在 C# 中创建逗号分隔列表
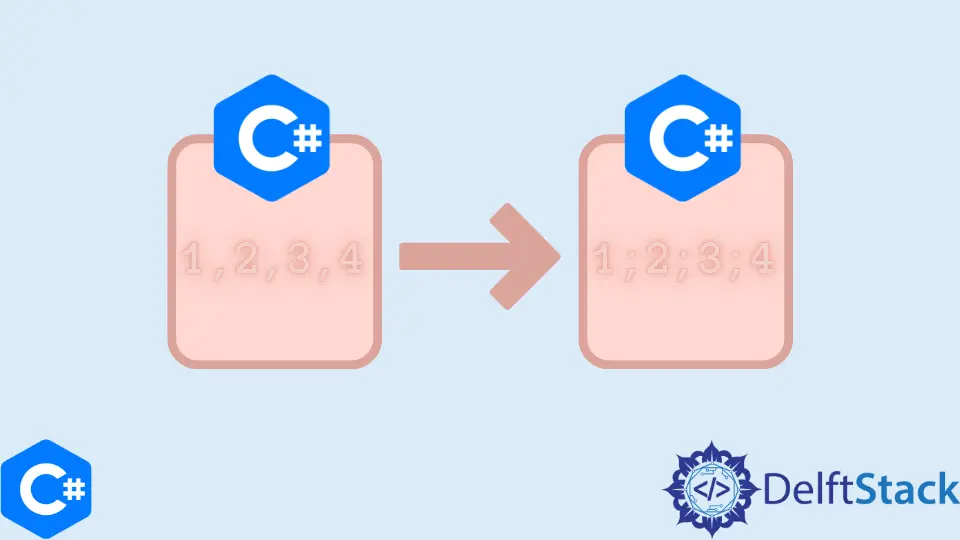
本教程将演示如何使用 string.Join()
、LINQ Aggregate,
或 StringBuilder
从诸如 IList<string
或 IEnumerable<string>
之类的容器创建逗号分隔的列表。
使用 String.Join()
方法在 C#
中创建逗号分隔列表
连接容器值的最简单方法是 string.Join()
。string.Join()
函数获取集合中的所有值,并将它们与任何定义的分隔符连接起来。使用此方法,你不仅可以制作用逗号分隔的列表,还可以制作任何其他分隔符。
string joined_str = string.Join(separator, source);
分隔符参数必须是字符串,而源可以是任何数据类型的集合。
例子:
using System;
using System.Collections.Generic;
namespace StringJoin_Example {
class Program {
static void Main(string[] args) {
// Initialize the IList
IList<Int32> list_strings = new List<Int32> { 1, 2, 3 };
// Join the IList with a comma
string joined_list = string.Join(", ", list_strings);
// Print the results
Console.WriteLine("From List: " + joined_list);
// Initialize the IEnumerable
IEnumerable<string> enum_strings =
new List<string>() { "Enum Sample 1", "Enum Sample 2", "Enum Sample 3" };
// Join the IEnumerable with a semi colon
string joined_enum = string.Join("; ", enum_strings);
// Print the results
Console.WriteLine("From Enumerable: " + joined_enum);
Console.ReadLine();
}
}
}
在上面的示例中,我们创建了两个不同的源:整数列表和可枚举的字符串列表。然后我们使用相同的 string.Join()
方法和两个不同的分隔符将它们连接起来,然后将生成的字符串打印到控制台。
输出:
From List: 1, 2, 3
From Enumerable: Enum Sample 1; Enum Sample 2; Enum Sample 3
使用 LINQ 聚合方法在 C#
中创建逗号分隔列表
加入集合值的另一个选项是使用 LINQ Aggregate
方法。虽然编码方便,但这被认为是低效的,尤其是输入越大。如果输入计数小于 1,此方法也会抛出错误,因此可能需要更多的错误处理以确保每次都能顺利运行。
string joined_str = input.Aggregate((x, y) => x + ", " + y);
与第一种方法一样,你可以使用任何字符串输入作为分隔符,但此方法将期望结果值与其输入相同。例如,如果输入集合是整数列表,则此函数将不起作用。
例子:
using System;
using System.Collections.Generic;
using System.Linq;
namespace LinqAggregate_Example {
class Program {
static void Main(string[] args) {
// Initialize the IList
IList<string> list_strings =
new List<string> { "List Sample 1", "List Sample 2", "List Sample 3" };
// Join the IList with a comma using LINQ Aggregate
string joined_list = list_strings.Aggregate((x, y) => x + ", " + y);
// Print the results
Console.WriteLine("From List: " + joined_list);
// Initialize the IEnumerable
IEnumerable<string> enum_strings =
new List<string>() { "Enum Sample 1", "Enum Sample 2", "Enum Sample 3" };
// Join the IEnumerable with a semi colon using LINQ Aggregate
string joined_enum = enum_strings.Aggregate((x, y) => x + "; " + y);
// Print the results
Console.WriteLine("From Enumerable: " + joined_enum);
Console.ReadLine();
}
}
}
在上面的示例中,我们使用 LINQ Aggregate
方法连接了两个集合。变量 x
和 y
可以更改为你喜欢的任何变量名称。此方法背后的想法是,如果仍有要连接的值,它将前一个字符串连接到下一个字符串。
输出:
From List: List Sample 1, List Sample 2, List Sample 3
From Enumerable: Enum Sample 1; Enum Sample 2; Enum Sample 3
在 C#
中使用 StringBuilder 方法创建逗号分隔列表
我们将讨论的最后一个选项是 StringBuilder
方法。与其他两种方法相比,这种方法需要编写更多代码,但允许进行更多自定义。在函数中,你可以添加更多步骤来修改你喜欢的值。例如,如果你传递日期时间列表,则可以在使用指定的 DateTime 格式时组合这些值。
例子:
using System;
using System.Collections.Generic;
using System.Text;
namespace StringBuilder_Example {
class Program {
static void Main(string[] args) {
// Initialize the IList
IList<string> list_strings =
new List<string> { "List Sample 1", "List Sample 2", "List Sample 3" };
// Join the IList with a comma using the StringBuilder method
string joined_list = join_collection(", ", list_strings);
// Print the results
Console.WriteLine("From List: " + joined_list);
// Initialize the IEnumerable
IEnumerable<string> enum_strings =
new List<string>() { "Enum Sample 1", "Enum Sample 2", "Enum Sample 3" };
// Join the IEnumerable with a semi colon using the StringBuilder method
string joined_enum = join_collection("; ", enum_strings);
// Print the results
Console.WriteLine("From Enumerable: " + joined_enum);
Console.ReadLine();
}
static string join_collection<T>(string separator, IEnumerable<T> values) {
// Initialize StringBuilder
StringBuilder sb = new StringBuilder();
// Intialize the item counter
int ctr = 0;
foreach (T item in values) {
string sep = "";
if (ctr > 0) {
// If the first item from the value collection has already been added, use the specified
// separator This is to avoid printing the separator as the first value of the string
sep = separator;
}
// Append the separator and the value as a string
sb.Append(sep);
sb.Append(item.ToString());
ctr++;
}
// Return the built string
return sb.ToString();
}
}
}
在上面的示例中,我们使用自定义函数 join_collection
加入集合,该函数接受字符串分隔符和可枚举集合。然后使用 StringBuilder
将这些值连接在一起,并附加分隔符和集合值。
输出:
From List: List Sample 1, List Sample 2, List Sample 3
From Enumerable: Enum Sample 1; Enum Sample 2; Enum Sample 3