Python 中迭代器和生成器的區別
Jesse John
2023年1月30日
Python
Python Iterator
Python Generator
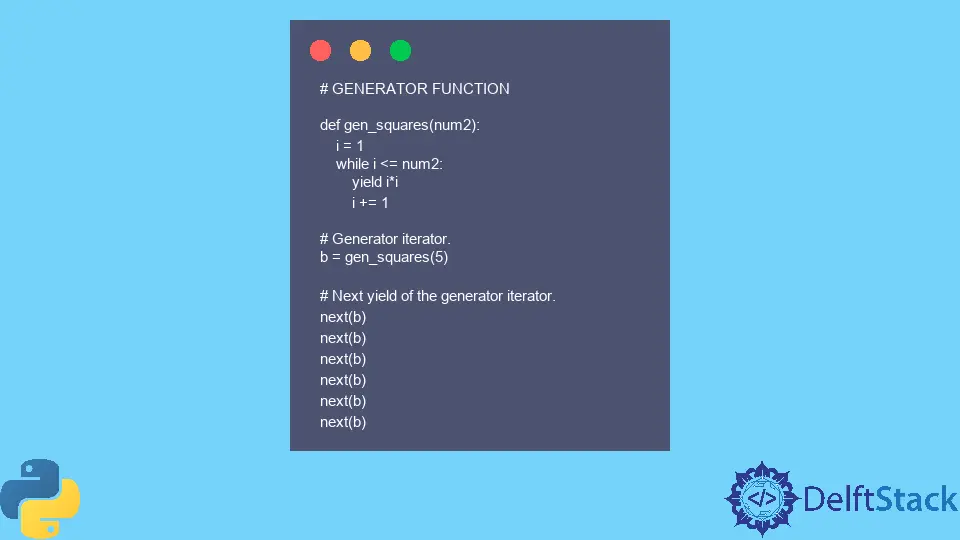
迭代器和生成器幫助我們生成一些輸出或迭代地處理一些程式碼,一次一位。在本文中,我們將通過一個簡單的示例瞭解 Python 的迭代器和生成器之間的一些基本區別。
Python 中的迭代器
迭代器的基本特徵如下:
- 迭代器是使用實現迭代器協議的類建立的物件。這意味著該類定義了
__iter__
和__next__
方法。 __next__
方法使用return
語句返回一個值。由於return
語句必須是該方法的最後一行,我們必須在return
語句之前更新要在__next__
下一次執行中使用的變數。
在最簡單的情況下,我們將看到,__iter__
返回 self
。
Python 中的生成器
生成器的基本特徵如下:
- 生成器是一個函式。
- 生成器函式使用
yield
關鍵字而不是return
關鍵字。
2.1yield
關鍵字產生一個值並暫停函式的執行。
2.2 對next()
的下一次呼叫在yield
語句之後恢復程式碼的執行。
生成器函式允許我們建立生成器迭代器,而無需使用類建立迭代器時所需的所有額外程式碼。
Python 中的迭代器和生成器示例
以下兩個示例突出了前兩節中提到的要點。這兩個示例都說明了如何生成從 1 開始的整數平方。
第一個示例顯示瞭如何使用迭代器完成它。第二個示例顯示了使用生成器的等效程式碼。
迭代器的示例程式碼:
# ITERATOR (Class)
class squares(object):
def __init__(self, num1):
self.nxt_sq_of = 1
self.lim = num1
def __iter__(self):
return self
def __next__(self):
if self.nxt_sq_of <= self.lim:
ret_sq_of = self.nxt_sq_of
self.nxt_sq_of += 1
return ret_sq_of * ret_sq_of
else:
raise StopIteration
# Iterator Object
a = squares(6)
# Next value of the iterator.
next(a)
next(a)
next(a)
next(a)
next(a)
next(a)
next(a)
next(a)
# Using the iterator in a loop.
a1 = squares(6)
while True:
print(next(a1))
輸出:
next(a)
Out[3]: 1
next(a)
Out[4]: 4
next(a)
Out[5]: 9
next(a)
Out[6]: 16
next(a)
Out[7]: 25
next(a)
Out[8]: 36
next(a)
Traceback (most recent call last):
File "<ipython-input-9-15841f3f11d4>", line 1, in <module>
next(a)
File "<ipython-input-1-9dbe8e565876>", line 17, in __next__
raise StopIteration
StopIteration
生成器示例程式碼:
# GENERATOR FUNCTION
def gen_squares(num2):
i = 1
while i <= num2:
yield i * i
i += 1
# Generator iterator.
b = gen_squares(5)
# Next yield of the generator iterator.
next(b)
next(b)
next(b)
next(b)
next(b)
next(b)
輸出:
next(b)
Out[3]: 1
next(b)
Out[4]: 4
next(b)
Out[5]: 9
next(b)
Out[6]: 16
next(b)
Out[7]: 25
next(b)
Traceback (most recent call last):
File "<ipython-input-8-adb3e17b0219>", line 1, in <module>
next(b)
StopIteration
まとめ
我們發現 Python 的生成器允許我們編寫簡潔的程式碼來建立生成器迭代器。
另一方面,迭代器更強大,因為它們允許程式設計師為 __iter__
方法編寫自定義程式碼。
有關詳細資訊,請參閱以下內容。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jesse John