PyQt5 Tutorial - Label
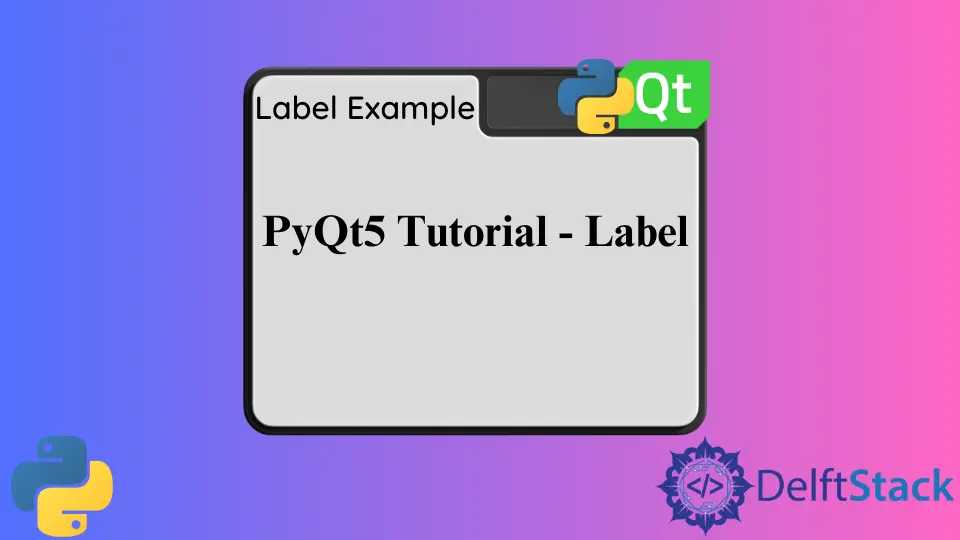
We will learn to use PyQt5 label widget QLabel
in this tutorial.
PyQt5 Label Widget
We’re going to add two labels to our window, where one of those labels is going to hold some text and one of those labels is going to hold an image.
import sys
from PyQt5 import QtWidgets, QtGui
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
labelA = QtWidgets.QLabel(windowExample)
labelB = QtWidgets.QLabel(windowExample)
labelA.setText("Label Example")
labelB.setPixmap(QtGui.QPixmap("python.jpg"))
windowExample.setWindowTitle("Label Example")
windowExample.setGeometry(100, 100, 300, 200)
labelA.move(100, 40)
labelB.move(120, 120)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
Where,
labelA = QtWidgets.QLabel(w)
The first label labelA
is a QtWidgets.QtLabel
and the QtWidgets
- w
is in parentheses because it tells the program that the label labelA
is added to the window w
.
labelA.setText("Label Example")
labelA.setText
sets the text in the label.
windowExample.setGeometry(100, 100, 300, 200)
It sets the window size to be (300, 200)
and left upper corner coordinate to be (100, 100)
. You could refer to the setGeometry
explanation in last section.
labelA.move(100, 40)
labelB.move(120, 120)
move()
method moves the label to the direction of right and down. Like labelA.move(100, 40)
moves the labelA
to the coordinate of (100, 40)
relative to the left-upper corner of the window.
labelB.setPixmap(QtGui.QPixmap("globe.png"))
It displays images in the labelB
. QPixmap
is the module in QtGui
and it takes an image of Qt
.
PyQt5 QLabel
Set Font
In the example above, we use the default font in label widget, and you could specify the preferred font like size, weight and font family of the label text.
import sys
from PyQt5 import QtWidgets, QtGui
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
labelA = QtWidgets.QLabel(windowExample)
labelB = QtWidgets.QLabel(windowExample)
labelA.setText("Times Font")
labelA.setFont(QtGui.QFont("Times", 12, QtGui.QFont.Bold))
labelB.setText("Arial Font")
labelB.setFont(QtGui.QFont("Arial", 14, QtGui.QFont.Black))
windowExample.setWindowTitle("Label Example")
windowExample.setGeometry(100, 100, 300, 200)
labelA.move(100, 40)
labelB.move(100, 120)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
labelA.setFont(QtGui.QFont("Times", 12, QtGui.QFont.Bold))
setFont()
method sets the font of the label. The QFont
class specifies a font with specific attributes.
PyQt5 Label Alignment
The label text is by default aligned to the left edge, and this property could be modified with the method QLabel.setAlignment()
.
import sys
from PyQt5 import QtWidgets, QtGui, QtCore
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
labelLeft = QtWidgets.QLabel(windowExample)
labelRight = QtWidgets.QLabel(windowExample)
labelCenter = QtWidgets.QLabel(windowExample)
labelLeft.setText("Left Align")
labelRight.setText("Right Align")
labelCenter.setText("Center Align")
windowExample.setWindowTitle("Label Align Example")
windowExample.setGeometry(100, 100, 300, 200)
labelLeft.setFixedWidth(160)
labelRight.setFixedWidth(160)
labelCenter.setFixedWidth(160)
labelLeft.setStyleSheet("border-radius: 25px;border: 1px solid black;")
labelRight.setStyleSheet("border-radius: 25px;border: 1px solid black;")
labelCenter.setStyleSheet("border-radius: 25px;border: 1px solid black;")
labelLeft.setAlignment(QtCore.Qt.AlignLeft)
labelRight.setAlignment(QtCore.Qt.AlignRight)
labelCenter.setAlignment(QtCore.Qt.AlignCenter)
labelLeft.move(80, 40)
labelRight.move(80, 80)
labelCenter.move(80, 120)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
labelLeft.setFixedWidth(160)
labelRight.setFixedWidth(160)
labelCenter.setFixedWidth(160)
It sets the fixed width to the three labels, otherwise, the label width is automatically set according to the label text length.
labelLeft.setStyleSheet("border-radius: 25px;border: 1px solid black;")
We could use CSS-alike style sheet to set the styles of the PyQt5 widgets. Here, the border of the label is set to be solid black with the border with of 1px, and the border radius is specified as 25px.
labelLeft.setAlignment(QtCore.Qt.AlignLeft)
labelRight.setAlignment(QtCore.Qt.AlignRight)
labelCenter.setAlignment(QtCore.Qt.AlignCenter)
The alignment property of the widget is set by the method setAlignment
and its options are in the module PyQt5.QtCore.Qt
, like
PyQt5.QtCore.Qt.AlignLeft
PyQt5.QtCore.Qt.AlignRight
PyQt5.QtCore.Qt.AlignCenter
As you could see from the image below, the labels are aligned according to their alignment properties.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook