C#의 LINQ Group by
Muhammad Maisam Abbas
2024년2월16일
Csharp
Csharp LINQ
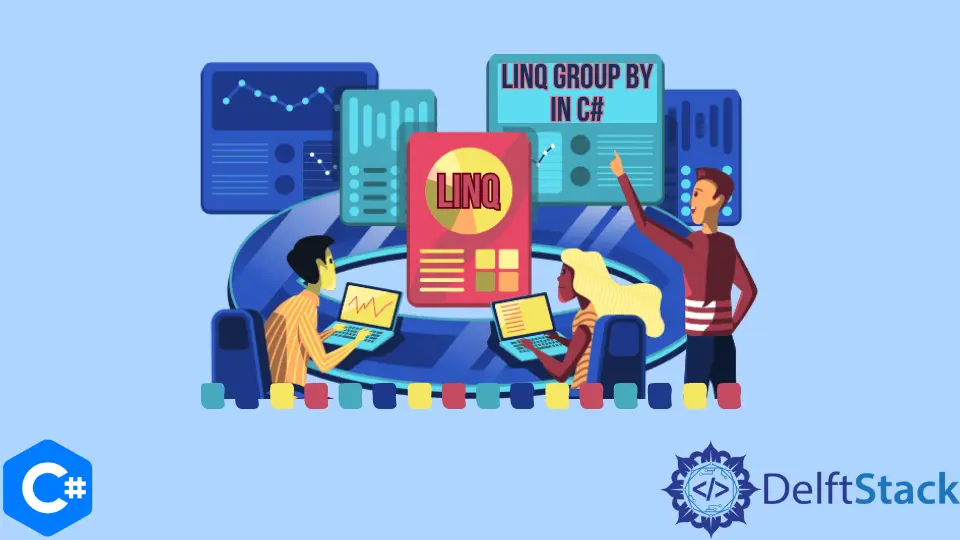
이 자습서에서는 C#의 값을 기준으로 개체 목록을 그룹화하는 방법에 대해 설명합니다.
C#의 LINQ Group by
LINQ는 SQL과 유사한 쿼리 기능을 C#의 데이터 구조와 통합합니다.
다음 클래스의 객체 목록이 있다고 가정합니다.
class Car {
public string Brand { get; set; }
public int Model { get; set; }
}
Brand
는 자동차 브랜드의 이름이고 Model
은 자동차의 모델 번호입니다. 여러 개체의 Brand
속성은 동일 할 수 있지만 Model
번호는 개체마다 달라야합니다. 브랜드 이름별로 개체 목록을 그룹화하려면 LINQ에서 GroupBy
메서드를 사용할 수 있습니다. 다음 코드 예제는 LINQ의GroupBy
메서드를 사용하여 특정 클래스의 개체를 값별로 그룹화하는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace linq_goup_by {
public class Car {
public string Brand { get; set; }
public int Model { get; set; }
public Car(string b, int m) {
Brand = b;
Model = m;
}
}
class Program {
static void Main(string[] args) {
List<Car> cars = new List<Car>();
cars.Add(new Car("Brand 1", 11));
cars.Add(new Car("Brand 1", 22));
cars.Add(new Car("Brand 2", 12));
cars.Add(new Car("Brand 2", 21));
var results = from c in cars group c by c.Brand;
foreach (var r in results) {
Console.WriteLine(r.Key);
foreach (Car c in r) {
Console.WriteLine(c.Model);
}
}
}
}
}
출력:
Brand 1
11
22
Brand 2
12
21
위의 코드에서 먼저cars
오브젝트 목록을 선언하고 초기화 한 다음Brand
속성으로 값을 그룹화하고results
변수에 저장합니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn