Angular で現在の日付を取得する
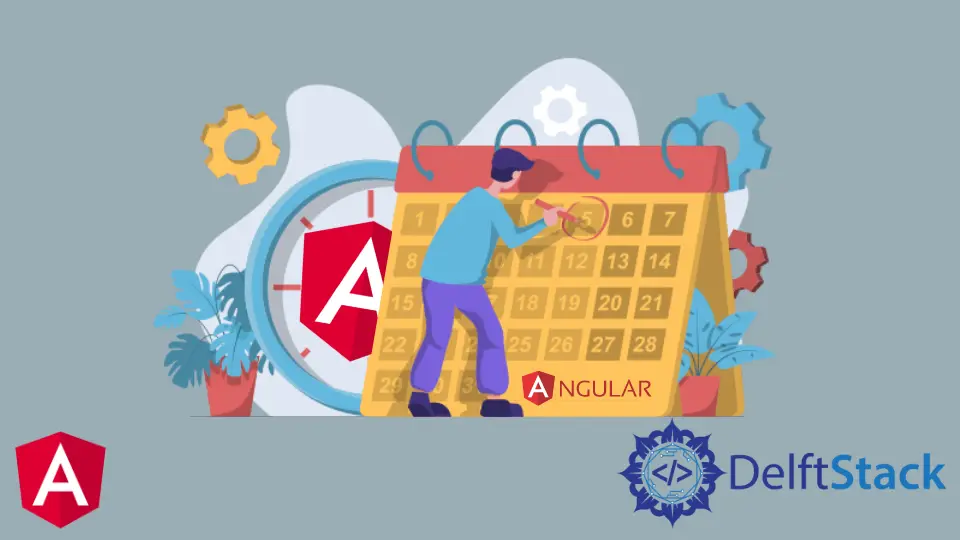
例を使用して、Angular アプリケーションで現在の日付を取得する簡単な方法を紹介します。 また、Angular で日付形式を変更する方法にも取り組みます。
Angular で現在の日付を取得する
date
は、アプリケーションで日付に基づいてすべてのレコードを保存または削除するために必要な、最も一般的で重要な要素の 1つです。 日付パラメーターに基づいてデータを管理および表示するのに役立ちます。
カスタム ユーザー インターフェイスでは、日付は日、月、年で構成されます。 理解を助けるために、現在の日付を簡単に取得する方法の例を次に示します。
次のコマンドを使用して、Angular で新しいアプリケーションを作成します。
# angular
ng new my-app
Angular でアプリケーションを作成したら、アプリケーション ディレクトリに移動します。
# angular
cd my-app
アプリを実行して、すべての依存関係が正しくインストールされているかどうかを確認します。
# angular
ng serve --open
app.component.ts
ファイルで date()
メソッドを使用して現在の日付を取得します。
# Angular
today = new Date();
この 1 行のコードは、出力に示されている現在の日付を取得します。
出力:
この 1 行のコードを使用して、現在の日付をこの形式で取得できます。 必要に応じて日付形式を変更してみましょう。
日付を保存し、そのフォーマットを変更する関数 changeFormat
を作成します。 したがって、使用するたびにフォーマットは同じままですが、他のフォーマットに変更したい場合は、関数を使用して簡単に変更したり、必要なフォーマットで表示したりできます。
以下に示すように、DatePipe
を app.component.ts
にインポートしてみましょう。
# Angular
import { DatePipe } from '@angular/common';
関数によってフォーマットが変更された後、日付の値を保存するために使用する新しい変数 ChangedDate
を定義します。 パラメータとして today
を受け取り、DatePipe()
を使用して日付を定義した新しい形式に変換する関数 changeFormat()
を作成します。
日付のタイムゾーンを格納する変数 pipe
を定義します。
app.component.ts
のコードは次のようになります。
# Angular
import { Component, VERSION } from '@angular/core';
import { DatePipe } from '@angular/common';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
name = 'Angular ';
today = new Date();
changedDate = '';
pipe = new DatePipe('en-US');
changeFormat(today){
let ChangedFormat = this.pipe.transform(this.today, 'dd/MM/YYYY');
this.changedDate = ChangedFormat;
console.log(this.changedDate);
}
}
このボタンをクリックすると changeFormat()
関数をトリガーする新しいボタンを作成します。 また、割り当てたばかりの新しい変数を使用して、日付を新しい形式で表示します。
# Angular
<hello name="{{ name }}"></hello>
<p>
Today is {{ today }}
</p>
<button (click)='changeFormat(today)'>Change Date</button>
<p>
Today is {{ this.changedDate }}
</p>
出力:
上記の例では、Tue Apr 05 2022 17:16:06 GMT+0500 (Pakistan Standard Time)
と表示されますが、"Change Date"
ボタンをクリックすると、内部で changeFormat()
関数が呼び出されます。 click()
メソッドを呼び出して、書式を変更した日付を返します。
このようにして、DatePipe()
を使用して形式を変更できるアプリケーションで現在の日付を取得できます。
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn