Embaralhar uma lista em C#
-
Misture uma lista com Linq em
C#
-
Embaralhe uma lista com o algoritmo de embaralhamento de Fisher-Yates em
C#
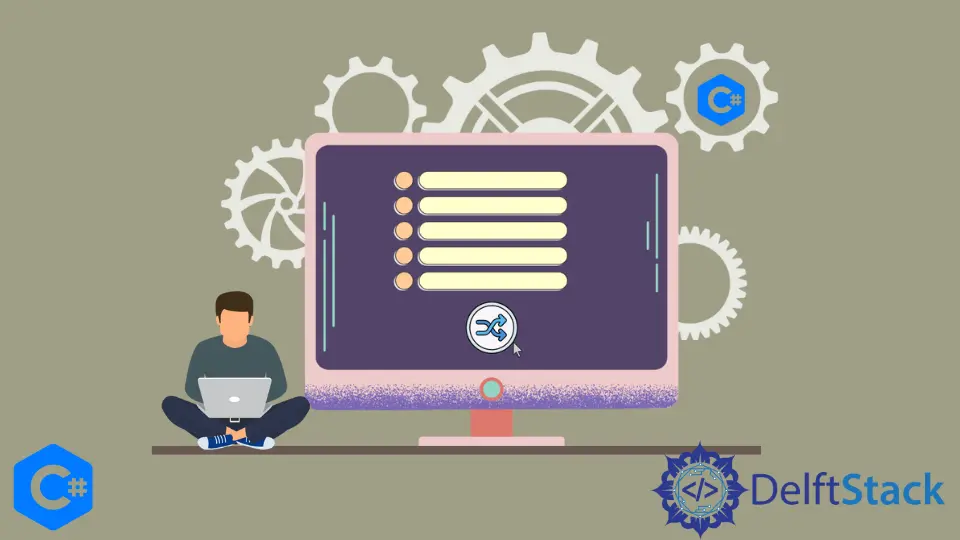
Neste tutorial, discutiremos métodos para embaralhar uma lista em C#.
Misture uma lista com Linq em C#
A consulta integrada de linguagem ou Linq
fornece uma maneira de integrar a capacidade de consultas em C#. O Linq fornece funcionalidade exatamente como SQL em C#. Podemos usar o Linq para randomizar uma lista. O exemplo de código a seguir nos mostra como podemos embaralhar uma lista com Linq em C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace shuffle_list {
class Program {
static void Main(string[] args) {
List<int> list1 = new List<int>() { 1, 2, 3, 4, 5 };
var rnd = new Random();
var randomized = list1.OrderBy(item => rnd.Next());
foreach (var value in randomized) {
Console.WriteLine(value);
}
}
}
}
Resultado:
1 4 5 3 2
Inicialmente inicializamos a lista list1
e depois embaralhamos a lista list1
com a função random.next()
e a função OrderBy()
do Linq em C#. O método acima também pode ser usado para embaralhar uma lista de objetos em C#. O exemplo de código a seguir nos mostra como embaralhar uma lista de objetos com Linq em C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace shuffle_list {
public class Person {
string name;
public Person(string name) {
Name = name;
}
public string Name {
get => name;
set => name = value;
}
}
class Program {
static void Main(string[] args) {
List<Person> list1 = new List<Person>();
list1.Add(new Person("Person 1"));
list1.Add(new Person("Person 2"));
list1.Add(new Person("Person 3"));
list1.Add(new Person("Person 4"));
list1.Add(new Person("Person 5"));
var rnd = new Random();
var randomized = list1.OrderBy(item => rnd.Next());
foreach (var value in randomized) {
Console.WriteLine(value.Name);
}
}
}
}
Resultado:
Person 5 Person 2 Person 1 Person 3 Person 4
Misturamos uma lista de objetos da classe Person
com o Linq em C#.
Embaralhe uma lista com o algoritmo de embaralhamento de Fisher-Yates em C#
O algoritmo de embaralhamento de Fisher-Yates embaralha uma estrutura de dados finita em C#. O algoritmo de embaralhamento Fisher-Yates fornece embaralhamento imparcial em C#. Ele armazena sequencialmente cada elemento da lista em um índice aleatório dentro da lista. O exemplo de codificação a seguir nos mostra como embaralhar uma lista com o algoritmo de embaralhamento de Fisher-Yates em C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace shuffle_list {
static class ExtensionsClass {
private static Random rng = new Random();
public static void Shuffle<T>(this IList<T> list) {
int n = list.Count;
while (n > 1) {
n--;
int k = rng.Next(n + 1);
T value = list[k];
list[k] = list[n];
list[n] = value;
}
}
}
class Program {
static void Main(string[] args) {
List<int> list1 = new List<int>() { 1, 2, 3, 4, 5 };
list1.Shuffle();
foreach (var value in list1) {
Console.WriteLine(value);
}
}
}
}
Resultado:
5 1 4 3 2
Misturamos uma lista de inteiros list1
com o algoritmo de embaralhamento Fisher-Yates em C#. Criamos um método de extensão para implementar o algoritmo Fisher-Yates em C#. Para criar um método de extensão, tivemos que definir a função Shuffle()
em outra classe static
chamada ExtensionClass
. O mesmo algoritmo também pode ser usado para embaralhar uma lista de objetos, conforme demonstrado nos códigos a seguir.
using System;
using System.Collections.Generic;
using System.Linq;
namespace shuffle_list {
public class Person {
string name;
public Person(string name) {
Name = name;
}
public string Name {
get => name;
set => name = value;
}
}
static class ExtensionsClass {
private static Random rng = new Random();
public static void Shuffle<T>(this IList<T> list) {
int n = list.Count;
while (n > 1) {
n--;
int k = rng.Next(n + 1);
T value = list[k];
list[k] = list[n];
list[n] = value;
}
}
}
class Program {
static void Main(string[] args) {
List<Person> list1 = new List<Person>();
list1.Add(new Person("Person 1"));
list1.Add(new Person("Person 2"));
list1.Add(new Person("Person 3"));
list1.Add(new Person("Person 4"));
list1.Add(new Person("Person 5"));
list1.Shuffle();
foreach (var value in list1) {
Console.WriteLine(value.Name);
}
}
}
}
Resultado:
Person 1 Person 4 Person 2 Person 5 Person 3
Misturamos uma lista de objetos da classe Person
com o algoritmo Fisher-Yates em C#. Criamos um método de extensão para implementar o algoritmo Fisher-Yates em C#. Para criar um método de extensão, tivemos que definir a função Shuffle()
em outra classe static
chamada ExtensionClass
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn