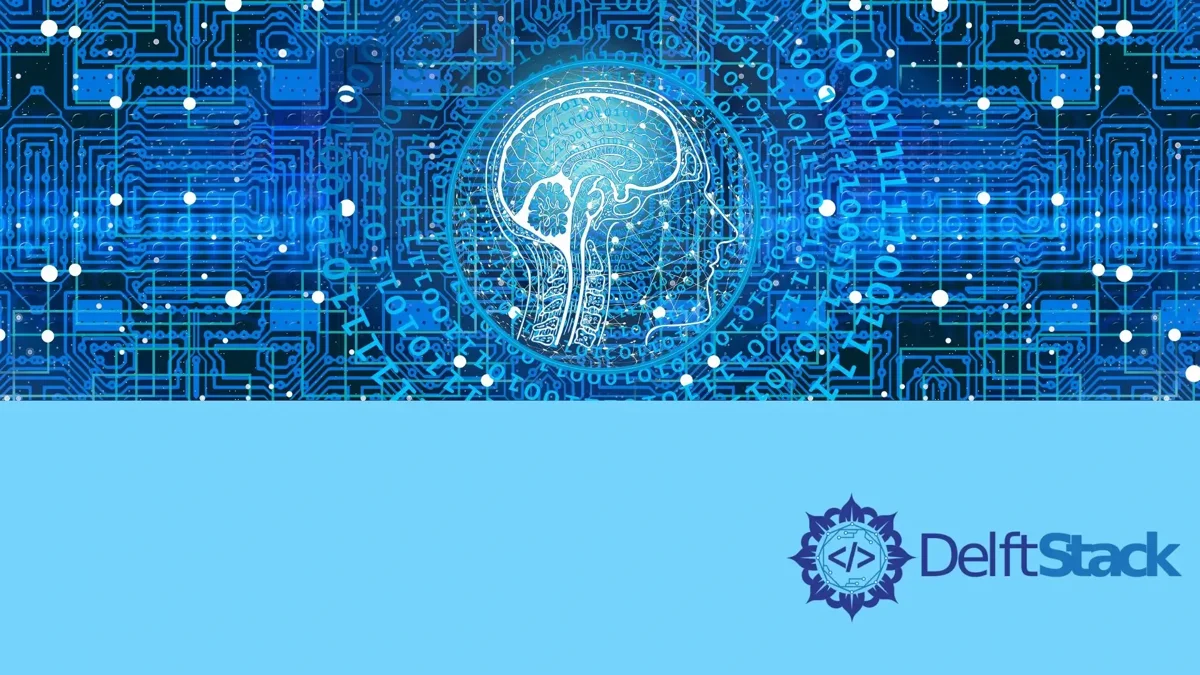
이 섹션에서는 클래스, 객체와 같은 Python 객체 지향 프로그래밍 (OOP)의 기본 사항에 대해 설명합니다.
파이썬은 객체 지향 프로그래밍 언어입니다. 즉, 파이썬의 모든 것은 객체입니다.
OOP 소개
객체 지향 프로그래밍 (OOP)의 기본 사항은 다음과 같은 용어로 구성됩니다.
- 수업
- 데이터 멤버
- 방법
- 객체
- 생성자
- 메소드 오버로딩
- 연산자 과부하
- 상속
- 클래스 인스턴스와 인스턴스화
파이썬 클래스와 객체
클래스는 객체의 개요로 생각할 수 있지만 객체는 데이터 멤버와 멤버 함수의 모음입니다.
클래스에는 해당 클래스의 객체를 사용하여 액세스 할 수있는 데이터 멤버 (변수) 및 멤버 함수 (방법)가 포함됩니다.
수업 만들기
클래스는 class
키워드와 클래스 이름을 사용하여 생성됩니다 :
구문은 다음과 같습니다.
class NameofClass:
# data members of the class
# member functions of the class
예:
>>> class Waiter:
name = "Andrew"
def display():
print('Waiter Class')
>>> print(Waiter.display)
<function Waiter.display at 0x0359E270>
>>> print(Waiter.name)
Andrew
이 예제는 데이터 멤버 name
과 멤버 메소드 display()
를 포함하는 Waiter
라는 클래스를 만듭니다.
클래스의 객체 인스턴스 만들기
다음 구문을 사용하여 객체 인스턴스를 만들 수 있습니다.
obj = className()
예:
>>> w = Waiter()
>>> print(w.name)
Andrew
>>> w.display()
Waiter Class
여기에서 Waiter
클래스의 객체가 도트 연산자를 사용하여 클래스의 멤버 (속성)에 액세스하는 데 사용된다는 것을 알 수 있습니다.
클래스 속성
데이터 멤버 및 멤버 함수와 함께 Python 클래스의 일부 내장 속성이 있습니다.
__dict__
: 클래스의 namespace를 포함하는 사전입니다.
__doc__
: 클래스의 문서 문자열입니다
__name__
: 클래스의 이름입니다
__module__
: 클래스가 정의 된 모듈입니다.
__bases__
: 기본 클래스를 포함하는 튜플입니다.
도트 연산자를 사용하여 이러한 속성에 액세스 할 수 있습니다.
예:
>>> class Waiter:
"This is a waiter class"
name="Andrew"
def display():
print("You are in waiter class")
>>> w = Waiter()
>>> print(w.__doc__)
This is a waiter class
>>> print(w.__dict__)
{}
>>> print(w.__module__)
__main__
파이썬 클래스 생성자
파이썬 OOP 의 생성자는__init __()
를 사용하여 생성됩니다. 이 내장 메소드는 해당 클래스의 오브젝트가 작성 될 때 호출되는 특수 메소드입니다.
생성자는 클래스의 데이터 멤버를 초기화하는 데 사용됩니다.
>>> class Waiter:
def __init__(self, n):
self.name=n
self.age=20
def display(self):
print(self.name)
>>> w=Waiter('Rob')
>>> w.display()
Rob
self
인수는 생성자 정의와 모든 클래스 메소드에서 사용됩니다. 따라서 w.display()
와 같은 함수를 호출하면 Waiter.display(w)
로 해석됩니다.
클래스 속성 삭제
del
문을 사용하여 클래스의 속성과 객체를 삭제할 수 있습니다.
>>> w=Waiter('Rob')
>>> w.__dict__
{'name': 'Rob', 'age': 20}
>>> del w.age
>>> w.__dict__
{'name': 'Rob'}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook