Crear un temporizador y un reloj usando jQuery
- Crear un temporizador en jQuery
-
Cree un reloj en jQuery usando la API
setTimeout
y jQuerytext()
-
Cree un reloj en jQuery usando la API
setInterval
y jQueryhtml()
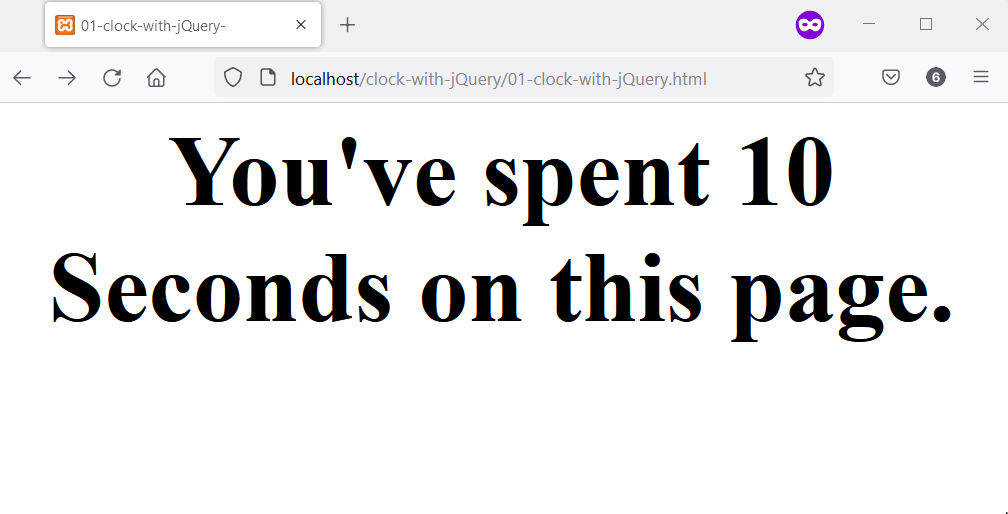
Este artículo presenta tres ejemplos de cómo crear un temporizador y un reloj en jQuery. El primer ejemplo es un temporizador que se inicia una vez que abre la página, mientras que el segundo y el tercer ejemplo contienen un reloj implementado de manera diferente.
Crear un temporizador en jQuery
Para crear un temporizador en jQuery, necesitará el objeto Date
, setInterval()
, Math.round()
y jQuery text()
API. El objeto Fecha
le permite calcular la diferencia horaria en segundos.
Al mismo tiempo, la función setInterval()
ejecutará los cálculos en todo momento. Como resultado, el temporizador se mostrará cuando inicie el script en su navegador web.
El trabajo de jQuery text()
API es colocar el temporizador en un elemento HTML seleccionado. Finalmente, la función Math.round()
permite que el temporizador se muestre como valores enteros, no decimales.
A continuación, lo juntamos todo y puede ejecutar el código para ver el resultado en su navegador web.
<!DOCTYPE html>
<html lang="en">
<head>
<title>01-clock-with-jQuery-</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<div style="text-align: center; font-size: 5em;">
<b class="jquery_timer"></b>
</div>
<script>
// Create a new date object.
var date_object = new Date;
// Use setInterval to run a custom function
// that displays the timer at all times.
setInterval(function() {
// Additional information to aid user experience.
let prepend_text = "You've spent ";
let append_text = " on this page."
// Use Math.round() to remove any decimal
// number from the timer.
$('.jquery_timer').text(prepend_text + Math.round((new Date - date_object) / 1000) + " Seconds" + append_text);
}, 1000);
</script>
</body>
</html>
Producción:
Cree un reloj en jQuery usando la API setTimeout
y jQuery text()
El núcleo de este reloj es una función personalizada que utiliza el objeto Fecha
para calcular la fecha. Para ello, utilizará los métodos getHours()
, getMinutes()
y getSeconds()
del objeto Date
.
Cuando se combinan, estos tres métodos le permitirán mostrar las horas, los minutos y los segundos del reloj. Luego, usará la API jQuery text()
para colocar el reloj en un elemento HTML.
Antes de eso, llamará a setTimeout()
en la función personalizada, que se ejecutará a intervalos regulares. Este último debe ser lo suficientemente pequeño y no hacer que la hora muestre una hora inexacta.
Nuestro ejemplo de código a continuación ha implementado el reloj usando toda la información. Mostrarás el reloj después de hacer clic en el botón HTML para que sea interesante.
<!DOCTYPE html>
<html lang="en">
<head>
<title>02-clock-with-jQuery</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
button {
cursor: pointer;
}
</style>
</head>
<body>
<main style="text-align: center; font-size: 3em;">
<button class="show_clock" style="font-size: 1em;">Show Clock</button>
<h1 class="clock_location"></h1>
</main>
<script>
$(document).ready(function(){
function get_current_date(){
let current_date = new Date();
let hours = current_date.getHours();
let minutes = current_date.getMinutes();
let seconds = current_date.getSeconds();
// Add a leading zero to the seconds and minutes if
// they are less than 10
if (seconds < 10){
seconds = "0" + seconds;
}
if (minutes < 10) {
minutes = "0" + minutes;
}
$(".clock_location").text(hours+" : "+minutes+" : "+seconds);
setTimeout(function(){
get_current_date()
}, 500);
}
// Wait for the user to click the button
// before you show the clock.
$(".show_clock").click(get_current_date);
});
</script>
</body>
</html>
Producción:
Cree un reloj en jQuery usando la API setInterval
y jQuery html()
Al igual que nuestro ejemplo anterior, creará un reloj con jQuery, pero de manera diferente. Esto se debe a que aquí utilizará la función setInterval()
y la API html()
de jQuery.
Al principio, llamará a la función setInterval()
en una función anónima. Esta función calculará y mostrará la fecha en su navegador web.
La función utilizará el objeto Fecha
para obtener la hora, los minutos y los segundos actuales. Puede agregar ceros iniciales a las partes del reloj y componerlas para mostrarlas.
Luego, use jQuery para tomar un elemento HTML donde le gustaría mostrar el reloj. El paso final es llamar a la API de jQuery html()
en el momento de la composición y mostrarlo dentro del elemento HTML.
<!DOCTYPE html>
<html lang="en">
<head>
<title>03-clock-with-jQuery</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main style="text-align: center; font-size: 5em;">
<p class="clock_location"></p>
</main>
<script>
// Use setInterval to run an anonymous function
// every one milliseconds.
setInterval(function() {
let current_date = new Date();
let clock_hours = current_date.getHours();
let clock_minutes = current_date.getMinutes();
let clock_seconds = current_date.getSeconds();
// Use the ternary operator to add leading
// zeros to elements of the clock if they
// are less than 10.
if (clock_hours < 10) {
clock_hours = "0" + clock_hours;
}
if (clock_minutes < 10) {
clock_minutes = "0" + clock_minutes;
}
if (clock_seconds < 10){
clock_seconds = "0" + clock_seconds;
}
// Compose the current time
let clock_delimeter = ":";
let current_time = clock_hours + clock_delimeter + clock_minutes + clock_delimeter + clock_seconds;
$(".clock_location").html(current_time);
}, 1000);
</script>
</body>
</html>
Producción:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn