JavaScript でクラスを拡張する
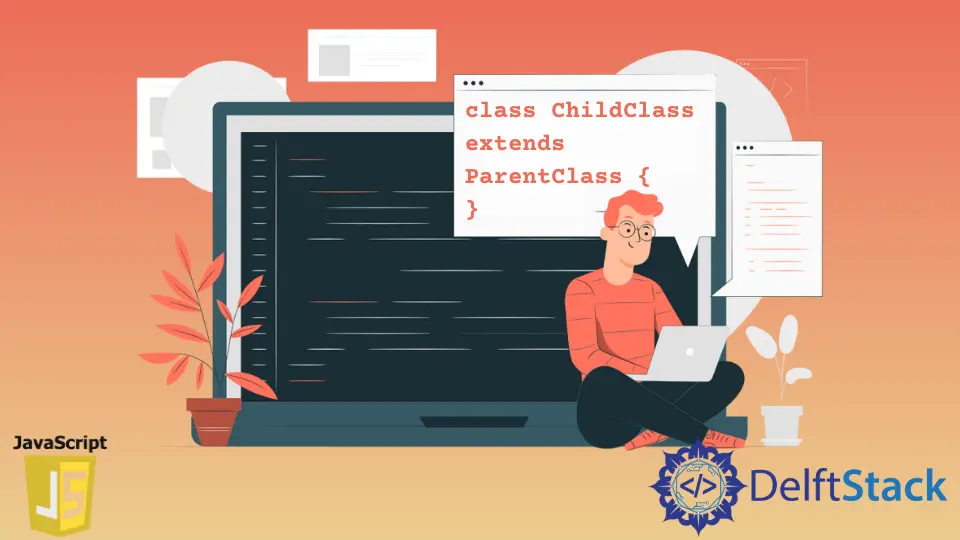
クラスは、オブジェクトを作成するためのテンプレートまたは設計図です。 コードを使用してデータをカプセル化し、そのデータを操作できます。
JS のクラスはプロトタイプに基づいていますが、ES5 のクラス セマンティクスと共有されていない構文とセマンティクスも持っています。
今日の投稿では、JavaScript でクラスを拡張する方法を学びます。
extends
キーワードを使用して JavaScript でクラスを拡張する
extends
キーワードは、メイン クラスからカスタム クラスと組み込みオブジェクトを派生させることができます。 クラス式またはクラス宣言で使用して、別の子であるクラスを作成します。
構文:
class ChildClass extends ParentClass { /* Logic goes here */
}
extends
の詳細については、こちら のドキュメントを参照してください。
たとえば、親クラスの name プロパティと数値を格納するカウンターで構成される ParentClass
があります。 ParentClass
には、親クラスの name プロパティの現在のインスタンスを表示する静的メソッドも含まれています。
ChildClass
はParentClass
のプロパティを拡張します。 親クラスを拡張することにより、子クラスはすべての親クラス プロパティにアクセスできます。
予約済みのキーワード super
は、スーパー コンストラクターの呼び出しを実行でき、親メソッドへのアクセスを提供します。
例:
class ParentClass {
constructor(counter) {
this.name = 'ParentClass';
this.counter = counter;
}
sayClassName() {
console.log('Hi, I am a ', this.name + '.');
}
}
const parent = new ParentClass(300);
parent.sayClassName();
console.log('The counter of this parent class is ' + parent.counter);
class ChildClass extends ParentClass {
constructor(counter) {
super(counter);
this.name = 'ChildClass';
}
get counter() {
return this.counter;
}
set counter(value) {
this.childCounter = value;
}
}
const child = new ChildClass(5);
child.sayClassName();
console.log('The counter of this parent class is ' + child.childCounter);
カウンターを 300 に設定する親クラスのインスタンスを作成しました。子クラスが生成されるとすぐに、カウンターが 5 に更新されます。
子クラスには counter プロパティがありません。 親クラスから継承されます。
出力:
"Hi, I am a ", "ParentClass."
"The counter of this parent class is 300"
"Hi, I am a ", "ChildClass."
"The counter of this parent class is 5"
ここ の例の完全なコードにアクセスすることもできます。
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn