文字列のすべての順列を C++ で出力する
胡金庫
2023年10月12日
C++
C++ String
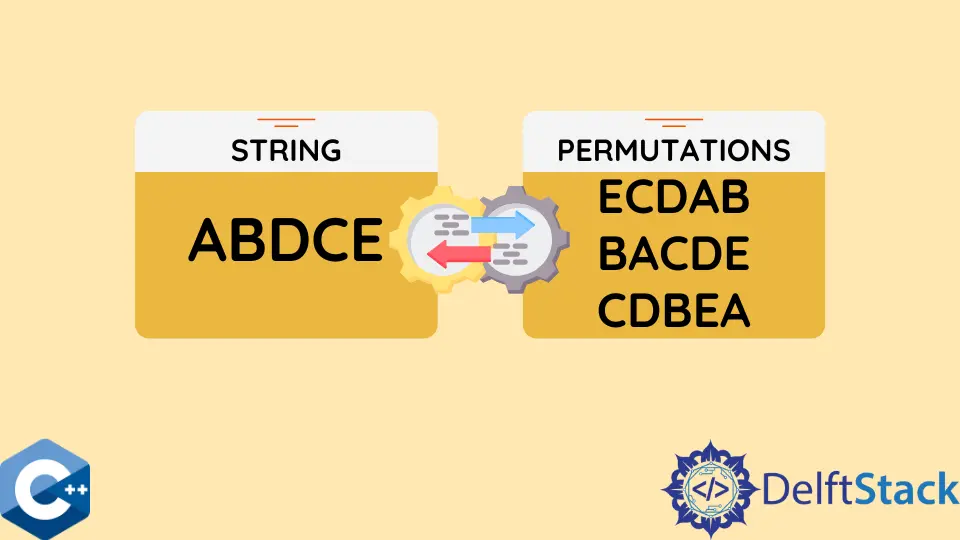
この記事では、指定された文字列のすべての順列を C++ で出力する方法を紹介します。
C++ で std::next_permutation
を使用して文字列のすべての順列を出力する
std:next_permutation
アルゴリズムは、要素の順列が辞書式順序で昇順になるように指定された範囲を変更し、そのような順列が存在する場合は真のブール値が返されます。この関数は、std::string
オブジェクトを操作して、文字列文字が降順で並べ替えられている場合にその順列を生成できます。std::sort
アルゴリズムと std::greater
関数オブジェクトを使用して文字列を並べ替え、false が返されるまで next_permutation
を呼び出すことができます。後者は、next_permutation
ステートメントを条件式として受け取り、各サイクルで文字列を cout
ストリームに出力する do...while
ループを使用して実装できます。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::next_permutation(str.begin(), str.end()));
}
int main() {
string input;
cout << "Enter string to print permutations: ";
cin >> input;
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
出力:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
C++ で std::prev_permutation
を使用して文字列のすべての順列を出力する
または、STL の別のアルゴリズム-std::prev_permutation
を利用して、同じ辞書式順序で指定された範囲の新しい順列を生成しますが、シーケンスが提供されたときに前の順列を保存します。最終的な解決策は、prev_permutation
関数が while
ループ条件で呼び出されることを除いて、前の例と同じままです。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::prev_permutation(str.begin(), str.end()));
}
int main() {
string input;
cout << "Enter string to print permutations: ";
cin >> input;
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
出力:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
さらに、ユーザーが有効な文字列を入力するまで入力プロンプトを出力するユーザー文字列検証関数を実装することで、より堅牢なコードを確保できます。リストされているすべてのソリューションは、コマンドライン入力からの単一の文字列引数のみを解析し、複数の単語の文字列は読み取られないことに注意してください。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::prev_permutation(str.begin(), str.end()));
}
template <typename T>
T &validateInput(T &val) {
while (true) {
cout << "Enter string to print permutations: ";
if (cin >> val) {
break;
} else {
if (cin.eof()) exit(EXIT_SUCCESS);
cout << "Enter string to print permutations\n";
cin.clear();
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
}
}
return val;
}
int main() {
string input;
validateInput(input);
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
出力:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫