C++에서 문자열의 모든 순열 인쇄
-
std::next_permutation
을 사용하여 C++에서 문자열의 모든 순열을 인쇄합니다 -
std::prev_permutation
을 사용하여 C++에서 문자열의 모든 순열을 인쇄합니다
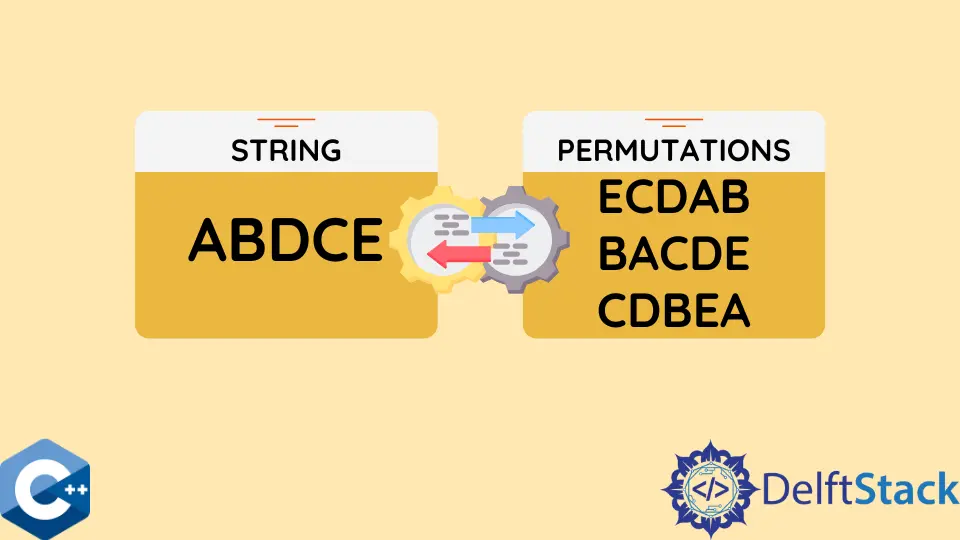
이 기사에서는 C++에서 주어진 문자열의 모든 순열을 인쇄하는 방법을 소개합니다.
std::next_permutation
을 사용하여 C++에서 문자열의 모든 순열을 인쇄합니다
std:next_permutation
알고리즘은 요소의 순열이 사전 순으로 오름차순으로 정렬되도록 지정된 범위를 수정하고 그러한 순열이 존재하면 참 부울 값이 반환됩니다. 문자열 문자가 내림차순으로 정렬 된 경우 함수는std::string
객체에서 작동하여 순열을 생성 할 수 있습니다. std::sort
알고리즘을std::greater
함수 객체와 함께 사용하여 문자열을 정렬 한 다음 false를 반환 할 때까지next_permutation
을 호출 할 수 있습니다. 후자는next_permutation
문을 조건 표현식으로 사용하고 매 사이클마다cout
스트림에 문자열을 인쇄하는do...while
루프를 사용하여 구현할 수 있습니다.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::next_permutation(str.begin(), str.end()));
}
int main() {
string input;
cout << "Enter string to print permutations: ";
cin >> input;
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
출력:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
std::prev_permutation
을 사용하여 C++에서 문자열의 모든 순열을 인쇄합니다
또는 STL의 다른 알고리즘 인std::prev_permutation
을 사용할 수 있습니다.이 알고리즘은 동일한 사전 순서로 주어진 범위의 새 순열을 생성하지만 시퀀스가 제공 될 때 이전 순열을 저장합니다. 최종 솔루션은prev_permutation
함수가while
루프 조건에서 호출된다는 점을 제외하고 이전 예제와 유사합니다.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::prev_permutation(str.begin(), str.end()));
}
int main() {
string input;
cout << "Enter string to print permutations: ";
cin >> input;
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
출력:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
또한 사용자가 유효한 문자열을 제공 할 때까지 입력 프롬프트를 인쇄하는 사용자 문자열 유효성 검사 기능을 구현하여보다 강력한 코드를 보장 할 수 있습니다. 나열된 모든 솔루션은 명령 줄 입력에서 단일 문자열 인수 만 구문 분석하고 다중 단어 문자열은 읽지 않습니다.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::prev_permutation(str.begin(), str.end()));
}
template <typename T>
T &validateInput(T &val) {
while (true) {
cout << "Enter string to print permutations: ";
if (cin >> val) {
break;
} else {
if (cin.eof()) exit(EXIT_SUCCESS);
cout << "Enter string to print permutations\n";
cin.clear();
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
}
}
return val;
}
int main() {
string input;
validateInput(input);
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
출력:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook