Python は文字列内のすべてのオカレンスを検索する
-
Python で
string.count()
関数を使用して文字列内の部分文字列のすべての出現箇所を検索する -
Python でリスト内包表記と
startswith()
を使用して文字列内の部分文字列のすべての出現箇所を検索する -
Python で
re.finditer()
を使用して文字列内の部分文字列のすべての出現箇所を検索する
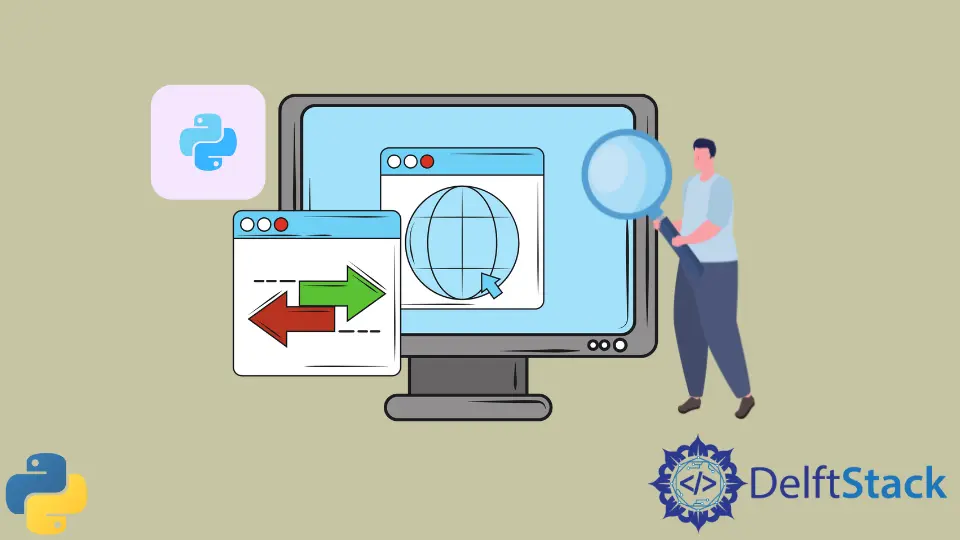
Python のサブストリングは、別のストリング内で発生する文字のクラスターです。部分文字列の処理は、多くの場合面倒です。そのような問題の 1つは、特定の文字列内の部分文字列のすべての出現を見つけることです。
このチュートリアルでは、Python で文字列内に出現するすべての部分文字列を見つけるためのさまざまな方法について説明します。
Python で string.count()
関数を使用して文字列内の部分文字列のすべての出現箇所を検索する
string.count()
は Python に組み込まれている関数で、特定の文字列内の部分文字列の出現回数または出現回数を返します。さらに、開始位置と終了位置のインデックスを指定するための追加パラメータ start
と end
があります。
count()
メソッドは文字列をトラバースし、特定のサブ文字列が文字列内で発生した回数を返します。
次のコードは、string.count()
関数を使用して、文字列内のすべての部分文字列を検索します。
# defining string and substring
str1 = "This dress looks good; you have good taste in clothes."
substr = "good"
# occurrence of word 'good' in whole string
count1 = str1.count(substr)
print(count1)
# occurrence of word 'good' from index 0 to 25
count2 = str1.count(substr, 0, 25)
print(count2)
出力:
2
1
これは簡単な方法であり、どのような場合でも機能します。このメソッドの唯一の欠点は、文字列内で部分文字列が出現するさまざまなインデックスを返さないことです。
Python でリスト内包表記と startswith()
を使用して文字列内の部分文字列のすべての出現箇所を検索する
このメソッドには、リスト内包表記と startswith()
メソッドの 2つが必要です。
startswith()
関数は、サブストリングの開始インデックスを取得するタスクを実行し、リスト内包表記を使用して、完全なターゲットストリングを反復処理します。
次のコードは、リスト内包表記と startswith()
を使用して、文字列内のすべての部分文字列を検索します。
# defining string
str1 = "This dress looks good; you have good taste in clothes."
# defining substring
substr = "good"
# printing original string
print("The original string is : " + str1)
# printing substring
print("The substring to find : " + substr)
# using list comprehension + startswith()
# All occurrences of substring in string
res = [i for i in range(len(str1)) if str1.startswith(substr, i)]
# printing result
print("The start indices of the substrings are : " + str(res))
出力:
The original string is : This dress looks good; you have good taste in clothes.
The substring to find : good
The start indices of the substrings are : [17, 34]
Python で re.finditer()
を使用して文字列内の部分文字列のすべての出現箇所を検索する
re.finditer()
は、Python がプログラマーがコードで使用するために提供する正規表現ライブラリの関数です。これは、文字列内の特定のパターンの出現を見つけるタスクを実行するのに役立ちます。この関数を使用するには、最初に正規表現ライブラリ re
をインポートする必要があります。
re.finditer()
は、構文で pattern
および string
パラメーターを使用します。この場合、パターンは部分文字列を参照します。
次のコードは、re.finditer()
関数を使用して、文字列内のすべての部分文字列を検索します。
import re
# defining string
str1 = "This dress looks good; you have good taste in clothes."
# defining substring
substr = "good"
print("The original string is: " + str1)
print("The substring to find: " + substr)
result = [_.start() for _ in re.finditer(substr, str1)]
print("The start indices of the substrings are : " + str(result))
出力:
The original string is: This dress looks good; you have good taste in clothes.
The substring to find: good
The start indices of the substrings are : [17, 34]
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn