jQuery を使用してタイマーと時計を作成する
- jQuery でタイマーを作成する
-
setTimeout
と jQuerytext()
API を使用して jQuery で時計を作成する -
setInterval
と jQueryhtml()
API を使用して jQuery で時計を作成する
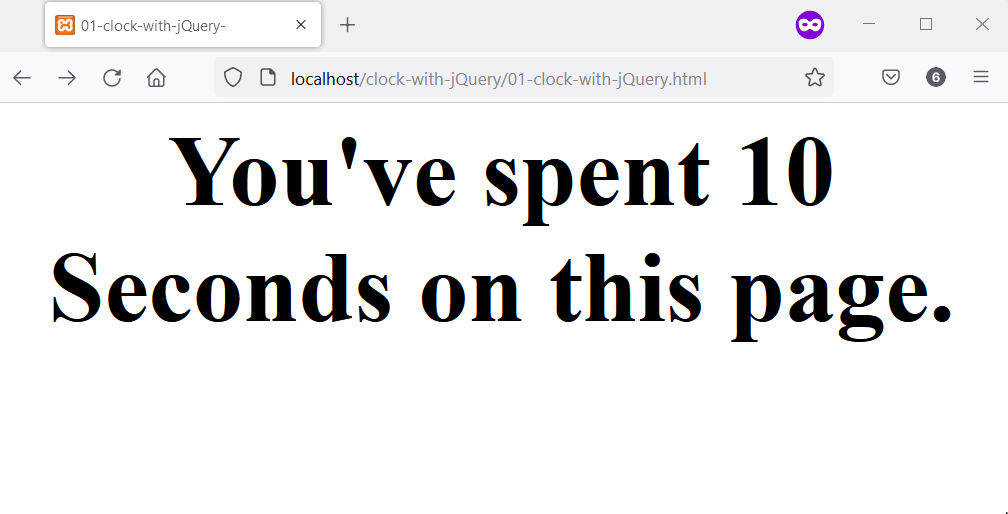
この記事では、jQuery でタイマーと時計を作成する 3つの例を紹介します。 最初の例は、ページを開くと開始するタイマーですが、2 番目と 3 番目の例には異なる方法で実装された時計が含まれています。
jQuery でタイマーを作成する
jQuery でタイマーを作成するには、Date
オブジェクト、setInterval()
、Math.round()
、および jQuery text()
API が必要です。 Date
オブジェクトを使用すると、時差を秒単位で計算できます。
同時に、setInterval()
関数は常に計算を実行します。 その結果、Web ブラウザーでスクリプトを起動すると、タイマーが表示されます。
jQuery text()
API の仕事は、選択した HTML 要素にタイマーを配置することです。 最後に、Math.round()
関数を使用すると、タイマーを 10 進数ではなく整数として表示できます。
以下にすべてをまとめました。コードを実行して、Web ブラウザーで結果を確認できます。
<!DOCTYPE html>
<html lang="en">
<head>
<title>01-clock-with-jQuery-</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<div style="text-align: center; font-size: 5em;">
<b class="jquery_timer"></b>
</div>
<script>
// Create a new date object.
var date_object = new Date;
// Use setInterval to run a custom function
// that displays the timer at all times.
setInterval(function() {
// Additional information to aid user experience.
let prepend_text = "You've spent ";
let append_text = " on this page."
// Use Math.round() to remove any decimal
// number from the timer.
$('.jquery_timer').text(prepend_text + Math.round((new Date - date_object) / 1000) + " Seconds" + append_text);
}, 1000);
</script>
</body>
</html>
出力:
setTimeout
と jQuery text()
API を使用して jQuery で時計を作成する
この時計の核となるのは、Date
オブジェクトを使用して日付を計算するカスタム関数です。 これを行うには、Date
オブジェクトの getHours()
、getMinutes()
、および getSeconds()
メソッドを使用します。
これら 3つの方法を組み合わせると、時計の時、分、秒を表示できます。 その後、jQuery text()
API を使用して、時計を HTML 要素に配置します。
その前に、定期的に実行されるカスタム関数で setTimeout()
を呼び出します。 後者は十分に小さく、時間が不正確な時間を表示しないようにする必要があります。
以下のコード例は、すべての情報を使用してクロックを実装しています。 HTML ボタンをクリックすると時計が表示され、興味深いものになります。
<!DOCTYPE html>
<html lang="en">
<head>
<title>02-clock-with-jQuery</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
button {
cursor: pointer;
}
</style>
</head>
<body>
<main style="text-align: center; font-size: 3em;">
<button class="show_clock" style="font-size: 1em;">Show Clock</button>
<h1 class="clock_location"></h1>
</main>
<script>
$(document).ready(function(){
function get_current_date(){
let current_date = new Date();
let hours = current_date.getHours();
let minutes = current_date.getMinutes();
let seconds = current_date.getSeconds();
// Add a leading zero to the seconds and minutes if
// they are less than 10
if (seconds < 10){
seconds = "0" + seconds;
}
if (minutes < 10) {
minutes = "0" + minutes;
}
$(".clock_location").text(hours+" : "+minutes+" : "+seconds);
setTimeout(function(){
get_current_date()
}, 500);
}
// Wait for the user to click the button
// before you show the clock.
$(".show_clock").click(get_current_date);
});
</script>
</body>
</html>
出力:
setInterval
と jQuery html()
API を使用して jQuery で時計を作成する
前の例と同様に、jQuery を使用して時計を作成しますが、別の方法があります。 これは、ここで setInterval()
関数と jQuery html()
API を使用するためです。
最初に、無名関数で setInterval()
関数を呼び出します。 この関数は、Web ブラウザーで日付を計算して表示します。
この関数は Date
オブジェクトを使用して現在の時、分、秒を取得します。 クロック パーツに先行ゼロを追加して、表示用に構成できます。
その後、jQuery を使用して、時計を表示する HTML 要素を取得します。 最後のステップは、構成された時間で jQuery html()
API を呼び出し、それを HTML 要素内に表示することです。
<!DOCTYPE html>
<html lang="en">
<head>
<title>03-clock-with-jQuery</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main style="text-align: center; font-size: 5em;">
<p class="clock_location"></p>
</main>
<script>
// Use setInterval to run an anonymous function
// every one milliseconds.
setInterval(function() {
let current_date = new Date();
let clock_hours = current_date.getHours();
let clock_minutes = current_date.getMinutes();
let clock_seconds = current_date.getSeconds();
// Use the ternary operator to add leading
// zeros to elements of the clock if they
// are less than 10.
if (clock_hours < 10) {
clock_hours = "0" + clock_hours;
}
if (clock_minutes < 10) {
clock_minutes = "0" + clock_minutes;
}
if (clock_seconds < 10){
clock_seconds = "0" + clock_seconds;
}
// Compose the current time
let clock_delimeter = ":";
let current_time = clock_hours + clock_delimeter + clock_minutes + clock_delimeter + clock_seconds;
$(".clock_location").html(current_time);
}, 1000);
</script>
</body>
</html>
出力:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn