C# でカンマ区切りリストを作成する
-
C#
でString.Join()
メソッドを使用してカンマ区切りリストを作成する -
C#
で LINQ Aggregate メソッドを使用してカンマ区切りリストを作成する -
C#
で StringBuilder メソッドを使用してカンマ区切りリストを作成する
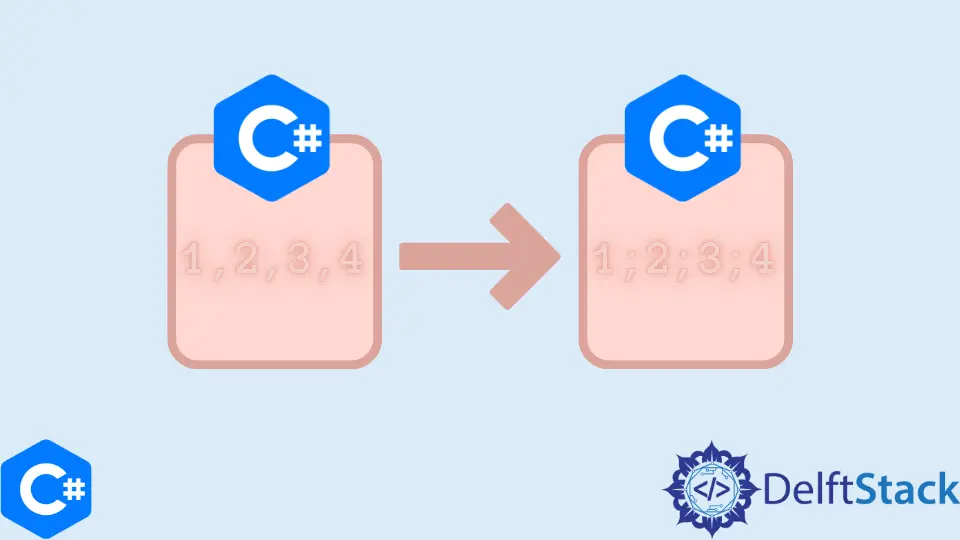
このチュートリアルでは、string.Join()
、LINQ Aggregate,
、または StringBuilder
のいずれかを使用して、IList<string
や IEnumerable<string>
などのコンテナからコンマ区切りのリストを作成する方法を示します。
C#
で String.Join()
メソッドを使用してカンマ区切りリストを作成する
コンテナの値を結合する最も簡単な方法は、string.Join()
です。string.Join()
関数は、コレクション内のすべての値を取得し、それらを定義済みの区切り文字と連結します。この方法を使用すると、リストをコンマで区切るだけでなく、他の区切り文字も作成できます。
string joined_str = string.Join(separator, source);
セパレータパラメータは文字列である必要がありますが、ソースは任意のデータ型のコレクションにすることができます。
例:
using System;
using System.Collections.Generic;
namespace StringJoin_Example {
class Program {
static void Main(string[] args) {
// Initialize the IList
IList<Int32> list_strings = new List<Int32> { 1, 2, 3 };
// Join the IList with a comma
string joined_list = string.Join(", ", list_strings);
// Print the results
Console.WriteLine("From List: " + joined_list);
// Initialize the IEnumerable
IEnumerable<string> enum_strings =
new List<string>() { "Enum Sample 1", "Enum Sample 2", "Enum Sample 3" };
// Join the IEnumerable with a semi colon
string joined_enum = string.Join("; ", enum_strings);
// Print the results
Console.WriteLine("From Enumerable: " + joined_enum);
Console.ReadLine();
}
}
}
上記の例では、整数のリストと文字列の列挙可能なリストの 2つの異なるソースを作成しました。次に、2つの異なるセパレータを使用して同じ string.Join()
メソッドを使用してそれらを結合し、結果の文字列をコンソールに出力しました。
出力:
From List: 1, 2, 3
From Enumerable: Enum Sample 1; Enum Sample 2; Enum Sample 3
C#
で LINQ Aggregate メソッドを使用してカンマ区切りリストを作成する
コレクションの値を結合するための別のオプションは、LINQ Aggregate
メソッドを使用することです。コーディングには便利ですが、特に入力が大きいほど、これは非効率的であると見なされます。このメソッドは、入力カウントが 1 未満の場合にもエラーをスローするため、これが毎回スムーズに実行されるようにするには、より多くのエラー処理が必要になる場合があります。
string joined_str = input.Aggregate((x, y) => x + ", " + y);
最初の方法と同様に、任意の文字列入力を区切り文字として使用できますが、この方法では、結果の値が入力と同じであると想定されます。たとえば、入力コレクションが整数リストの場合、この関数は機能しません。
例:
using System;
using System.Collections.Generic;
using System.Linq;
namespace LinqAggregate_Example {
class Program {
static void Main(string[] args) {
// Initialize the IList
IList<string> list_strings =
new List<string> { "List Sample 1", "List Sample 2", "List Sample 3" };
// Join the IList with a comma using LINQ Aggregate
string joined_list = list_strings.Aggregate((x, y) => x + ", " + y);
// Print the results
Console.WriteLine("From List: " + joined_list);
// Initialize the IEnumerable
IEnumerable<string> enum_strings =
new List<string>() { "Enum Sample 1", "Enum Sample 2", "Enum Sample 3" };
// Join the IEnumerable with a semi colon using LINQ Aggregate
string joined_enum = enum_strings.Aggregate((x, y) => x + "; " + y);
// Print the results
Console.WriteLine("From Enumerable: " + joined_enum);
Console.ReadLine();
}
}
}
上記の例では、LINQ Aggregate
メソッドを使用して 2つのコレクションを結合しました。変数 x
および y
は、任意の変数名に変更できます。このメソッドの背後にある考え方は、結合する値がまだある場合に、前の文字列を次の文字列に連結することです。
出力:
From List: List Sample 1, List Sample 2, List Sample 3
From Enumerable: Enum Sample 1; Enum Sample 2; Enum Sample 3
C#
で StringBuilder メソッドを使用してカンマ区切りリストを作成する
ここで説明する最後のオプションは、StringBuilder
メソッドです。他の 2つの方法と比較すると、この方法では、もう少し多くのコードを記述する必要がありますが、さらに多くのカスタマイズが可能です。関数内で、必要に応じて値を変更するためのステップをさらに追加できます。たとえば、日時のリストを渡す場合、指定された日時形式を使用しながら値を組み合わせることができます。
例:
using System;
using System.Collections.Generic;
using System.Text;
namespace StringBuilder_Example {
class Program {
static void Main(string[] args) {
// Initialize the IList
IList<string> list_strings =
new List<string> { "List Sample 1", "List Sample 2", "List Sample 3" };
// Join the IList with a comma using the StringBuilder method
string joined_list = join_collection(", ", list_strings);
// Print the results
Console.WriteLine("From List: " + joined_list);
// Initialize the IEnumerable
IEnumerable<string> enum_strings =
new List<string>() { "Enum Sample 1", "Enum Sample 2", "Enum Sample 3" };
// Join the IEnumerable with a semi colon using the StringBuilder method
string joined_enum = join_collection("; ", enum_strings);
// Print the results
Console.WriteLine("From Enumerable: " + joined_enum);
Console.ReadLine();
}
static string join_collection<T>(string separator, IEnumerable<T> values) {
// Initialize StringBuilder
StringBuilder sb = new StringBuilder();
// Intialize the item counter
int ctr = 0;
foreach (T item in values) {
string sep = "";
if (ctr > 0) {
// If the first item from the value collection has already been added, use the specified
// separator This is to avoid printing the separator as the first value of the string
sep = separator;
}
// Append the separator and the value as a string
sb.Append(sep);
sb.Append(item.ToString());
ctr++;
}
// Return the built string
return sb.ToString();
}
}
}
上記の例では、文字列区切り文字と列挙可能なコレクションを受け入れるカスタム関数 join_collection
を使用してコレクションを結合しました。次に、StringBuilder
を使用して値を結合し、セパレータとコレクションの値を追加します。
出力:
From List: List Sample 1, List Sample 2, List Sample 3
From Enumerable: Enum Sample 1; Enum Sample 2; Enum Sample 3