Python Tutorial - Data Type-Tuple
- Advantages of Tuple Over List
- Create a Tuple
- Access Tuple Elements
- Python Tuple Methods
- Tuple Built-In Functions:
- Tuple Membership Check
- Iterate Through a Tuple
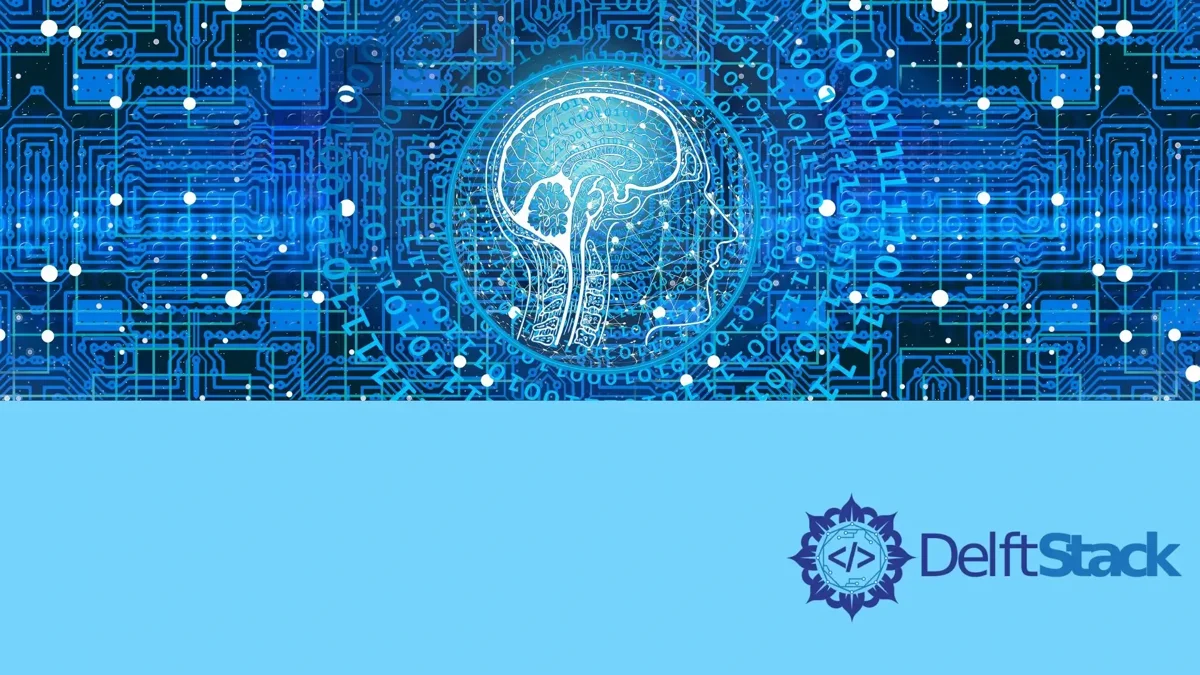
In this section, we will introduce how to create and use tuples.
A tuple is similar to a list with the difference that the tuple is immutable but the list is mutable.
Advantages of Tuple Over List
- Tuples are mostly used when the elements have different data types whereas, lists are used when the elements are of the same data type.
- Traversing through tuples is faster as you cannot update a tuple.
- You can use the immutable elements of the tuple as a key in the dictionary. This cannot be done with a list.
- Tuples guarantee the protection of the data as they are immutable.
Create a Tuple
You can define a Python tuple using parenthesis ()
and separate elements with commas ,
. A tuple can have elements of any data type.
>>> x = (3, 'pink', 3+8j)
>>> print('x[0] =', x[0])
x[0] = 3
>>> print('x[0:2] =', x[0:2])
x[0:2] = (3, 'pink')
>>> x[0] = 4
TypeError: 'tuple' object does not support item assignment
If the tuple contains only one element, for example, the element is of string data type, then the tuple will not be considered as a tuple rather it will be considered as a string. See the example below:
>>> x = ("Python")
>>> print(type(x))
<class 'str'>
You can see here that the data type of x
is str
rather than tuple
. To make it a tuple a trailing comma will be used as:
>>> x = "Python",
>>> print(type(x))
<class 'tuple'>
Access Tuple Elements
The elements of the tuple can be accessed by using any of the following ways:
Index
An index operator []
can be used to access elements of a tuple. The index of tuple starts from 0. If the index is not in range (item not defined at that index in the tuple), you will have an IndexError
. It should be noted here that index must be an integer otherwise TypeError
will occur.
>>> x = (3, 'pink', 3+8j)
>>> print(x[0])
3
>>> print(x[2])
(3+8j)
Negative Index
The same as Python list, you could use negative indexes to access tuple elements.
>>> l = (2, 4, 6, 8, 10)
>>> print(l[-1])
10
>>> print(l[-2])
8
>>> print(l[-3])
6
Slice
A slicing operator :
is used to extract a range of elements from a tuple.
>>> l = (2, 4, 6, 8, 10)
>>> print(l[1:3]) #prints from location 1 to 3
(4, 6)
>>> print(l[:2]) #prints from the beginning to location 2
(2, 4)
>>> print(l[2:]) #prints elements from location 2 onwards
(6, 8, 10)
>>> print(l[:]) #prints entire list
(2, 4, 6, 8, 10)
Concatenate Tuples
Two tuples can be concatenated by using +
operator.
>>> l = (2, 4, 6, 8, 10)
>>> print(l + (12, 14, 16)
(2, 4, 6, 8, 10, 12, 14, 16)
Delete a Tuple
The elements of a tuple cannot be deleted because tuples are immutable. But you can delete the entire tuple using the del
keyword:
l = (2, 4, 6, 8, 10)
del l
Python Tuple Methods
Tuples have only two methods as tuples are immutable:
Methods | Description |
---|---|
count(a) |
is used to return number of elements equal to a . |
index(a) |
is used to return index of the first element equal to a |
Tuple Built-In Functions:
Below are tuple built-in functions applicable to tuple
to perform different tasks:
Functions | Description |
---|---|
all() |
return True when all the elements of the tuple are True . It also returns True when the tuple is empty. |
any() |
return True when any of the element of the tuple is True . It returns False when the tuple is empty. |
enumerate() |
return the index and the value of all the elements of the tuple as a tuple. It returns an enumerate object. |
len() |
return the number of items in a tuple or the length of the tuple. |
tuple() |
convert a sequence (tuple, set, string, dictionary) to tuple. |
max() |
return the maximum number in the tuple. |
min() |
return the minimum number in the tuple. |
sorted() |
return a sorted tuple. |
sum() |
return the sum of all elements of the tuple. |
Tuple Membership Check
The in
keyword checks if an item is a member of the tuple or not. See the code exaple below:
>>> l = (2, 4, 6, 8, 10)
>>> print(5 in l)
False
>>> print(2 in l
True
Iterate Through a Tuple
You can iterate through a tuple by using for
loop:
l = (2, 4, 6, 8, 10)
for i in l:
print(i)
2
4
6
8
10
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook