JavaScript 추상 클래스
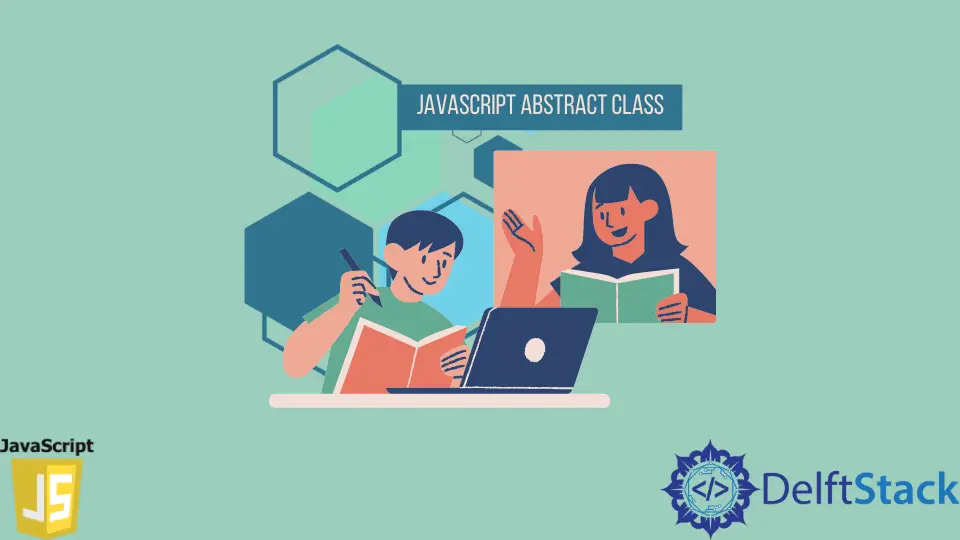
JavaScript 추상 클래스로 작업할 때 두 가지 주요 개념인 추상화 및 상속을 고려해야 합니다.
추상화를 통해 특정 세부 정보를 숨기고 개체의 필수 정보만 표시하는 보안을 가능하게 하는 반면 상속은 다른 클래스의 속성을 가져와서 부모-자식 관계를 만듭니다.
JavaScript 추상 클래스
추상 클래스는 하나 이상의 추상 메서드를 포함하지만 인스턴스화할 수 없는 클래스입니다. 추상 클래스는 인스턴스화할 수 없지만 메서드를 사용하여 확장할 수 있습니다.
또한 추상 클래스는 상속되어야 하므로 내부에 선언된 메서드를 사용하여 추상 클래스를 확장하려면 구체적인 클래스 또는 하위 클래스가 필요합니다.
추상 메서드는 선언만 가능하고 구현은 없는 메서드입니다.
먼저 추상 클래스를 정의하고 그 안에 생성자를 만들 수 있습니다. 그 다음에는 추상 클래스를 확장할 때 함수를 정의해야 합니다.
이 예제에서는 Fruit
를 추상 클래스 이름으로 정의하고 내부에 과일의 색상을 나타내는 color()
와 과일이 소비되었는지 여부를 확인하는 eat()
라는 두 가지 메서드를 생성했습니다.
여기에서 예로 든 과일은 모두 소비가 가능하기 때문에 추상 클래스 내에서 먹다
로 표시하는 방법을 구현했습니다. 그러나 각 구체적인 클래스를 정의할 때 각각에 대해 color()
메서드를 구현해야 합니다.
class Fruit {
constructor() {
if (this.constructor == Fruit) {
throw new Error('Abstract classes can\'t be instantiated.');
}
}
color() {
throw new Error('Method \'color()\' must be implemented.');
}
eat() {
console.log('eating');
}
}
구체적인 클래스로 추상 클래스 확장
추상 클래스를 만든 후에는 구체적인 클래스를 만들어야 합니다. 구체적인 클래스를 만들 때 추상 클래스의 모든 기능과 동작을 상속하도록 해야 합니다.
아래 스니펫은 추상 클래스용으로 생성된 구체적인 클래스 Apple
및 Orange
를 보여줍니다. extends
키워드를 사용하여 상속을 수행합니다.
class Apple extends Fruit {
color() {
console.log('Red');
}
}
class Orange extends Fruit {
color() {
console.log('Orange');
}
}
추상 클래스와 구체적인 클래스를 정의함으로써 추상화와 상속이 달성되었습니다.
전체 코드는 위의 코드 스니펫과 전체 코드를 결합하여 그림에 표시된 결과로 구성됩니다. 여기서 두 구체적인 클래스를 추상 클래스라고 하며 메서드에서 구현된 출력을 표시합니다.
그 외에도 생성자가 추상 클래스와 이름이 같으면 오류가 발생합니다. 그러나 구체적인 클래스로 인스턴스화하면 완벽하게 작동합니다.
암호:
// Abstract Class 'Fruit'.
class Fruit {
constructor() {
if (this.constructor == Fruit) {
throw new Error('Abstract classes can\'t be instantiated.');
}
}
// Method 'color'
color() {
throw new Error('Method \'color()\' must be implemented.');
}
// Method 'eat'
eat() {
console.log('eating');
}
}
// Concrete class 'Apple'
class Apple extends Fruit {
color() {
console.log('Red');
}
}
// Concrete class 'Orange'
class Orange extends Fruit {
color() {
console.log('Orange');
}
}
// Calling the classes
new Apple().eat();
new Orange().eat();
new Apple().color();
new Orange().color();
출력:
구체적인 클래스 Apple
도 내부에 color()
메서드를 구현했지만 banana()
는 구현하지 않았습니다. 따라서 구상 클래스 banana()
는 추상 클래스 속성만 상속합니다.
따라서 color()
메소드가 호출되면 banana
는 색상을 인쇄하는 대신 오류를 발생시킵니다.
아래 코드 스니펫은 상속 개념을 잘 보여줍니다.
// Abstract class 'Fruit'
class Fruit {
constructor() {
if (this.constructor == Fruit) {
throw new Error('Abstract classes can\'t be instantiated.');
}
}
// Method 'color'
color() {
throw new Error('Method \'color()\' must be implemented.');
}
// Method 'eat'
eat() {
console.log('eating');
}
}
// Concrete class 'Apple'
class Apple extends Fruit {
color() {
console.log('Red');
}
}
// Concrete class 'Banana'
class Banana extends Fruit {}
// Calling the classes
new Apple().eat();
new Banana().eat();
new Apple().color();
new Banana().color();
출력:
또한 인스턴스를 생성해도 오류가 발생합니다. 추상 클래스는 인스턴스화할 수 없으므로 호출할 수 없습니다.
암호:
// Abstract class 'Fruit'
class Fruit {
constructor() {
if (this.constructor == Fruit) {
throw new Error('Abstract classes can\'t be instantiated.');
}
}
// Method 'color'
color() {
throw new Error('Method \'color()\' must be implemented.');
}
// Method 'eat'
eat() {
console.log('eating');
}
}
// Concrete class 'Apple'
class Apple extends Fruit {
color() {
console.log('Red');
}
}
// Calling the class
new Fruit();
출력:
결론
전반적으로 JavaScript 및 기타 OOP 언어에서 이 추상화 기능을 사용하면 개발자와 독자가 코드를 이해하고 유지 관리할 수 있으며 코드 중복이 줄어듭니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.