C# 中的 LINQ 分組
Muhammad Maisam Abbas
2024年2月16日
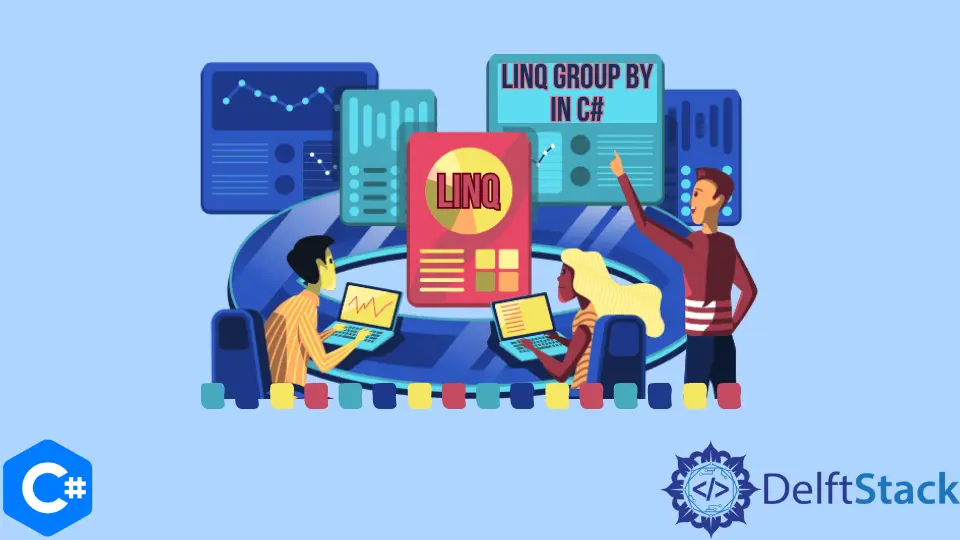
本教程將討論在 C# 中按值對物件列表進行分組的方法。
C# 中的 LINQ 分組
LINQ 將類似 SQL 的查詢功能與 C# 中的資料結構整合在一起。
假設我們有以下類別的物件的列表。
class Car {
public string Brand { get; set; }
public int Model { get; set; }
}
Brand
是汽車的品牌名稱,Model
是汽車的型號。多個物件的 Brand
屬性可以相同,但是每個物件的 Model
編號必須不同。如果要按品牌名稱對物件列表進行分組,可以在 LINQ 中使用 GroupBy
方法。以下程式碼示例向我們展示瞭如何使用 LINQ 中的 GroupBy
方法按某個值對特定類的物件進行分組。
using System;
using System.Collections.Generic;
using System.Linq;
namespace linq_goup_by {
public class Car {
public string Brand { get; set; }
public int Model { get; set; }
public Car(string b, int m) {
Brand = b;
Model = m;
}
}
class Program {
static void Main(string[] args) {
List<Car> cars = new List<Car>();
cars.Add(new Car("Brand 1", 11));
cars.Add(new Car("Brand 1", 22));
cars.Add(new Car("Brand 2", 12));
cars.Add(new Car("Brand 2", 21));
var results = from c in cars group c by c.Brand;
foreach (var r in results) {
Console.WriteLine(r.Key);
foreach (Car c in r) {
Console.WriteLine(c.Model);
}
}
}
}
}
輸出:
Brand 1
11
22
Brand 2
12
21
在上面的程式碼中,我們首先宣告並初始化了物件 cars
的列表,然後通過 Brand
屬性對值進行分組並將其儲存在 result
變數中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn