Python を使用して Pandas データ フレームを Google スプレッドシートにエクスポートする
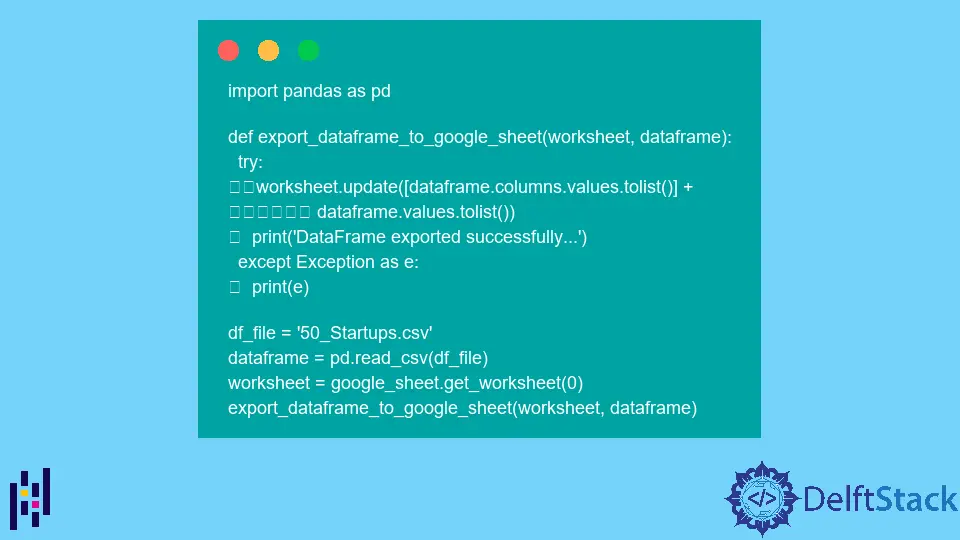
Google スプレッドシートは、Google が提供するオンラインの Web ベースのスプレッドシート プログラムです。 これは、ユーザーがスプレッドシートを作成および変更し、データをオンラインで共有できるリアルタイム アプリケーションです。
ほとんどの組織では、複数のユーザーに 1つのスプレッドシートで同時に作業するように依頼しています。 この共同作業は、ドキュメント内で特定のユーザーが行ったすべての更新を追跡します。
Pandas データ フレームを 2 次元データ構造、つまり、2 次元配列に似ているがラベル付きの軸を持つ行と列を持つテーブルとして使用できます。
Pandas データ フレームを Google スプレッドシートにエクスポートする場合は、このチュートリアルで説明されているように、Python を使用してエクスポートできます。
Python を使用して Pandas データ フレームを Google スプレッドシートにエクスポートする
Pandas データ フレームを Google シートにエクスポートするには、以下に示す 3つの手順に従う必要があります。
- プロジェクトを Google API コンソールと同期します。
- Google Sheet API にアクセスします。
- Pandas データ フレームを Google スプレッドシートにエクスポートします。
-
プロジェクトを Google API コンソールと同期します。
プロジェクトを Google API コンソールと同期するには、まず Google Cloud Console を介してプロジェクトを作成する必要があります。
認証情報を作成するには、検索バーで検索して、以下の 2つの API を有効にする必要があります。
- Google ドライブ API
- Google シート API
次に、資格情報を作成する方法を教えてください。 そのためには、以下の手順に従う必要があります。
資格情報の作成
をクリックします。サービス アカウント
を選択し、サービス アカウント名
を入力して、完了
を押します (残りの情報はオプションです)。- 作成したら、それをクリックし、[キー] タブに移動して、キーを JSON 形式でダウンロードします。
ダウンロードされた JSON ファイルのコンテンツ (
service_account.json
ファイルに保存されます) は、次のようになります。{ "type": "service_account", "project_id": "some-project-id", "private_key_id": "eb...25", "private_key": "-----BEGIN PRIVATE KEY-----\nNrDyLw...jINQh/9\n-----END PRIVATE KEY-----\n", "client_email": "123...999-yourclientemail@projectid.iam.gserviceaccount.com", "client_id": "473...hd.apps.googleusercontent.com", ... }
-
Google シート API にアクセスします。
以下の Python ライブラリをインストールして、Google スプレッドシートに接続して操作します。
gspread
PS C:\> pip install gspread
oauth2client
PS C:\> pip install oauth2client
ダウンロードした API を使ってコードで
client
を作成し、Google スプレッドシートに接続してみましょう。コード例 (
demo.py
に保存):import gspread from oauth2client.service_account import ServiceAccountCredentials def create_connection(service_file): client = None scope = [ "https://www.googleapis.com/auth/drive", "https://www.googleapis.com/auth/drive.file", ] try: credentials = ServiceAccountCredentials.from_json_keyfile_name( service_file, scope ) client = gspread.authorize(credentials) print("Connection established successfully...") except Exception as e: print(e) return client service = "service_account.json" # downloaded Credential file in JSON format client = create_connection(service)
まず、
gspread
をインポートし、Python パッケージouth2client
から、service_account.py
ファイルに格納されているクラスServiceAccountCredentials
をインポートしました。関数
create_connection()
はclient
を Google スプレッドシートに接続します。 クレデンシャルキーが保存される引数としてservice_file
を取ります。service_account.json
をdemo.py
と同じディレクトリに保持するか、そのパスをservice
変数に保存することを忘れないでください。 この関数内で、ServiceAccountCredentials.from_json_keyfile_name()
を使用してcredentials
を作成します。次に、
credentials
をgspread.authorize()
に提供します。gspread.authorize()
はその信頼性をチェックし、service_file
に保存されているキーが有効な場合はclient
を作成します。エラーが発生した場合、この関数はプログラムを中断することなく
Exception
を出力します。 -
作成した Google シートに Pandas データ フレームをエクスポートします。
Pandas データ フレームを Google シートにエクスポートするには、まずそれを作成する必要があります。 Google シートを作成するコードを書きましょう。
コード例 (
demo.py
に保存):def create_google_sheet(client, sheet_name): google_sheet = None try: google_sheet = client.create(sheet_name) google_sheet.share( "@gmail.com", # enter the email you want to create a google sheet on perm_type="user", role="writer", ) print("Google Sheet created successfully...") except Exception as e: print(e) return google_sheet service = "service_account.json" # downloaded Credential file in JSON format client = create_connection(service) sheet_name = "50_Startups" # google sheet name of your choice google_sheet = create_google_sheet(client, sheet_name)
関数
create_google_sheet
は、作成済みのclient
とsheet_name
を引数として受け取り、client.create()
関数を使用して Google シートを作成します。この Google シートは、ダウンロードした API を使用して構築された
client
で作成されることに注意してください。 そのため、サービス アカウントの作成に使用したメールと共有し、google_sheet.share()
関数を使用して書き込み権限を付与する必要があります。ここでの最後のステップは、データを Google スプレッドシートにエクスポートすることです。 これを行うには、以下を使用して Python ライブラリ
pandas
をインストールする必要があります。PS C:\> pip install pandas
Pandas データ フレームを最終的に Google シートにエクスポートするコードを実行してみましょう。
コード例 (
demo.py
に保存):import pandas as pd def export_dataframe_to_google_sheet(worksheet, dataframe): try: worksheet.update( [dataframe.columns.values.tolist()] + dataframe.values.tolist() ) print("DataFrame exported successfully...") except Exception as e: print(e) df_file = "50_Startups.csv" dataframe = pd.read_csv(df_file) worksheet = google_sheet.get_worksheet(0) export_dataframe_to_google_sheet(worksheet, dataframe)
デフォルトでは、Google スプレッドシート内に既に 1つのワークシートが作成されています。 google_sheet.get_worksheet(0)
として index を使用して google_sheet
からアクセスできます。
次に、関数 export_dataframe_to_google_sheet()
を使用してデータ フレームをエクスポートします。 データ フレームを一覧表示し、Google シートにエクスポートするだけです。
次に、Python ファイル demo.py
を次のように実行します。
PS C:\> python demo.py
出力 (コンソールに出力):
一方、Google シートにエクスポートされたデータ フレームは次のとおりです。