Selezionare le colonne del DataFrame Pandas
Suraj Joshi
30 gennaio 2023
Pandas
Pandas DataFrame Column
- Seleziona colonne da un DataFrame Pandas utilizzando l’operazione di indicizzazione
-
Selezionare le colonne da un DataFrame Pandas utilizzando il metodo
DataFrame.drop()
-
Seleziona colonne da un DataFrame Pandas usando il metodo
DataFrame.filter()
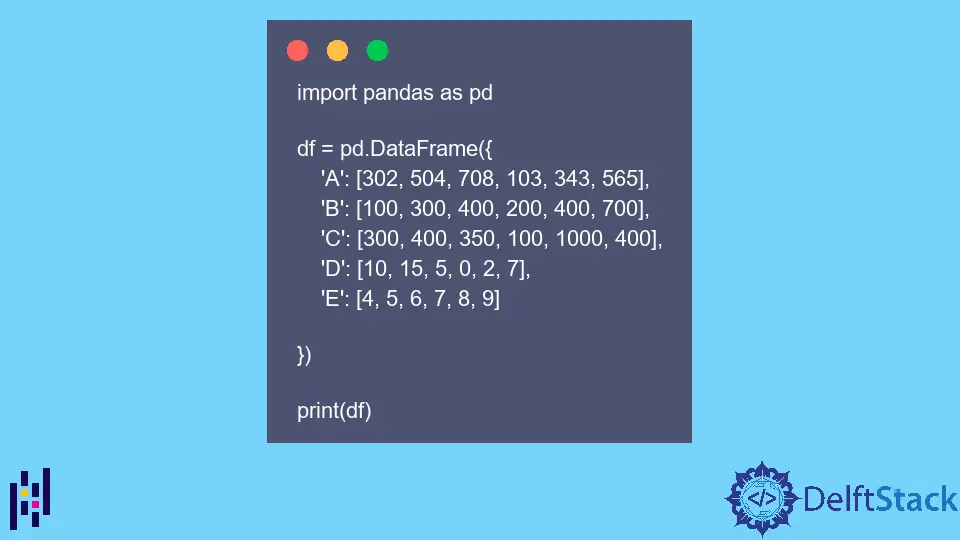
Questo tutorial spiega come possiamo selezionare le colonne da un Pandas DataFrame indicizzando o usando i metodi DataFrame.drop()
e DataFrame.filter()
.
Useremo il DataFrame df
come sotto per spiegare come possiamo selezionare le colonne da un Pandas DataFrame.
import pandas as pd
df = pd.DataFrame(
{
"A": [302, 504, 708, 103, 343, 565],
"B": [100, 300, 400, 200, 400, 700],
"C": [300, 400, 350, 100, 1000, 400],
"D": [10, 15, 5, 0, 2, 7],
"E": [4, 5, 6, 7, 8, 9],
}
)
print(df)
Produzione:
A B C D E
0 302 100 300 10 4
1 504 300 400 15 5
2 708 400 350 5 6
3 103 200 100 0 7
4 343 400 1000 2 8
5 565 700 400 7 9
Seleziona colonne da un DataFrame Pandas utilizzando l’operazione di indicizzazione
import pandas as pd
df = pd.DataFrame(
{
"A": [302, 504, 708, 103, 343, 565],
"B": [100, 300, 400, 200, 400, 700],
"C": [300, 400, 350, 100, 1000, 400],
"D": [10, 15, 5, 0, 2, 7],
"E": [4, 5, 6, 7, 8, 9],
}
)
derived_df = df[["A", "C", "E"]]
print("The initial DataFrame is:")
print(df, "\n")
print("The DataFrame with A,C and E columns is:")
print(derived_df, "\n")
Produzione:
The initial DataFrame is:
A B C D E
0 302 100 300 10 4
1 504 300 400 15 5
2 708 400 350 5 6
3 103 200 100 0 7
4 343 400 1000 2 8
5 565 700 400 7 9
The DataFrame with A,C and E columns is:
A C E
0 302 300 4
1 504 400 5
2 708 350 6
3 103 100 7
4 343 1000 8
5 565 400 9
Seleziona le colonne A
, C
e E
dal DataFrame df
e assegna queste colonne al DataFrame derivato_df
.
Selezionare le colonne da un DataFrame Pandas utilizzando il metodo DataFrame.drop()
import pandas as pd
df = pd.DataFrame(
{
"A": [302, 504, 708, 103, 343, 565],
"B": [100, 300, 400, 200, 400, 700],
"C": [300, 400, 350, 100, 1000, 400],
"D": [10, 15, 5, 0, 2, 7],
"E": [4, 5, 6, 7, 8, 9],
}
)
derived_df = df.drop(["B", "D"], axis=1)
print("The initial DataFrame is:")
print(df, "\n")
print("The DataFrame with A,C and E columns is:")
print(derived_df, "\n")
Produzione:
The initial DataFrame is:
A B C D E
0 302 100 300 10 4
1 504 300 400 15 5
2 708 400 350 5 6
3 103 200 100 0 7
4 343 400 1000 2 8
5 565 700 400 7 9
The DataFrame with A,C and E columns is:
A C E
0 302 300 4
1 504 400 5
2 708 350 6
3 103 100 7
4 343 1000 8
5 565 400 9
Elimina le colonne B
e D
dal DataFrame df
e assegna le colonne rimanenti a derived_df
. In alternativa, seleziona tutte le colonne tranne B
e D
e le assegna al DataFrame derived_df
.
Seleziona colonne da un DataFrame Pandas usando il metodo DataFrame.filter()
import pandas as pd
df = pd.DataFrame(
{
"A": [302, 504, 708, 103, 343, 565],
"B": [100, 300, 400, 200, 400, 700],
"C": [300, 400, 350, 100, 1000, 400],
"D": [10, 15, 5, 0, 2, 7],
"E": [4, 5, 6, 7, 8, 9],
}
)
derived_df = df.filter(["A", "C", "E"])
print("The initial DataFrame is:")
print(df, "\n")
print("The DataFrame with A,C and E columns is:")
print(derived_df, "\n")
Produzione:
The initial DataFrame is:
A B C D E
0 302 100 300 10 4
1 504 300 400 15 5
2 708 400 350 5 6
3 103 200 100 0 7
4 343 400 1000 2 8
5 565 700 400 7 9
The DataFrame with A,C and E columns is:
A C E
0 302 300 4
1 504 400 5
2 708 350 6
3 103 100 7
4 343 1000 8
5 565 400 9
Estrae o filtra le colonne A
, C
e E
dal DataFrame df
e lo assegna al DataFrame derived_df
.
Ti piacciono i nostri tutorial? Iscriviti a DelftStack su YouTube per aiutarci a creare altre guide video di alta qualità. Iscriviti
Autore: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInArticolo correlato - Pandas DataFrame Column
- Come ottenere le intestazioni delle colonne DataFrame Pandas come lista
- Come cancellare la colonna DataFrame Pandas DataFrame
- Come convertire la colonna DataFrame in data e ora in pandas
- Ottieni la colonna della somma dei pandas
- Modificare l'ordine delle colonne DataFrame di Pandas
- Converti colonna DataFrame in stringa in Pandas