jQuery를 사용하여 타이머 및 시계 만들기
- jQuery에서 타이머 만들기
-
setTimeout
및 jQuerytext()
API를 사용하여 jQuery에서 시계 만들기 -
setInterval
및 jQueryhtml()
API를 사용하여 jQuery에서 시계 만들기
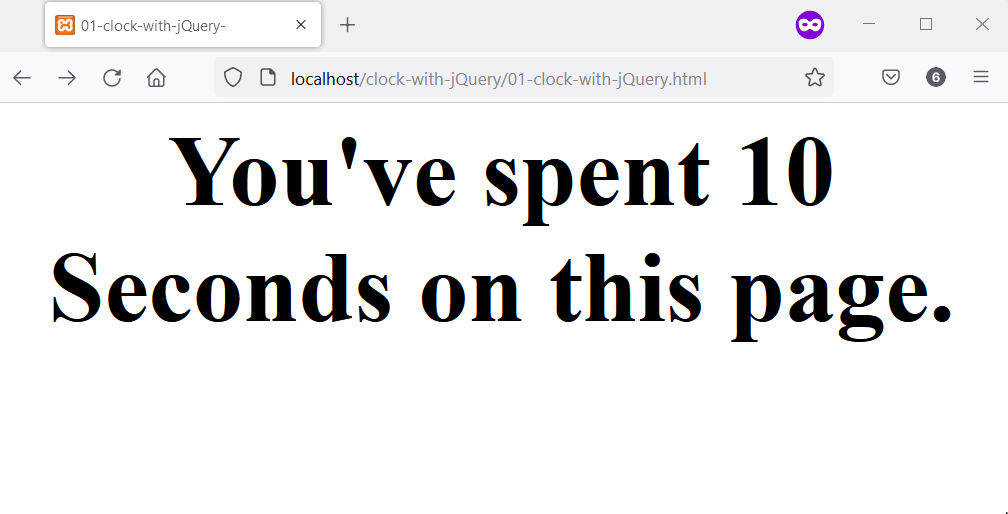
이 기사에서는 jQuery에서 타이머와 시계를 생성하는 세 가지 예를 소개합니다. 첫 번째 예는 페이지를 열면 시작되는 타이머이고 두 번째와 세 번째 예는 다르게 구현된 시계를 포함합니다.
jQuery에서 타이머 만들기
jQuery에서 타이머를 생성하려면 Date
객체, setInterval()
, Math.round()
및 jQuery text()
API가 필요합니다. 날짜
개체를 사용하면 시차를 초 단위로 계산할 수 있습니다.
동시에 setInterval()
함수는 항상 계산을 실행합니다. 결과적으로 웹 브라우저에서 스크립트를 실행할 때 타이머가 표시됩니다.
jQuery text()
API의 작업은 선택된 HTML 요소에 타이머를 배치하는 것입니다. 마지막으로 Math.round()
함수를 사용하면 타이머가 소수 값이 아닌 정수로 표시됩니다.
다음에서는 모든 것을 종합했으며 코드를 실행하여 웹 브라우저에서 결과를 볼 수 있습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<title>01-clock-with-jQuery-</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<div style="text-align: center; font-size: 5em;">
<b class="jquery_timer"></b>
</div>
<script>
// Create a new date object.
var date_object = new Date;
// Use setInterval to run a custom function
// that displays the timer at all times.
setInterval(function() {
// Additional information to aid user experience.
let prepend_text = "You've spent ";
let append_text = " on this page."
// Use Math.round() to remove any decimal
// number from the timer.
$('.jquery_timer').text(prepend_text + Math.round((new Date - date_object) / 1000) + " Seconds" + append_text);
}, 1000);
</script>
</body>
</html>
출력:
setTimeout
및 jQuery text()
API를 사용하여 jQuery에서 시계 만들기
이 시계의 핵심은 Date
개체를 사용하여 날짜를 계산하는 사용자 지정 함수입니다. 이를 위해 Date
개체의 getHours()
, getMinutes()
및 getSeconds()
메서드를 사용합니다.
이 세 가지 방법을 결합하면 시계의 시, 분, 초를 표시할 수 있습니다. 그런 다음 jQuery text()
API를 사용하여 HTML 요소에 시계를 배치합니다.
그 전에는 정기적으로 실행되는 사용자 정의 함수에서 setTimeout()
을 호출합니다. 후자는 충분히 작아야 하며 시간이 부정확하게 표시되지 않도록 해야 합니다.
아래 코드 예제는 모든 정보를 사용하여 시계를 구현했습니다. 흥미롭게 만들기 위해 HTML 버튼을 클릭한 후 시계를 표시합니다.
<!DOCTYPE html>
<html lang="en">
<head>
<title>02-clock-with-jQuery</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
button {
cursor: pointer;
}
</style>
</head>
<body>
<main style="text-align: center; font-size: 3em;">
<button class="show_clock" style="font-size: 1em;">Show Clock</button>
<h1 class="clock_location"></h1>
</main>
<script>
$(document).ready(function(){
function get_current_date(){
let current_date = new Date();
let hours = current_date.getHours();
let minutes = current_date.getMinutes();
let seconds = current_date.getSeconds();
// Add a leading zero to the seconds and minutes if
// they are less than 10
if (seconds < 10){
seconds = "0" + seconds;
}
if (minutes < 10) {
minutes = "0" + minutes;
}
$(".clock_location").text(hours+" : "+minutes+" : "+seconds);
setTimeout(function(){
get_current_date()
}, 500);
}
// Wait for the user to click the button
// before you show the clock.
$(".show_clock").click(get_current_date);
});
</script>
</body>
</html>
출력:
setInterval
및 jQuery html()
API를 사용하여 jQuery에서 시계 만들기
이전 예제와 마찬가지로 jQuery를 사용하여 시계를 만들지만 다른 방법이 있습니다. 여기서는 setInterval()
함수와 jQuery html()
API를 사용하기 때문입니다.
처음에는 익명 함수에서 setInterval()
함수를 호출합니다. 이 기능은 웹 브라우저에서 날짜를 계산하고 표시합니다.
이 함수는 Date
개체를 사용하여 현재 시, 분, 초를 가져옵니다. 시계 부분에 선행 0을 추가하고 표시용으로 구성할 수 있습니다.
그런 다음 jQuery를 사용하여 시계를 표시하려는 HTML 요소를 가져옵니다. 마지막 단계는 구성된 시간에 jQuery html()
API를 호출하고 HTML 요소 내에 표시하는 것입니다.
<!DOCTYPE html>
<html lang="en">
<head>
<title>03-clock-with-jQuery</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main style="text-align: center; font-size: 5em;">
<p class="clock_location"></p>
</main>
<script>
// Use setInterval to run an anonymous function
// every one milliseconds.
setInterval(function() {
let current_date = new Date();
let clock_hours = current_date.getHours();
let clock_minutes = current_date.getMinutes();
let clock_seconds = current_date.getSeconds();
// Use the ternary operator to add leading
// zeros to elements of the clock if they
// are less than 10.
if (clock_hours < 10) {
clock_hours = "0" + clock_hours;
}
if (clock_minutes < 10) {
clock_minutes = "0" + clock_minutes;
}
if (clock_seconds < 10){
clock_seconds = "0" + clock_seconds;
}
// Compose the current time
let clock_delimeter = ":";
let current_time = clock_hours + clock_delimeter + clock_minutes + clock_delimeter + clock_seconds;
$(".clock_location").html(current_time);
}, 1000);
</script>
</body>
</html>
출력:
![setInterval 함수를 사용하여 jQuery에서 시계](</img/jQuery/setinterval function.gif>를 사용하여 juery에서 시계
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn