Angular でのオブジェクト プロパティによるフィルタリング
- Angular の任意のオブジェクト プロパティでフィルタリングする
- Angular での特定のオブジェクト プロパティによるフィルタリング
- Angular でパイプを使用してオブジェクト プロパティでフィルター処理する
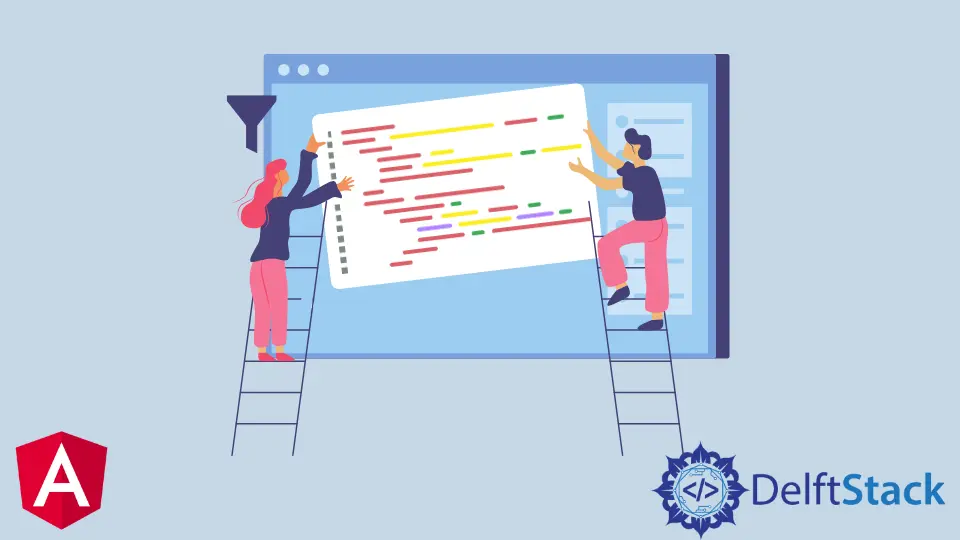
Web ページのフィルター機能を使用すると、アイテムが長いリストの中にあるかどうかを評価したい場合など、ユーザーは検索をすばやく絞り込むことができます。 また、フィルター機能を使用して、車のリストなどのリストを除外することもできます。
プロパティでオブジェクトをフィルタリングするには、文字列や数値などでリストをフィルタリングできます。この記事では、Web ページ内でフィルタを実行するさまざまな方法を見ていきます。
Angular の任意のオブジェクト プロパティでフィルタリングする
この例では、文字列や数値など、任意の条件でフィルター処理できる Web アプリを作成します。 そこで、新しいプロジェクト フォルダーを作成し、index.html
ファイルに移動してコードを記述します。
コード スニペット - index.html
:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Example Filter</title>
<script src="//code.angularjs.org/snapshot/angular.min.js"></script>
</head>
<body ng-app="">
<div ng-init="friends = [{name:'John', phone:'555-1276'},
{name:'Mary', phone:'800-BIG-MARY'},
{name:'Mike', phone:'555-4321'},
{name:'Adam', phone:'555-5678'},
{name:'Julie', phone:'555-8765'},
{name:'Juliette', phone:'555-5678'}]"></div>
<label>Search: <input ng-model="searchText"></label>
<table id="searchTextResults">
<tr><th>Name</th><th>Phone</th></tr>
<tr ng-repeat="friend in friends | filter:searchText">
<td>{{friend.name}}</td>
<td>{{friend.phone}}</td>
</tr>
</table>
<hr>
</table>
</body>
</html>
オブジェクトの配列をテーブルに整理し、オブジェクトのフィルタリングに使用する入力バーも提供します。 次に、filter.js
という名前の新しいファイルを作成する必要があります。 これは、アプリの機能を追加する場所です。
コード スニペット - filter.js
:
var expectFriendNames = function(expectedNames, key) {
element.all(by.repeater(key + ' in friends').column(key + '.name')).then(function(arr) {
arr.forEach(function(wd, i) {
expect(wd.getText()).toMatch(expectedNames[i]);
});
});
};
it('should search across all fields when filtering with a string', function() {
var searchText = element(by.model('searchText'));
searchText.clear();
searchText.sendKeys('m');
expectFriendNames(['Mary', 'Mike', 'Adam'], 'friend');
searchText.clear();
searchText.sendKeys('76');
expectFriendNames(['John', 'Julie'], 'friend');
});
そのため、検索入力用の関数を作成し、searchText 変数内でモデルを呼び出しました。 以下の出力を参照してください。
Angular での特定のオブジェクト プロパティによるフィルタリング
今回は、特定のプロパティでフィルタリングできるアプリ、文字列でフィルタリングするアプリ、文字列のみが機能するアプリ、数値でフィルタリングするアプリ、数値のみが機能するアプリを作成したいと考えています。 文字列と数値の両方で検索できます。
新しい angular プロジェクトを作成し、index.html
ファイルに移動して、ページの構造を作成するコードを追加します。 任意、番号のみ、文字列のみの検索バーが含まれます。
コード スニペット - index.html
:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Example - example-filter-filter-production</title>
<script src="//code.angularjs.org/snapshot/angular.min.js"></script>
</head>
<body ng-app="">
<div ng-init="friends = [{name:'John', phone:'555-1276'},
{name:'Mary', phone:'555-2578'},
{name:'Mike', phone:'555-4321'},
{name:'Adam', phone:'555-5678'},
{name:'Julie', phone:'555-8765'},
{name:'Juliette', phone:'555-5678'}]">
</div>
<hr>
<label>Any: <input ng-model="search.$"></label> <br>
<label>Name only <input ng-model="search.name"></label><br>
<label>Phone only <input ng-model="search.phone"></label><br>
<table id="searchObjResults">
<tr><th>Name</th><th>Phone</th></tr>
<tr ng-repeat="friendObj in friends | filter:search:strict">
<td>{{friendObj.name}}</td>
<td>{{friendObj.phone}}</td>
</tr>
</table>
</body>
</html>
この時点で、上で定義した構造の Web ページが表示されるはずです。 次に、アプリの機能を設計します。
filter.js
という名前の新しいファイルを作成し、これらのコードを入力します。
コード スニペット - filter.js
:
var expectFriendNames = function(expectedNames, key) {
it('should search in specific fields when filtering with a predicate object', function() {
var searchAny = element(by.model('search.$'));
searchAny.clear();
searchAny.sendKeys('i');
expectFriendNames(['Mary', 'Mike', 'Julie', 'Juliette'], 'friendObj');
});
it('should use a equal comparison when comparator is true', function() {
var searchName = element(by.model('search.name'));
var strict = element(by.model('strict'));
searchName.clear();
searchName.sendKeys('Julie');
strict.click();
expectFriendNames(['Julie'], 'friendObj');
});
searchAny
変数を使用して、ユーザーが文字列または数値を使用してフィルタリングできることを示します。
searchName
変数を宣言した後、strict
変数も宣言しました。これにより、数値の入力フィールドで文字列を使用してフィルター処理した場合、データが表示されなくなります。 文字列の入力フィールドの数値でフィルタリングしようとすると、同じことが起こります。
以下の出力を参照してください。
Angular でパイプを使用してオブジェクト プロパティでフィルター処理する
パイプは、2つのコマンドまたはプロセスを実行するのに理想的です。これは、ここで行っていることにとって理想的です。 たとえば、1つのプロセスであるフィルター処理を行い、2 番目のプロセスであるフィルターの結果を返すようにします。
そのため、新しい angular プロジェクトを作成し、フィルタリングするアイテムの詳細を保持する新しいファイルを作成します。 ファイルにcars.ts
という名前を付け、次のコードを入力します。
コード スニペット - cars.ts
:
export const CARS = [
{
brand: 'Audi',
model: 'A4',
year: 2018
}, {
brand: 'Audi',
model: 'A5 Sportback',
year: 2021
}, {
brand: 'BMW',
model: '3 Series',
year: 2015
}, {
brand: 'BMW',
model: '4 Series',
year: 2017
}, {
brand: 'Mercedes-Benz',
model: 'C Klasse',
year: 2016
}
];
次に、フィルターの入力フィールドを含む cars.ts
のデータをテーブルに整理するファイル構造を作成します。 そのためのコードを app.component.html
ファイル内に入力します。
コード スニペット - app.component.html
<div class="container">
<h2 class="py-4">Cars</h2>
<div class="form-group mb-4">
<input class="form-control" type="text" [(ngModel)]="searchText" placeholder="Search">
</div>
<table class="table" *ngIf="(cars | filter: searchText).length > 0; else noResults">
<colgroup>
<col width="5%">
<col width="*">
<col width="35%">
<col width="15%">
</colgroup>
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Brand</th>
<th scope="col">Model</th>
<th scope="col">Year</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let car of cars | filter: searchText; let i = index">
<th scope="row">{{i + 1}}</th>
<td>{{car.brand}}</td>
<td>{{car.model}}</td>
<td>{{car.year}}</td>
</tr>
</tbody>
</table>
<ng-template #noResults>
<p>No results found for "{{searchText}}".</p>
</ng-template>
</div>
次に、アプリの機能に取り組みたいと思います。 新しいファイルを作成し、filter.pipe.ts
という名前を付けて、次のコードを含めます。
コード スニペット - filter.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'filter'
})
export class FilterPipe implements PipeTransform {
transform(items: any[], searchText: string): any[] {
if (!items) return [];
if (!searchText) return items;
return items.filter(item => {
return Object.keys(item).some(key => {
return String(item[key]).toLowerCase().includes(searchText.toLowerCase());
});
});
}
}
Pipe
と PipeTransform
を angular コアからインポートしたため、アイテムをフィルタリングすると、データが変換され、フィルタリングされた結果が返されます。 次に、app.component.ts
ファイルにアクセスして、機能を追加するためのコードを少し追加する必要があります。
コード スニペット - app.component.ts
:
import { Component } from '@angular/core';
import { CARS } from './cars';
interface Car {
brand: string;
model: string;
year: number;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'filthree';
cars: Car[] = CARS;
searchText: string;
}
cars.ts
の配列内の項目のデータ型を宣言しました。 最後に、必要なモジュールを app.module.ts
ファイルにインポートする必要があります。
コード スニペット - app.module.ts
:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { FilterPipe } from './filter.pipe';
@NgModule({
imports: [ BrowserModule, FormsModule ],
declarations: [ AppComponent, FilterPipe ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
すべての手順が完了すると、次の出力が表示されます。
したがって、Angular フィルターを使用したアイテムの並べ替えは、必要なものをすばやく検索するのに役立つため、Web サイトのユーザーを便利にする機能の 1つです。
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn