C#에서 목록 섞기
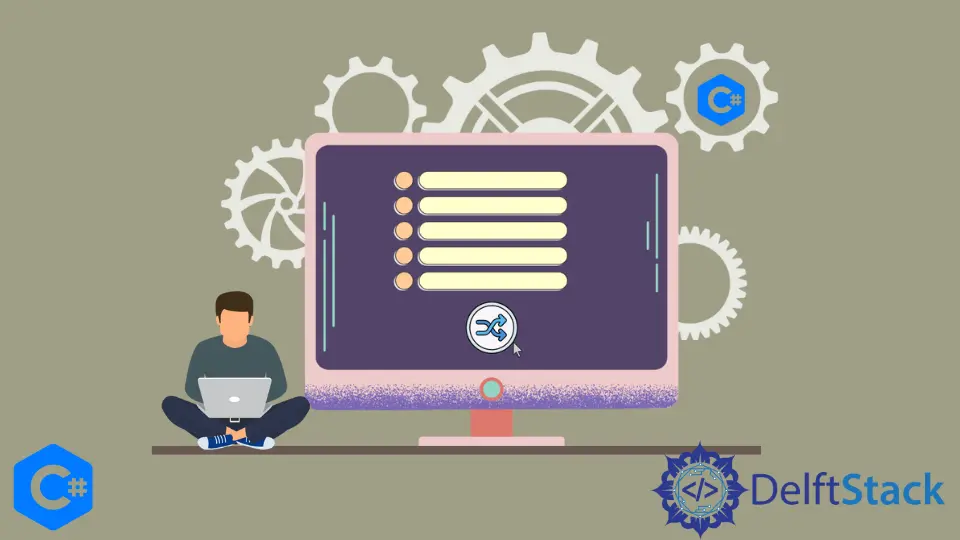
이 자습서에서는 C#에서 List를 섞는 방법에 대해 설명합니다.
C#에서 Linq로 목록 섞기
언어 통합 쿼리 또는 Linq
는 C#에서 쿼리 기능을 통합하는 방법을 제공합니다. Linq는 C#의 SQL과 같은 기능을 제공합니다. Linq를 사용하여 목록을 무작위화할 수 있습니다. 다음 코드 예제는 C#에서 Linq로 목록을 섞는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace shuffle_list {
class Program {
static void Main(string[] args) {
List<int> list1 = new List<int>() { 1, 2, 3, 4, 5 };
var rnd = new Random();
var randomized = list1.OrderBy(item => rnd.Next());
foreach (var value in randomized) {
Console.WriteLine(value);
}
}
}
}
출력:
1
4
5
3
2
먼저list1
목록을 초기화 한 다음random.next()
함수와 C#의 Linq OrderBy()
함수를 사용하여list1
목록을 섞었습니다. 위의 방법을 사용하여 C#에서 개체 목록을 섞을 수도 있습니다. 다음 코드 예제는 C#에서 Linq를 사용하여 개체 목록을 섞는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace shuffle_list {
public class Person {
string name;
public Person(string name) {
Name = name;
}
public string Name {
get => name;
set => name = value;
}
}
class Program {
static void Main(string[] args) {
List<Person> list1 = new List<Person>();
list1.Add(new Person("Person 1"));
list1.Add(new Person("Person 2"));
list1.Add(new Person("Person 3"));
list1.Add(new Person("Person 4"));
list1.Add(new Person("Person 5"));
var rnd = new Random();
var randomized = list1.OrderBy(item => rnd.Next());
foreach (var value in randomized) {
Console.WriteLine(value.Name);
}
}
}
}
출력:
Person 5
Person 2
Person 1
Person 3
Person 4
Person
클래스의 객체 목록을 C#의 Linq와 섞었습니다.
Fisher-Yates Shuffle 알고리즘을 사용하여 목록 섞기
Fisher-Yates 셔플 알고리즘은 C#에서 유한 데이터 구조를 셔플합니다. Fisher-Yates 셔플 알고리즘은 C#에서 편향되지 않은 셔플 링을 제공합니다. 목록의 각 요소를 목록 내의 임의 색인에 순차적으로 저장합니다. 다음 코딩 예제는 C#에서 Fisher-Yates 셔플 알고리즘을 사용하여 목록을 셔플하는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace shuffle_list {
static class ExtensionsClass {
private static Random rng = new Random();
public static void Shuffle<T>(this IList<T> list) {
int n = list.Count;
while (n > 1) {
n--;
int k = rng.Next(n + 1);
T value = list[k];
list[k] = list[n];
list[n] = value;
}
}
}
class Program {
static void Main(string[] args) {
List<int> list1 = new List<int>() { 1, 2, 3, 4, 5 };
list1.Shuffle();
foreach (var value in list1) {
Console.WriteLine(value);
}
}
}
}
출력:
5
1
4
3
2
C#의 Fisher-Yates 셔플 알고리즘을 사용하여 정수 목록list1
을 섞었습니다. C#에서 Fisher-Yates 알고리즘을 구현하기위한 확장 메서드를 만들었습니다. 확장 메서드를 생성하려면ExtensionClass
라는 다른static
클래스에서Shuffle()
함수를 정의해야했습니다. 아래 코드에서 설명하는 것처럼 동일한 알고리즘을 사용하여 객체 목록을 섞을 수도 있습니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace shuffle_list {
public class Person {
string name;
public Person(string name) {
Name = name;
}
public string Name {
get => name;
set => name = value;
}
}
static class ExtensionsClass {
private static Random rng = new Random();
public static void Shuffle<T>(this IList<T> list) {
int n = list.Count;
while (n > 1) {
n--;
int k = rng.Next(n + 1);
T value = list[k];
list[k] = list[n];
list[n] = value;
}
}
}
class Program {
static void Main(string[] args) {
List<Person> list1 = new List<Person>();
list1.Add(new Person("Person 1"));
list1.Add(new Person("Person 2"));
list1.Add(new Person("Person 3"));
list1.Add(new Person("Person 4"));
list1.Add(new Person("Person 5"));
list1.Shuffle();
foreach (var value in list1) {
Console.WriteLine(value.Name);
}
}
}
}
출력:
Person 1
Person 4
Person 2
Person 5
Person 3
C#에서 Fisher-Yates 알고리즘을 사용하여Person
클래스의 객체 목록을 섞었습니다. C#에서 Fisher-Yates 알고리즘을 구현하기위한 확장 메서드를 만들었습니다. 확장 메서드를 생성하려면ExtensionClass
라는 다른static
클래스에서Shuffle()
함수를 정의해야했습니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn