Python でイテレーターとジェネレーターを区別する
Jesse John
2023年1月30日
Python
Python Iterator
Python Generator
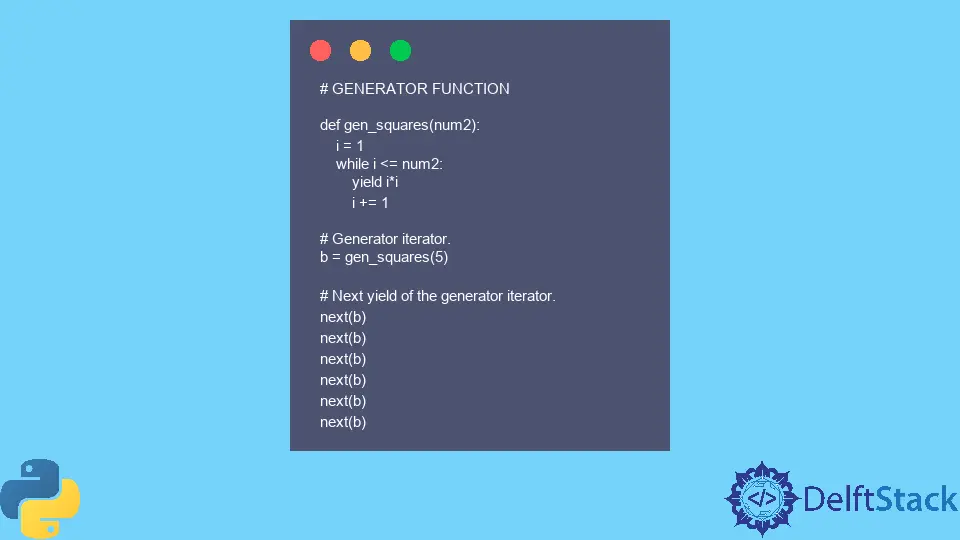
イテレーターとジェネレーターは、出力を生成したり、コードを 1 ビットずつ繰り返し処理したりするのに役立ちます。この記事では、Python のイテレーターとジェネレーターの基本的な違いを簡単な例で学びます。
Python のイテレーター
イテレーターの基本的な機能は次のとおりです。
- イテレーターは、イテレータープロトコルを実装するクラスを使用して作成されたオブジェクトです。これは、クラスに
__iter__
メソッドと__next__
メソッドが定義されていることを意味します。 __next__
メソッドは、return
ステートメントを使用して値を返します。return
ステートメントはそのメソッドの最後の行である必要があるため、return
ステートメントの前に__next__
の次の実行で使用される変数を更新する必要があります。
最も単純なケースでは、__iter__
が self
を返します。
Python のジェネレータ
ジェネレータの基本的な機能は次のとおりです。
- ジェネレーターは機能です。
- ジェネレーター関数は、
return
キーワードの代わりにyield
キーワードを使用します。
2.1yield
キーワードは値を生成し、関数の実行を一時停止します。
2.2next()
への次の呼び出しは、yield
ステートメントの後にコードの実行を再開します。
ジェネレーター関数を使用すると、クラスを使用してイテレーターを作成するときに必要な余分なコードをすべて使用せずに、ジェネレーターイテレーターを作成できます。
Python でのイテレーターとジェネレーターの例
次の 2つの例は、前の 2つのセクションで説明したポイントを強調しています。どちらの例も、1 から始まる整数の 2 乗を生成する方法を示しています。
最初の例は、イテレーターを使用してそれがどのように行われるかを示しています。2 番目の例は、ジェネレーターを使用した同等のコードを示しています。
Iterator のサンプルコード:
# ITERATOR (Class)
class squares(object):
def __init__(self, num1):
self.nxt_sq_of = 1
self.lim = num1
def __iter__(self):
return self
def __next__(self):
if self.nxt_sq_of <= self.lim:
ret_sq_of = self.nxt_sq_of
self.nxt_sq_of += 1
return ret_sq_of * ret_sq_of
else:
raise StopIteration
# Iterator Object
a = squares(6)
# Next value of the iterator.
next(a)
next(a)
next(a)
next(a)
next(a)
next(a)
next(a)
next(a)
# Using the iterator in a loop.
a1 = squares(6)
while True:
print(next(a1))
出力:
next(a)
Out[3]: 1
next(a)
Out[4]: 4
next(a)
Out[5]: 9
next(a)
Out[6]: 16
next(a)
Out[7]: 25
next(a)
Out[8]: 36
next(a)
Traceback (most recent call last):
File "<ipython-input-9-15841f3f11d4>", line 1, in <module>
next(a)
File "<ipython-input-1-9dbe8e565876>", line 17, in __next__
raise StopIteration
StopIteration
ジェネレータのサンプルコード:
# GENERATOR FUNCTION
def gen_squares(num2):
i = 1
while i <= num2:
yield i * i
i += 1
# Generator iterator.
b = gen_squares(5)
# Next yield of the generator iterator.
next(b)
next(b)
next(b)
next(b)
next(b)
next(b)
出力:
next(b)
Out[3]: 1
next(b)
Out[4]: 4
next(b)
Out[5]: 9
next(b)
Out[6]: 16
next(b)
Out[7]: 25
next(b)
Traceback (most recent call last):
File "<ipython-input-8-adb3e17b0219>", line 1, in <module>
next(b)
StopIteration
まとめ
Python のジェネレーターを使用すると、ジェネレーターの反復子を作成するための簡潔なコードを記述できることがわかります。
一方、イテレーターは、プログラマーが __iter__
メソッドのカスタムコードを記述できるため、はるかに強力です。
詳細については、以下を参照してください。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Jesse John