Angular フィルターを使用してデータをグループ化する
-
filter
関数を使用して Angular でデータをグループ化する - ピュアHTML を使用して Angular でデータをグループ化する
-
ngFor
を使用して Angular でデータをグループ化する - まとめ
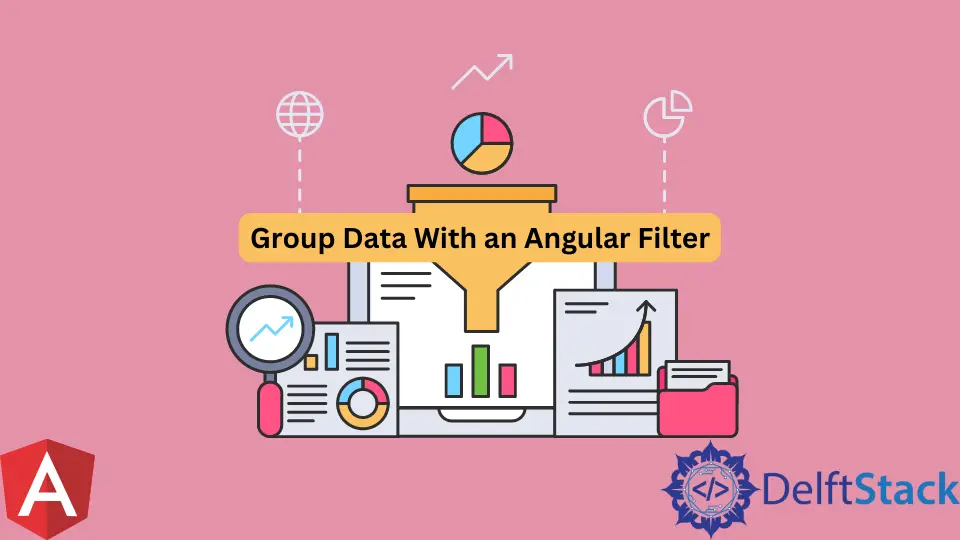
Web ページはさまざまな理由で使用されます。 問題を解決するために設計されたウェブサイトがあります。 一部は教育目的で設計されています。
このような場合、これらの Web サイトは分類されたプレゼンテーションでアイテムを表示する必要がある場合があります。 クラスに存在するアイテムは、国、州、地区などのクラスでデータを提示するなど、各アイテムがどこに属しているかを示すために必要です。
Angular フレームワークを使用してアイテムをグループ化するさまざまな方法を見ていきます。
filter
関数を使用して Angular でデータをグループ化する
この最初の方法は、データをグループに分けて表示し、フィルター機能を備えた検索エンジンを備えています。
まず、新しいプロジェクト フォルダーを作成します。次に、プロジェクト フォルダーの src>>app
フォルダー内にさらに 2つのファイルを作成する必要があります。
最初のファイルは cars.ts
という名前になります。 これには、グループ化するデータが配列の形式で含まれます。 2 番目のファイルは filter.pipe.ts
という名前になります。 これは、検索フィルターのパイプ関数を作成する場所です。
プロジェクト フォルダの app.component.html
ファイルに移動し、これらのコードを入力します。
コード スニペット - app.component.html
:
<div class="container">
<h2 class="py-4">Cars</h2>
<div class="form-group mb-4">
<input class="form-control" type="text" [(ngModel)]="searchText" placeholder="Search">
</div>
<table class="table" *ngIf="(cars | filter: searchText).length > 0; else noResults">
<colgroup>
<col width="5%">
<col width="*">
<col width="35%">
<col width="15%">
</colgroup>
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Brand</th>
<th scope="col">Model</th>
<th scope="col">Year</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let car of cars | filter: searchText; let i = index">
<th scope="row">{{i + 1}}</th>
<td>{{car.brand}}</td>
<td>{{car.model}}</td>
<td>{{car.year}}</td>
</tr>
</tbody>
</table>
<ng-template #noResults>
<p>No results found for "{{searchText}}".</p>
</ng-template>
</div>
ここでは、ページの構造とデータのグループ化形式を作成し、見出しに *ngFor
関数を宣言して検索フィルターを有効にしました。
次に、app.component.ts
ファイルに移動して、これらのいくつかのコードを入力します。
コード スニペット - app.component.ts
:
import { Component } from '@angular/core';
import { CARS } from './cars';
interface Car {
brand: string;
model: string;
year: number;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'filterone';
cars: Car[] = CARS;
searchText: string;
}
ここで行ったことは、グループ化するデータを宣言したことだけです。 brand
、model
、および検索フィールド内の入力の型については、車のモデルの year
の型番号を宣言している間、文字列型として宣言しています。
次に、グループ化するデータを挿入するために、前に作成した car.ts
ファイルに移動する必要があります。 これらのコードを入力します。
コード スニペット - cars.ts
:
export const CARS = [
{
brand: 'Audi',
model: 'A4',
year: 2018
}, {
brand: 'Audi',
model: 'A5 Sportback',
year: 2021
}, {
brand: 'BMW',
model: '3 Series',
year: 2015
}, {
brand: 'BMW',
model: '4 Series',
year: 2017
}, {
brand: 'Mercedes-Benz',
model: 'C Klasse',
year: 2016
}
];
ここでは、配列の形式でグループ化したいデータを入力しました。それぞれは、app.component.ts
ファイルで宣言したデータ型です。
次に、filter.pipe.ts
ファイルをナビゲートして、これらのコードを入力します。
コード スニペット - filter.pipe.ts
:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'filter'
})
export class FilterPipe implements PipeTransform {
transform(items: any[], searchText: string): any[] {
if (!items) return [];
if (!searchText) return items;
return items.filter(item => {
return Object.keys(item).some(key => {
return String(item[key]).toLowerCase().includes(searchText.toLowerCase());
});
});
}
}
pipe
関数が行うことは、グループ化されたデータの変更を検出し、変更が発生した後に新しい値を返すことです。
そのため、transform()
関数内で変更可能な項目を宣言し、if
ステートメントを使用して、宣言された項目で変更が発生すると、フィルタリングされた項目を返すことを Angular に伝えます。 検索バーに要素を入力すると、入力した単語に関連するアイテムがページに表示されます。
最後に、app.module.ts
ファイル内でいくつかの作業を行います。
コード スニペット - app.module.ts
:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { FilterPipe } from './filter.pipe';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [
AppComponent,
FilterPipe
],
imports: [
BrowserModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
ここで、アプリケーションを機能させるモジュールをインポートします。 また、filter.pipe.ts
から Filterpipe
コンポーネントを宣言しました。
出力:
ピュアHTML を使用して Angular でデータをグループ化する
グループ化されたデータの構造を見ると、純粋な HTML を使用して簡単にコード化できます。これをこの例で行います。
app.component.html
ファイル内で、すべてのコーディングを行います。
コード スニペット - app.component.html
:
<ol class="list-group list-group-light list-group-numbered">
<li class="list-group-item d-flex justify-content-between align-items-start">
<div class="ms-2 me-auto">
<div class="fw-bold"><h4>Fruits</h4></div>
<ol>Pineapple</ol>
<ol>apple</ol>
<ol>Pine</ol>
</div>
</li>
<li class="list-group-item d-flex justify-content-between align-items-start">
<div class="ms-2 me-auto">
<div class="fw-bold"><h4>Cars</h4></div>
<ol>BMW</ol>
<ol>Benz</ol>
<ol>Audi</ol>
</div>
</li>
<li class="list-group-item d-flex justify-content-between align-items-start">
<div class="ms-2 me-auto">
<div class="fw-bold"><h4>Countries</h4></div>
<ol>US</ol>
<ol>Italy</ol>
<ol>France</ol>
</div>
</li>
</ol>
各グループのヘッダーを h4
タグ内で宣言して、分類しているアイテムよりも大きく見えるようにしました。 次に、順序付きリスト タグ内で各項目を宣言しました。
出力:
ngFor
を使用して Angular でデータをグループ化する
ngFor
ディレクティブは、配列の形式で表示されるローカル データを利用できるようにする Angular の組み込み関数です。 表示するデータをグループ化されたデータの形式で編成します。
新しいプロジェクト フォルダーを作成し、app.component.ts
ファイルに移動して、これらのコードを入力します。
コード スニペット - app.component.ts
:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'filterone';
listofStatesCity : any[]=
[
{'State' : 'Karnataka', 'City':[{'Name': 'Bengaluru'}, {'Name': 'Mangaluru'}]},
{'State' : 'Tamil Nadu', 'City':[{'Name': 'Chennai'}, {'Name': 'Vellore'}]},
{'State' : 'Jharkhand', 'City':[{'Name': 'Ranchi'}, {'Name': 'Bokaro'}]}
]
}
State
と City
の 2つのクラスにデータをグループ化しています。 各グループの下に州と都市の名前を宣言しました。
次に、app.component.html
に移動して、配列データの構造を作成します。
コード スニペット - app.component.html
:
<ul *ngFor = "let state of listofStatesCity">
<li>{{state.State}}</li>
<ul *ngFor = "let city of state.City">
<li>
{{city.Name}}
</li>
</ul>
各グループに対して ngFor
ディレクティブを宣言したため、データが Web ページにレンダリングされると、データは State
と City
に分類されます。
出力:
まとめ
Angular フレームワーク内でさまざまな方法を使用してデータをグループ化できます。 データをグループ化する理由によって、採用するアプローチが決まる可能性があります。特に、検索フィルター オプションを作成できる pipe
関数を使用する場合はそうです。
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn