Kotlin の Sleep 関数を使用してスレッドの実行を一時停止する
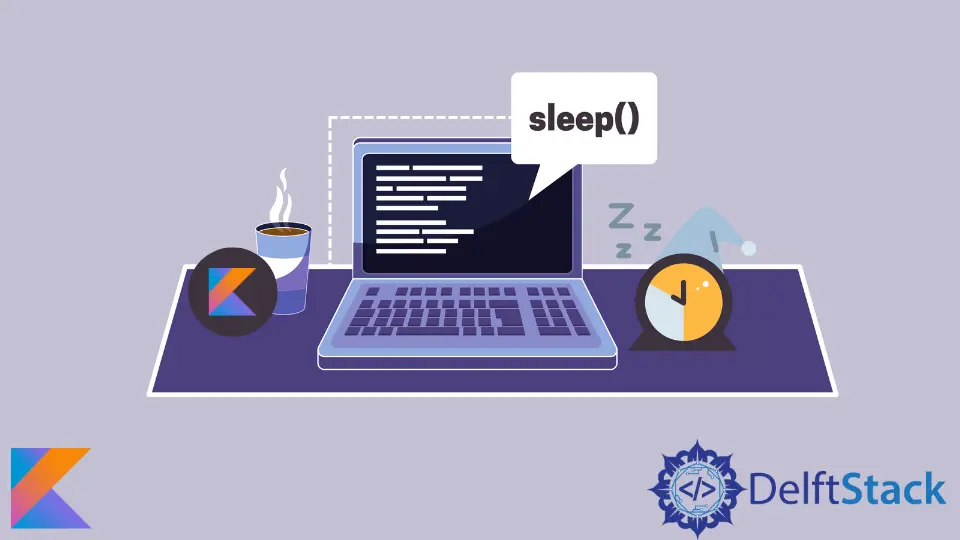
Java には、現在のスレッドの実行を一時停止する wait()
関数があります。スレッドは、別のスレッドが入り、スリープしているスレッドに通知するまでスリープします。
しかし、Kotlin で利用可能な同等のものはありますか?Kotlin には wait()
関数はありませんが、sleep()
関数はあります。
Kotlin の sleep()
関数は、特定の coroutine の実行を一時停止します。これにより実行が一時停止することはありませんが、他の coroutine の実行が可能になります。
この記事では、Kotlin で sleep()
関数を使用する方法について説明します。それに加えて、delay()
関数を使用して coroutine を一時停止する別の方法も学習します。
KotlinThread.Sleep()
関数の使用
Thread.sleep()
関数を使用して、コルーチンをスリープ状態にし、他の独立したコルーチンを実行できるようにすることができます。
sleep()
関数を使用するための構文は次のとおりです。
Thread.sleep(milliseconds)
これは、Kotlinsleep()
関数を使用して、coroutine の実行を 3 秒中断する例です。
fun main() {
println("Suspending execution")
try {
// making the coroutine sleep for 3 seconds
Thread.sleep(3000)
} catch (e: InterruptedException) {
e.printStackTrace()
}
println("Resuming execution")
}
出力:
KotlinTimeUnit``Sleep()
関数の使用
Thread.sleep()
と同様に、TimeUnit
を使用してスレッドの実行を一時停止することもできます。
Thread
手法は入力としてミリ秒数のみを受け入れますが、TimeUnit
は 7つの異なる時間単位を受け入れます。これらの 7つの時間単位は次のとおりです。
- ナノ秒
- マイクロ秒
- ミリ秒
- 秒
- 分
- 時間
- 日
TimeUnit
は、渡された値を自動的にミリ秒に変換します。これは、sleep()
関数が受け入れます。
TimeUnit
メソッドは、java.util.concurrent
ライブラリの一部です。したがって、このメソッドを使用するには、concurrent
ライブラリをインポートする必要があります。
これは、TimeUnit
の Kotlinsleep()
関数を示す例です。
import java.util.concurrent.TimeUnit
fun main() {
println("Suspending execution")
try {
// // making the coroutine sleep for 3 seconds
TimeUnit.SECONDS.sleep(3)
} catch (e: InterruptedException) {
e.printStackTrace()
}
println("Resuming execution")
}
出力:
KotlinDelay()
関数の使用
Kotlin の sleep()
関数に加えて、delay()
関数を使用して、coroutine の実行を一時停止することもできます。delay()
関数は、ミリ秒を入力として受け入れます。
Kotlindelay()
関数の使用法を示す例を次に示します。
import kotlinx.coroutines.*
fun main() = runBlocking {
launch { // launches a new coroutine
delay(2000L) // This delays the execution by 2 seconds allowing other coroutines to run independently
println("welcome!") // This will be printed after the delay
}
print("Hi and ") // This will be printed first since the other coroutine is delayed
}
出力:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn