Kotlin 절전 기능을 사용하여 스레드 실행 일시 중단
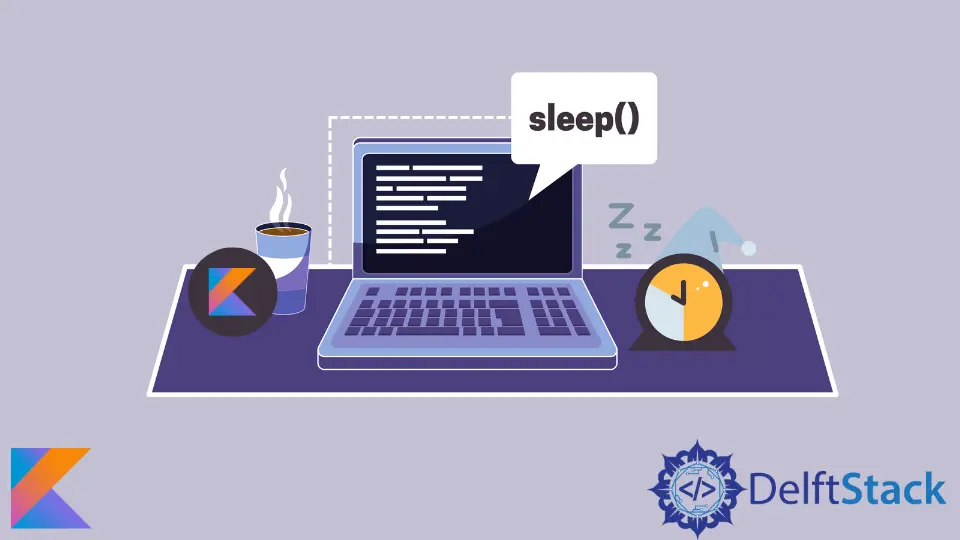
Java에는 현재 스레드의 실행을 일시 중지하는 wait()
함수가 있습니다. 스레드는 다른 스레드가 들어와 잠자는 스레드에 알릴 때까지 잠자기 상태입니다.
그러나 Kotlin에서 동등한 기능을 사용할 수 있습니까? Kotlin에는 wait()
기능이 없지만 sleep()
기능은 있습니다.
Kotlin sleep()
함수는 특정 코루틴의 실행을 일시 중단합니다. 이것은 실행을 일시 중지하지 않지만 다른 코루틴의 실행을 허용합니다.
이 기사에서는 Kotlin에서 sleep()
함수를 사용하는 방법을 설명합니다. 그 외에도 delay()
함수를 사용하여 코루틴을 일시 중단하는 또 다른 방법을 배웁니다.
Kotlin Thread.Sleep()
함수 사용
Thread.sleep()
함수를 사용하여 코루틴을 절전 모드로 전환하고 다른 독립 코루틴을 실행할 수 있습니다.
sleep()
함수를 사용하는 구문은 다음과 같습니다.
Thread.sleep(milliseconds)
다음은 Kotlin sleep()
함수를 사용하여 코루틴 실행을 3초 동안 일시 중단하는 예입니다.
fun main() {
println("Suspending execution")
try {
// making the coroutine sleep for 3 seconds
Thread.sleep(3000)
} catch (e: InterruptedException) {
e.printStackTrace()
}
println("Resuming execution")
}
출력:
Kotlin TimeUnit
Sleep()
함수 사용
Thread.sleep()
과 같이 TimeUnit
을 사용하여 스레드 실행을 일시 중단할 수도 있습니다.
Thread
기술은 입력으로 밀리초 수만 허용하지만 TimeUnit
은 7개의 다른 시간 단위를 허용합니다. 이 7개의 시간 단위는 다음과 같습니다.
- 나노초
- 마이크로초
- 밀리세컨드
- 초
- 분
- 시간
- 일
TimeUnit
은 전달된 값을 sleep()
함수가 허용하는 밀리초로 자동 변환합니다.
TimeUnit
메소드는 java.util.concurrent
라이브러리의 일부입니다. 따라서 이 방법을 사용하려면 concurrent
라이브러리를 가져와야 합니다.
다음은 TimeUnit
의 Kotlin sleep()
함수를 보여주는 예입니다.
import java.util.concurrent.TimeUnit
fun main() {
println("Suspending execution")
try {
// // making the coroutine sleep for 3 seconds
TimeUnit.SECONDS.sleep(3)
} catch (e: InterruptedException) {
e.printStackTrace()
}
println("Resuming execution")
}
출력:
Kotlin Delay()
함수 사용
Kotlin sleep()
함수 외에도 delay()
함수를 사용하여 코루틴 실행을 일시 중단할 수도 있습니다. delay()
함수는 밀리초도 입력으로 받아들입니다.
다음은 Kotlin delay()
함수의 사용법을 보여주는 예입니다.
import kotlinx.coroutines.*
fun main() = runBlocking {
launch { // launches a new coroutine
delay(2000L) // This delays the execution by 2 seconds allowing other coroutines to run independently
println("welcome!") // This will be printed after the delay
}
print("Hi and ") // This will be printed first since the other coroutine is delayed
}
출력:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn