C# 中的 LINQ 分组
Muhammad Maisam Abbas
2023年10月12日
Csharp
Csharp LINQ
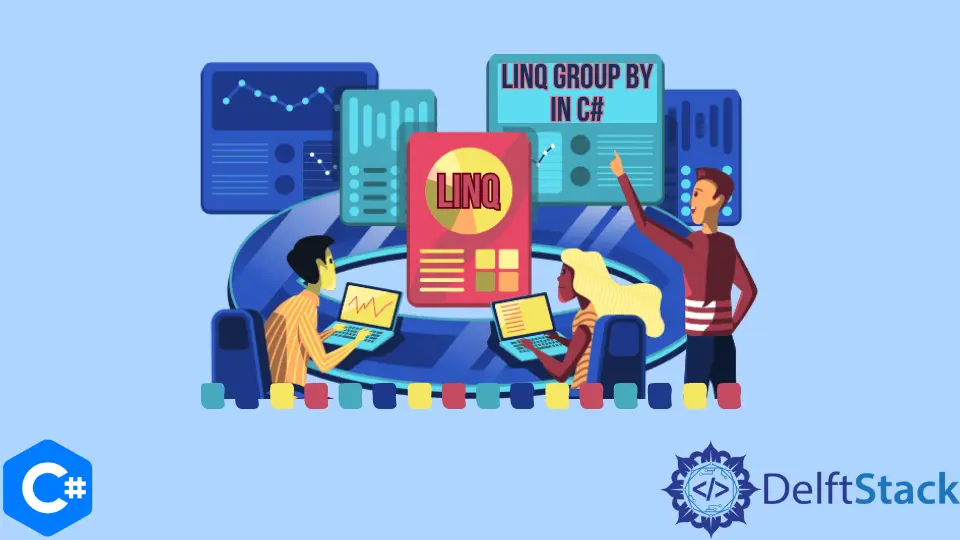
本教程将讨论在 C# 中按值对对象列表进行分组的方法。
C# 中的 LINQ 分组
LINQ 将类似 SQL 的查询功能与 C# 中的数据结构集成在一起。
假设我们有以下类别的对象的列表。
class Car {
public string Brand { get; set; }
public int Model { get; set; }
}
Brand
是汽车的品牌名称,Model
是汽车的型号。多个对象的 Brand
属性可以相同,但是每个对象的 Model
编号必须不同。如果要按品牌名称对对象列表进行分组,可以在 LINQ 中使用 GroupBy
方法。以下代码示例向我们展示了如何使用 LINQ 中的 GroupBy
方法按某个值对特定类的对象进行分组。
using System;
using System.Collections.Generic;
using System.Linq;
namespace linq_goup_by {
public class Car {
public string Brand { get; set; }
public int Model { get; set; }
public Car(string b, int m) {
Brand = b;
Model = m;
}
}
class Program {
static void Main(string[] args) {
List<Car> cars = new List<Car>();
cars.Add(new Car("Brand 1", 11));
cars.Add(new Car("Brand 1", 22));
cars.Add(new Car("Brand 2", 12));
cars.Add(new Car("Brand 2", 21));
var results = from c in cars group c by c.Brand;
foreach (var r in results) {
Console.WriteLine(r.Key);
foreach (Car c in r) {
Console.WriteLine(c.Model);
}
}
}
}
}
输出:
Brand 1
11
22
Brand 2
12
21
在上面的代码中,我们首先声明并初始化了对象 cars
的列表,然后通过 Brand
属性对值进行分组并将其保存在 result
变量中。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn