Python Tutorial - Data Type-Array
- Python Array Declaration
- Python Array Index
- Array Negative Index
- Traverse Through a Python Array
- Python Array Update
- Different Ways to Modify a Python Array
- Delete Elements From a Python Array
- Array Methods
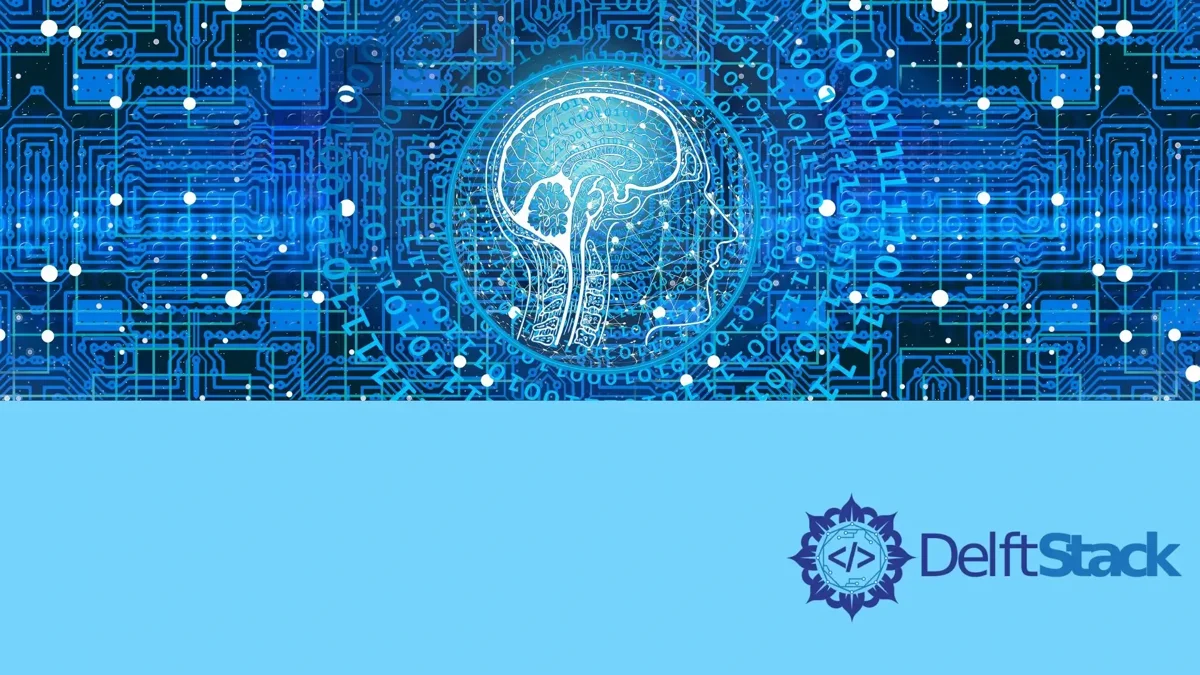
In this section, you will learn how to create and access elements of arrays in Python.
An array is a collection of elements of the same data type. The main difference between an array and a list is that a list can have elements of different data types.
In Python programming, to create an array, you need to import array module because an array is not a fundamental data type.
from array import *
Python Array Declaration
To declare an array you have to follow the syntax below:
arrayName = array(typecode, [initializers])
Here, arrayName
is the name of the array, typecode
determines the data type of the array and initializers
are the values in the array.
You will learn more about typecode
parameter later in this section.
Consider the example below:
a = array("i", [1, 2, 3, 4])
The name of the array is a
, typecode
is i
which specifies that values of the array will be of integer data type. If you try to store values other than the integers in this array, an error will be raised:
>>> a=array('i', [1,2,3.4,4])
Traceback (most recent call last):
File "<pyshell#4>", line 1, in <module>
a=array('i', [1,2,3.4,4])
TypeError: integer argument expected, got float
Let’s check the example of an array that contains some integers:
>>> from array import *
>>> a = array('i', [1,2,3,4])
>>> for i in a:
print(i)
1
2
3
4
typecode
Parameter
Parameter | Meaning |
---|---|
b |
a signed integer of 1 byte |
B |
an unsigned integer of 1 byte |
u |
a Unicode character of 2 bytes |
h |
a signed integer of 2 bytes |
H |
an unsigned integer of 2 bytes |
i |
a signed integer of 2 bytes |
I |
an unsigned integer of 2 bytes |
l |
a signed integer of 4 bytes |
L |
an unsigned integer of 4 bytes |
f |
a floating point number of 4 bytes |
d |
a floating point number of 8 bytes |
Python Array Index
You can fetch an element of an array using the index operator []
.
Retrieve an Element of the Array Using Index Operator
>>> a = array('i', [1,2,3,4])
>>> print("First element of array =", a[0])
First element of array = 1
>>> print("Second element of array =", a[1])
Second element of array = 2
Get the Index of Given Value by Using Index(x)
Method
The index(x)
method returns the smallest index of the first occurrence of x
.
>>> a = array('i', [1,2,3,4])
>>> print("1 is found at location:", a.index(1))
1 is found at location: 0
>>> print("4 is found at location:", a.index(4))
4 is found at location: 3
Array Negative Index
Similar to other Python sequential data types like strings
, lists
, tuples
, range
objects, you could also use negative indexes to access elements in the array.
>>> a = array('i', [1,2,3,4])
>>> print("last element of array:",a[-1])
last element of array: 4
Traverse Through a Python Array
First thing first, finding the length of the array:
Array Len()
Method
The len()
method returns the number of elements in an array.
>>> a = array('i', [1,2,3,4])
>>> print("length of a:", len(a))
length of a: 4
Iterate Over an Array
You can use for
loop to iterate through elements of an array.
>>> a = array('i', [1,2,3,4])
>>> for i in range(len(a)):
print(a[i])
1
2
3
4
or
>>> a = array('i', [1,2,3,4])
>>> for i in a:
print(i)
1
2
3
4
Python Array Update
Append()
You can use the array method append()
to append an item to the end of an array.
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> a.append(10)
>>> print(a)
array('i', [1, 2, 3, 4, 5, 7, 8, 9, 10])
Insert(i, X)
You could use insert(i, x)
method to insert the element x
to the given index i
.
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> a.insert(3,44)
>>> print(a)
array('i', [1, 2, 3, 44, 4, 5, 7, 8, 9])
Extend(x)
append(x)
adds the item x
as one item to the array. extend(x)
method is the right method to extend the array by appending all the elements in the given object x
.
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> a.extend([10,11,12,13,14,15])
>>> print(a)
array('i', [1, 2, 3, 4, 5, 7, 8, 9, 10, 11, 12, 13, 14, 15])
x
is another array, it shall have exactly the same data type code, otherwise, TypeError
will be raised.If
x
is not a Python array, it must be iterable and its elements must have the same data type with the array to be extended.Fromlist()
fromlist()
adds items from a list into the array.
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> l = [10,11]
>>> a.fromlist(l)
>>> print(a)
array('i', [1, 2, 3, 4, 5, 7, 8, 9, 10, 11])
Different Ways to Modify a Python Array
Reverse()
reverse()
reverses the array.
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> a.reverse()
>>> print(a)
array('i', [9, 8, 7, 5, 4, 3, 2, 1])
+
Operator
Two arrays can be concatenated by using +
operator:
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> b = array('i', [10,11])
>>> print(a + b)
array('i', [1, 2, 3, 4, 5, 7, 8, 9, 10, 11])
*
Operator
*
operator repeats elements in the array a specific number of times.
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> a = a * 2
>>> print(a)
array('i', [1, 2, 3, 4, 5, 7, 8, 9, 1, 2, 3, 4, 5, 7, 8, 9])
Here the elements are repeated twice.
Delete Elements From a Python Array
The del
statement deletes one or more items from an array. You can also use del
to delete the entire array:
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> del a[3]
>>> print(a)
array('i', [1, 2, 3, 5, 7, 8, 9])
>>> del a #entire array is deleted
Remove(x)
remove()
removes the first occurrence of x
from an array:
>>> a = array('i', [1,2,3,4,5,7,8,9,3])
>>> a.remove(3)
>>> print(a)
array('i', [1, 2, 4, 5, 7, 8, 9, 3])
Pop()
pop()
will remove and return the last element from the array.
>>> a = array('i', [1,2,3,4,5,7,8,9])
>>> a.pop()
9
>>> print(a)
array('i', [1, 2, 3, 4, 5, 7, 8])
Array Methods
The following table describes the array methods,:
Methods | Description |
---|---|
append() |
add an item to the end of the array |
extend() |
add more than one items to an array. It works by adding elements of an array to another array (in which you want to add elements). |
insert() |
add an element at the desired location. |
remove() |
remove a particular item from the array. |
pop() |
remove an item from a given location and returns that item. |
clear() |
remove all elements from the array. |
index() |
return the index of the first matched element in the array. |
count() |
return the total number of items passed |
sort() |
sort elements of the array in ascending order. |
reverse() |
reverse the order of the elements of the array. |
copy() |
return a copy of an already existing array. |
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook