使用 Kotlin sleep 函数暂停线程的执行
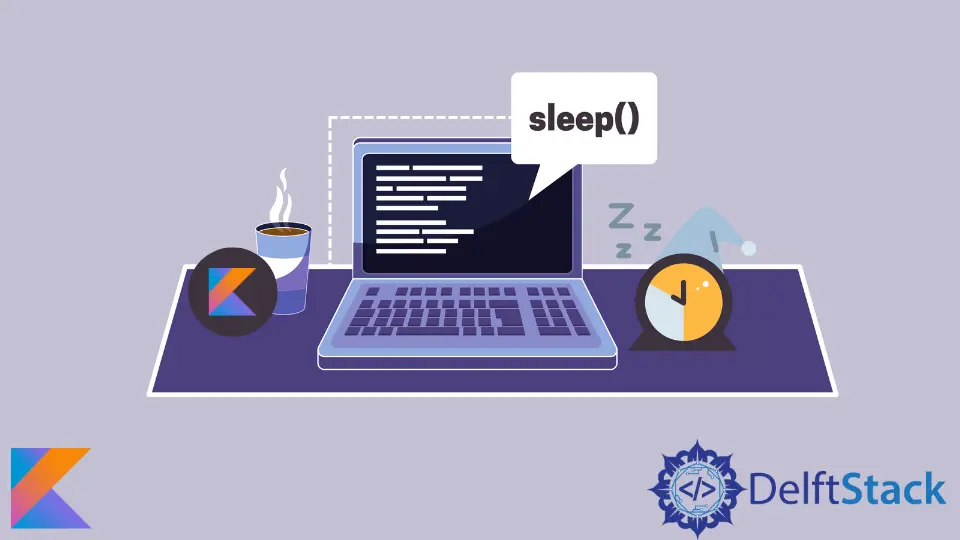
Java 有一个 wait()
函数可以暂停当前线程的执行。线程休眠直到另一个线程进入并通知休眠线程。
但是 Kotlin 中是否有等价物?Kotlin 没有 wait()
函数,但它具有 sleep()
函数。
Kotlin sleep()
函数暂停特定协程的执行。虽然这不会暂停执行,但它允许执行其他协程。
本文介绍如何在 Kotlin 中使用 sleep()
函数。除此之外,我们还将学习另一种使用 delay()
函数暂停协程的方法。
Kotlin Thread.Sleep()
函数的使用
我们可以使用 Thread.sleep()
函数让协程进入睡眠状态并允许其他独立协程运行。
使用 sleep()
函数的语法是:
Thread.sleep(milliseconds)
这是一个示例,我们使用 Kotlin sleep()
函数将协程的执行暂停 3 秒。
fun main() {
println("Suspending execution")
try {
// making the coroutine sleep for 3 seconds
Thread.sleep(3000)
} catch (e: InterruptedException) {
e.printStackTrace()
}
println("Resuming execution")
}
输出:
Kotlin TimeUnit
Sleep()
函数的使用
像 Thread.sleep()
一样,我们也可以使用 TimeUnit
来暂停线程的执行。
Thread
技术只接受毫秒数作为输入,TimeUnit
接受 7 个不同的时间单位。这 7 个时间单位是:
- 纳秒
- 微秒
- 毫秒
- 秒
- 分钟
- 小时
- 天
TimeUnit
自动将传递的值转换为 sleep()
函数接受的毫秒数。
TimeUnit
方法是 java.util.concurrent
库的一部分。因此,我们需要导入 concurrent
库来使用此方法。
这是一个示例,展示了来自 TimeUnit
的 Kotlin sleep()
函数。
import java.util.concurrent.TimeUnit
fun main() {
println("Suspending execution")
try {
// // making the coroutine sleep for 3 seconds
TimeUnit.SECONDS.sleep(3)
} catch (e: InterruptedException) {
e.printStackTrace()
}
println("Resuming execution")
}
输出:
Kotlin Delay()
函数的使用
除了 Kotlin 的 sleep()
函数,我们还可以使用 delay()
函数来暂停协程的执行。delay()
函数也接受毫秒作为输入。
这是一个展示 Kotlin delay()
函数用法的示例。
import kotlinx.coroutines.*
fun main() = runBlocking {
launch { // launches a new coroutine
delay(2000L) // This delays the execution by 2 seconds allowing other coroutines to run independently
println("welcome!") // This will be printed after the delay
}
print("Hi and ") // This will be printed first since the other coroutine is delayed
}
输出:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn