JavaScript 抽象クラス
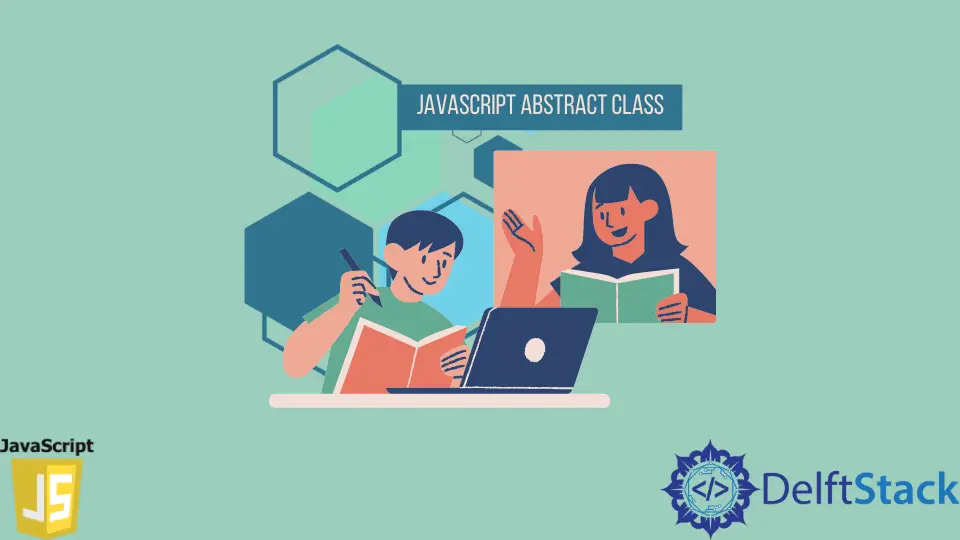
JavaScript 抽象クラスを使用する場合は、抽象化と継承という 2つの主要な概念を考慮する必要があります。
抽象化により、セキュリティを有効にし、特定の詳細を隠し、オブジェクトの重要な情報のみを表示します。一方、継承は別のクラスからプロパティを取得し、それらの間に親子関係を作成します。
JavaScript 抽象クラス
抽象クラスは、1つ以上の抽象メソッドを含むがインスタンス化できないクラスです。 抽象クラスはインスタンス化できませんが、メソッドを使用して拡張できます。
さらに、抽象クラスは継承する必要があり、具体的なクラスまたはサブクラスは、内部で宣言されたメソッドで抽象クラスを拡張する必要があります。
抽象メソッドは、宣言のみが可能で、実装を持たないメソッドです。
まず、抽象クラスを定義し、その中にコンストラクターを作成できます。 その後、抽象クラスを拡張するときに関数を定義する必要があります。
この例では、Fruit
を抽象クラス名として定義し、その中に 2つのメソッドを作成しました: color()
は果物の色を示し、eat()
は果物が食べられたかどうかを調べます。
ここで例に挙げた果物はすべて食べられるので、抽象クラス内で食べる
と表示するメソッドを実装しました。 しかし、各具象クラスを定義するときは、それぞれに color()
メソッドを実装する必要があります。
class Fruit {
constructor() {
if (this.constructor == Fruit) {
throw new Error('Abstract classes can\'t be instantiated.');
}
}
color() {
throw new Error('Method \'color()\' must be implemented.');
}
eat() {
console.log('eating');
}
}
抽象クラスを具象クラスで拡張する
抽象クラスを作成したら、具象クラスを作成する必要があります。 具象クラスを構築するときは、抽象クラスのすべての機能と動作を継承させる必要があります。
以下のスニペットは、抽象クラス用に作成された具象クラス Apple
と Orange
を示しています。 extends
キーワードを使用して、継承を実行します。
class Apple extends Fruit {
color() {
console.log('Red');
}
}
class Orange extends Fruit {
color() {
console.log('Orange');
}
}
抽象クラスと具象クラスを定義することで、抽象化と継承が実現されています。
完全なコードは、上記のコード スニペットと完全なコードを組み合わせて、図に示されている結果で構成されます。 ここでは、両方の具象クラスを抽象クラスと呼び、メソッドで実装された出力を表示します。
それとは別に、コンストラクターが抽象クラスと同じ名前の場合、エラーがスローされます。 しかし、具象クラスでインスタンス化すると、問題なく動作します。
コード:
// Abstract Class 'Fruit'.
class Fruit {
constructor() {
if (this.constructor == Fruit) {
throw new Error('Abstract classes can\'t be instantiated.');
}
}
// Method 'color'
color() {
throw new Error('Method \'color()\' must be implemented.');
}
// Method 'eat'
eat() {
console.log('eating');
}
}
// Concrete class 'Apple'
class Apple extends Fruit {
color() {
console.log('Red');
}
}
// Concrete class 'Orange'
class Orange extends Fruit {
color() {
console.log('Orange');
}
}
// Calling the classes
new Apple().eat();
new Orange().eat();
new Apple().color();
new Orange().color();
出力:
具象クラス Apple
でさえ color()
メソッドを実装していますが、banana()
は実装していません。 したがって、具象クラス banana()
は抽象クラスのプロパティのみを継承します。
そのため、メソッド color()
が呼び出されると、banana
は色を出力する代わりにエラーをスローします。
以下のコード スニペットは、継承の概念をよく表しています。
// Abstract class 'Fruit'
class Fruit {
constructor() {
if (this.constructor == Fruit) {
throw new Error('Abstract classes can\'t be instantiated.');
}
}
// Method 'color'
color() {
throw new Error('Method \'color()\' must be implemented.');
}
// Method 'eat'
eat() {
console.log('eating');
}
}
// Concrete class 'Apple'
class Apple extends Fruit {
color() {
console.log('Red');
}
}
// Concrete class 'Banana'
class Banana extends Fruit {}
// Calling the classes
new Apple().eat();
new Banana().eat();
new Apple().color();
new Banana().color();
出力:
それとは別に、インスタンスの作成もエラーをスローします。 抽象クラスはインスタンス化できないため、それらを呼び出すことはできません。
コード:
// Abstract class 'Fruit'
class Fruit {
constructor() {
if (this.constructor == Fruit) {
throw new Error('Abstract classes can\'t be instantiated.');
}
}
// Method 'color'
color() {
throw new Error('Method \'color()\' must be implemented.');
}
// Method 'eat'
eat() {
console.log('eating');
}
}
// Concrete class 'Apple'
class Apple extends Fruit {
color() {
console.log('Red');
}
}
// Calling the class
new Fruit();
出力:
まとめ
全体として、JavaScript やその他の OOP 言語でこの抽象化機能を使用すると、開発者と読者にとってコードの理解と保守が容易になり、コードの重複が減少します。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.