JavaScript에서 여러 클래스 확장
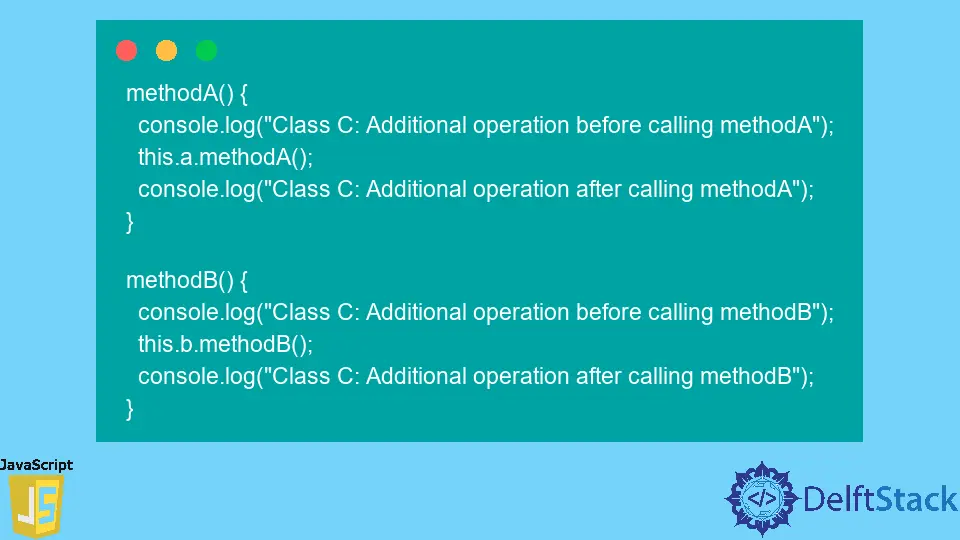
JavaScript에는 여러 클래스를 확장하기 위한 기본 제공 메커니즘이 없습니다. 그러나 JavaScript에서 다중 상속과 유사한 효과를 얻으려면 구성
및 행동 위임
이라는 기술을 사용하십시오.
JavaScript에서 여러 클래스를 확장하기 위한 컴포지션 접근 방식
구성을 사용하면 여러 클래스에서 속성과 메서드를 상속하는 대신 각 클래스의 인스턴스를 만들어 새 클래스 속성으로 저장한 다음 메서드 호출을 해당 인스턴스에 위임할 수 있습니다.
이 접근 방식을 사용하면 클래스의 동작을 더 잘 제어할 수 있다는 점에 유의해야 합니다. 필요한 경우 구성된 클래스의 속성 및 메서드에 액세스하고 메서드를 재정의할 수 있습니다.
여러 클래스를 확장하기 위한 구현
다음 예제에서 클래스 C는 속성으로 클래스 A와 클래스 B의 인스턴스를 가지며 메서드 호출을 해당 인스턴스에 위임합니다. 이렇게 하면 클래스 C는 클래스 A와 클래스 B의 메서드에 모두 액세스할 수 있습니다.
클래스 A에는 호출 시 Class A: methodA called
메시지를 콘솔에 출력하는 methodA
라는 단일 메서드가 있습니다. 클래스 B에는 호출 시 Class B: methodB called
메시지를 콘솔에 출력하는 methodB
라는 단일 메서드가 있습니다.
class A {
methodA() {
console.log('Class A: methodA called');
}
}
class B {
methodB() {
console.log('Class B: methodB called');
}
}
클래스 C는 구성 패턴을 사용하여 다중 상속과 유사한 효과를 얻습니다. 생성자는 클래스 A 및 클래스 B의 인스턴스를 생성하고 속성 a
및 b
로 각각 저장합니다.
constructor() {
this.a = new A();
this.b = new B();
}
클래스 C에는 클래스 A 및 B의 해당 메서드 호출 전후에 추가 작업을 수행하는 methodA
및 methodB
의 두 가지 메서드가 있습니다.
methodA() {
console.log('Class C: Additional operation before calling methodA');
this.a.methodA();
console.log('Class C: Additional operation after calling methodA');
}
methodB() {
console.log('Class C: Additional operation before calling methodB');
this.b.methodB();
console.log('Class C: Additional operation after calling methodB');
}
마지막 두 줄이 실행될 때 클래스 C의 인스턴스를 생성한 다음 methodA()
및 methodB().
를 호출합니다.
const c = new C();
c.methodA();
c.methodB();
소스 코드:
class A {
methodA() {
console.log('Class A: methodA called');
}
}
class B {
methodB() {
console.log('Class B: methodB called');
}
}
class C {
constructor() {
this.a = new A();
this.b = new B();
}
methodA() {
console.log("Class C: Additional operation before calling methodA");
this.a.methodA();
console.log("Class C: Additional operation after calling methodA");
}
methodB() {
console.log("Class C: Additional operation before calling methodB");
this.b.methodB();
console.log("Class C: Additional operation after calling methodB");
}
}
const c = new C();
c.methodA();
c.methodB();
출력:
JavaScript에서 여러 클래스를 확장하기 위한 행동 위임 방식
이 접근 방식의 또 다른 변형은 구성된 클래스의 인스턴스를 저장하는 대신 메서드 호출을 구성된 클래스의 속성에 직접 위임하는 동작 위임
을 사용하는 것입니다.
여러 클래스를 확장하기 위한 구현
클래스 C의 methodA
가 호출되면 먼저 클래스 C: methodA를 호출하기 전에 추가 작업
메시지를 콘솔에 출력한 다음 A.prototype.methodA.call을 사용하여 클래스 A의
methodA를 호출합니다. (this);
, 콘솔에 Class A: methodA called
를 출력합니다.
마지막으로 콘솔에 Class C: methodA 호출 후 추가 작업
메시지를 출력합니다.
마찬가지로 클래스 C의 methodB
가 호출되면 먼저 클래스 C: methodB를 호출하기 전에 추가 작업
메시지를 콘솔에 출력한 다음 B.prototype.methodB를 사용하여 클래스 B의
methodB를 호출합니다. .call(this);
, 콘솔에 Class B: methodB called
를 출력합니다.
마지막으로 콘솔에 Class C: methodB 호출 후 추가 작업
메시지를 출력합니다.
이러한 방식으로 클래스 C는 클래스 A와 클래스 B의 메서드에 모두 액세스할 수 있으며 원래 클래스를 수정하지 않고도 메서드에 몇 가지 추가 작업을 추가할 수 있습니다.
이 경우 클래스 C에는 A와 B의 인스턴스가 없습니다. 대신 call
메서드를 사용하여 클래스 C의 컨텍스트에서 A와 B의 메서드를 호출합니다.
소스 코드:
class A {
methodA() {
console.log('Class A: methodA called');
}
}
class B {
methodB() {
console.log('Class B: methodB called');
}
}
class C {
methodA() {
console.log("Class C: Additional operation before calling methodA");
A.prototype.methodA.call(this);
console.log("Class C: Additional operation after calling methodA");
}
methodB() {
console.log("Class C: Additional operation before calling methodB");
B.prototype.methodB.call(this);
console.log("Class C: Additional operation after calling methodB");
}
}
const c = new C();
c.methodA();
c.methodB();
출력:
이 접근 방식은 구성보다 메모리 효율적이지만 구성 클래스의 속성에 액세스할 수 없기 때문에 유연성이 떨어집니다.
보시다시피 두 경우 모두 클래스 C는 원래 클래스를 수정하지 않고 클래스 A와 클래스 B의 메서드에 몇 가지 추가 작업을 추가할 수 있습니다. 이렇게 하면 원래 클래스를 깨끗하게 유지하고 분리할 수 있으며 새 클래스 C에 특정 기능을 추가할 수 있습니다.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn