Angular 필터로 데이터 그룹화
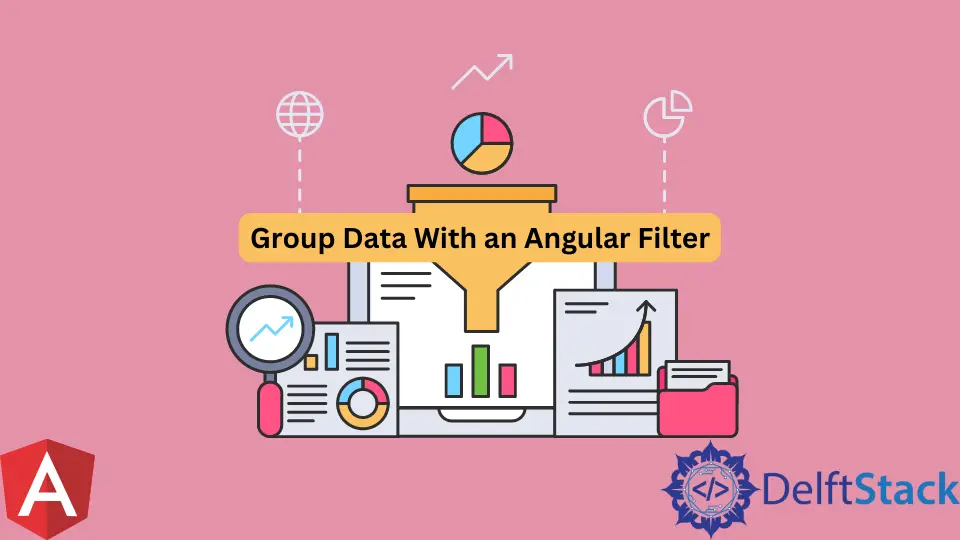
웹 페이지는 여러 가지 이유로 사용됩니다. 문제를 해결하도록 설계된 웹사이트가 있습니다. 일부는 교육 목적으로 설계되었습니다.
이러한 경우 이러한 웹 사이트는 항목을 분류된 프레젠테이션으로 표시해야 할 수 있습니다. 클래스에 있는 항목은 국가, 주, 지구 등의 클래스에 데이터를 표시하는 것과 같이 각 항목이 속한 위치를 표시하는 데 필요합니다.
Angular 프레임워크를 사용하여 항목을 그룹화하는 다양한 방법을 살펴보겠습니다.
filter
기능을 사용하여 Angular로 데이터 그룹화
이 첫 번째 방법은 데이터를 그룹으로 표시하고 필터 기능이 있는 검색 엔진을 제공합니다.
새 프로젝트 폴더를 생성하는 것으로 시작한 다음 프로젝트 폴더의 src>>app
폴더 내에 두 개의 파일을 더 생성해야 합니다.
첫 번째 파일의 이름은 cars.ts
입니다. 여기에는 배열 형태로 그룹화하려는 데이터가 포함됩니다. 두 번째 파일의 이름은 filter.pipe.ts
입니다. 여기에서 검색 필터에 대한 파이프 함수를 만듭니다.
이제 프로젝트 폴더의 app.component.html
파일로 이동하여 이 코드를 입력합니다.
코드 스니펫 - app.component.html
:
<div class="container">
<h2 class="py-4">Cars</h2>
<div class="form-group mb-4">
<input class="form-control" type="text" [(ngModel)]="searchText" placeholder="Search">
</div>
<table class="table" *ngIf="(cars | filter: searchText).length > 0; else noResults">
<colgroup>
<col width="5%">
<col width="*">
<col width="35%">
<col width="15%">
</colgroup>
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Brand</th>
<th scope="col">Model</th>
<th scope="col">Year</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let car of cars | filter: searchText; let i = index">
<th scope="row">{{i + 1}}</th>
<td>{{car.brand}}</td>
<td>{{car.model}}</td>
<td>{{car.year}}</td>
</tr>
</tbody>
</table>
<ng-template #noResults>
<p>No results found for "{{searchText}}".</p>
</ng-template>
</div>
여기에서 페이지의 구조와 데이터의 그룹화 형식을 만든 다음 제목에 *ngFor
함수를 선언하여 검색 필터를 활성화했습니다.
다음으로 app.component.ts
파일로 이동하여 이러한 몇 가지 코드를 입력합니다.
코드 스니펫 - app.component.ts
:
import { Component } from '@angular/core';
import { CARS } from './cars';
interface Car {
brand: string;
model: string;
year: number;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'filterone';
cars: Car[] = CARS;
searchText: string;
}
여기서 우리가 한 일은 그룹화하려는 데이터를 선언하는 것뿐입니다. 브랜드
, 모델
및 검색 필드 내 입력 유형의 경우 문자열 유형으로 선언하고 자동차 모델의 연도
에 대한 유형 번호를 선언했습니다.
이제 그룹화하려는 데이터를 삽입하기 위해 이전에 생성한 car.ts
파일로 이동해야 합니다. 이 코드를 입력합니다.
코드 스니펫 - cars.ts
:
export const CARS = [
{
brand: 'Audi',
model: 'A4',
year: 2018
}, {
brand: 'Audi',
model: 'A5 Sportback',
year: 2021
}, {
brand: 'BMW',
model: '3 Series',
year: 2015
}, {
brand: 'BMW',
model: '4 Series',
year: 2017
}, {
brand: 'Mercedes-Benz',
model: 'C Klasse',
year: 2016
}
];
여기에 배열 형식으로 그룹화하려는 데이터를 입력했으며 각각은 app.component.ts
파일에서 선언한 데이터 유형에 있습니다.
다음으로 filter.pipe.ts
파일을 탐색하고 이 코드를 입력합니다.
코드 스니펫 - filter.pipe.ts
:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'filter'
})
export class FilterPipe implements PipeTransform {
transform(items: any[], searchText: string): any[] {
if (!items) return [];
if (!searchText) return items;
return items.filter(item => {
return Object.keys(item).some(key => {
return String(item[key]).toLowerCase().includes(searchText.toLowerCase());
});
});
}
}
파이프
기능이 하는 일은 그룹화된 데이터의 변경을 감지하고 변경이 발생한 후 새 값을 반환하는 것입니다.
따라서 transform()
함수 내에서 변경할 수 있는 항목을 선언한 다음 if
문을 사용하여 선언된 항목에서 변경이 발생하면 필터링된 항목을 반환하도록 Angular에 알립니다. 검색창에 요소를 입력하면 입력한 단어와 관련된 항목을 반환하는 페이지가 표시됩니다.
마지막으로 app.module.ts
파일 내에서 몇 가지 작업을 수행합니다.
코드 스니펫 - app.module.ts
:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { FilterPipe } from './filter.pipe';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [
AppComponent,
FilterPipe
],
imports: [
BrowserModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
여기에서 애플리케이션이 작동하도록 허용하는 모듈을 가져옵니다. 또한 filter.pipe.ts
에서 Filterpipe
구성 요소를 선언했습니다.
출력:
순수 HTML을 사용하여 Angular로 데이터 그룹화
그룹화된 데이터의 구조를 볼 때 순수 HTML을 사용하여 쉽게 코드화할 수 있으며, 이 예에서 수행할 작업입니다.
app.component.html
파일 내부에서 모든 코딩을 수행할 것입니다.
코드 스니펫 - app.component.html
:
<ol class="list-group list-group-light list-group-numbered">
<li class="list-group-item d-flex justify-content-between align-items-start">
<div class="ms-2 me-auto">
<div class="fw-bold"><h4>Fruits</h4></div>
<ol>Pineapple</ol>
<ol>apple</ol>
<ol>Pine</ol>
</div>
</li>
<li class="list-group-item d-flex justify-content-between align-items-start">
<div class="ms-2 me-auto">
<div class="fw-bold"><h4>Cars</h4></div>
<ol>BMW</ol>
<ol>Benz</ol>
<ol>Audi</ol>
</div>
</li>
<li class="list-group-item d-flex justify-content-between align-items-start">
<div class="ms-2 me-auto">
<div class="fw-bold"><h4>Countries</h4></div>
<ol>US</ol>
<ol>Italy</ol>
<ol>France</ol>
</div>
</li>
</ol>
분류하는 항목보다 더 크게 보이도록 h4
태그 안에 각 그룹의 헤더를 선언했습니다. 그런 다음 정렬된 목록 태그 안에 각 항목을 선언했습니다.
출력:
ngFor
를 사용하여 Angular로 데이터 그룹화
ngFor
지시문은 배열 형태로 표시되는 로컬 데이터를 활용할 수 있게 해주는 Angular의 내장 함수입니다. 표시할 데이터를 그룹화된 데이터 형태로 정리합니다.
새 프로젝트 폴더를 만들고 app.component.ts
파일로 이동한 다음 이 코드를 입력합니다.
코드 스니펫 - app.component.ts
:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'filterone';
listofStatesCity : any[]=
[
{'State' : 'Karnataka', 'City':[{'Name': 'Bengaluru'}, {'Name': 'Mangaluru'}]},
{'State' : 'Tamil Nadu', 'City':[{'Name': 'Chennai'}, {'Name': 'Vellore'}]},
{'State' : 'Jharkhand', 'City':[{'Name': 'Ranchi'}, {'Name': 'Bokaro'}]}
]
}
데이터를 State
및 City
의 두 클래스로 그룹화합니다. 우리는 각 그룹 아래에 있는 주와 도시의 이름을 선언했습니다.
그런 다음 app.component.html
로 이동하여 배열 데이터의 구조를 생성합니다.
코드 스니펫 - app.component.html
:
<ul *ngFor = "let state of listofStatesCity">
<li>{{state.State}}</li>
<ul *ngFor = "let city of state.City">
<li>
{{city.Name}}
</li>
</ul>
그룹별로 ngFor
지시어를 선언하여 데이터가 웹 페이지에 렌더링될 때 State
와 City
로 분류됩니다.
출력:
결론
Angular 프레임워크 내에서 다양한 방법을 사용하여 데이터를 그룹화할 수 있습니다. 데이터를 그룹화하는 이유는 특히 검색 필터 옵션을 만들 수 있는 pipe
기능을 사용하여 채택할 접근 방식을 결정할 수 있습니다.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn